Excel VBA: Automating Desktop Shortcut Creation for Excel Files
Did you know that manual navigation to network folders can consume up to 30% of an average worker’s productive time? That’s a significant amount of valuable time that could be better spent on important tasks. Fortunately, with the power of Excel VBA, you can automate the process of creating desktop shortcuts for your Excel files, streamlining your workflow and saving you precious time.
Creating Excel File Shortcuts with VBA Macros
You can create Excel file shortcuts using VBA macros by utilizing the CreateShortcut
method from the WshShell
object in VBA. Here’s a simple example to create a desktop shortcut for an Excel file:
Sub CreateShortcut()
Dim WshShell As Object
Dim MyShortcut As Object
Dim DesktopPath As String
Dim ExcelFilePath As String ' Set the path to the Excel file
ExcelFilePath = "C:\Path\To\Your\Excel\File.xlsx"
' Get the desktop path
DesktopPath = CreateObject("WScript.Shell").SpecialFolders("Desktop")
' Create a WshShell object
Set WshShell = CreateObject("WScript.Shell")
' Create a shortcut object
Set MyShortcut = WshShell.CreateShortcut(DesktopPath & "\ExcelShortcut.lnk")
' Set properties of the shortcut
MyShortcut.TargetPath = ExcelFilePath
MyShortcut.Save
' Clean up
Set MyShortcut = Nothing
Set WshShell = Nothing
MsgBox "Shortcut created successfully on desktop!"
End Sub
Make sure to replace "C:\Path\To\Your\Excel\File.xlsx"
with the actual path to your Excel file. When you run this macro, it will create a shortcut named “ExcelShortcut.lnk” on your desktop that points to your specified Excel file.
You can further modify this code to customize the shortcut properties such as icon, working directory, etc., by adjusting the properties of the MyShortcut
object accordingly.
Dealing with the Macro Security Warning Prompt
When you encounter the macro security warning prompt when opening a file through a VBA-created shortcut, it typically means that the security settings in Microsoft Excel are set to prompt the user whenever a file containing macros is opened. There are a few approaches to handle this situation:
- Change Macro Security Settings: One way to handle this is to change the macro security settings in Excel to either lower the security level or to trust the location where the file is stored. However, this approach may not be ideal if you are concerned about security risks associated with running macros from unknown sources.
- Digitally Sign Macros: If the macros in the Excel file are digitally signed with a trusted certificate, Excel may not prompt the user to enable macros each time the file is opened. This requires obtaining a digital certificate from a trusted Certificate Authority (CA) and signing the macros using tools provided by Excel.
- Consider Trusted Locations: You can designate specific folders as trusted locations in Excel where files with macros can be stored. Excel typically doesn’t prompt users to enable macros when opening files from trusted locations. This approach is more secure than lowering the macro security settings for all files.
- Use Group Policy: If you’re in an organization and managing multiple computers, you can use Group Policy to configure macro security settings centrally. This allows you to enforce security policies consistently across all computers.
- VBA Code Signing: If the macros are running from a location not considered trusted by Excel, signing the VBA project with a trusted digital certificate can help mitigate the security warnings. This involves using tools like Microsoft’s SignTool to sign the VBA code.
Customizing Shortcut Creation with Cell References
While the previously mentioned VBA code allows for the creation of desktop shortcuts, it can be further enhanced by integrating cell references. By utilizing cell references, users can generate shortcuts that dynamically update based on the values stored in specific cells. This level of customization provides a flexible and efficient solution for managing shortcuts to multiple Excel files.
How to Customize Shortcut Creation with Cell References
To customize shortcut creation with cell references, modifications can be made to the VBA code described earlier. The following steps outline the process:
- Identify the cells containing the file paths that will serve as the basis for the shortcuts.
- In the VBA code, replace the hardcoded file path with the cell reference using the appropriate syntax.
- Implement error handling mechanisms to account for any potential errors that may occur due to changes in the referenced cells.
By incorporating cell references into the “TargetPath” property of the shortcut, users can ensure that the shortcuts automatically update based on changes in the referenced cells. This eliminates the need for manual updates and provides a streamlined solution for managing shortcuts to Excel files.
An Example of Customized Shortcut Creation with Cell References
Consider the following example scenario:
Cell A1 | Cell B1 |
---|---|
File 1 | C:\Documents\File1.xlsx |
File 2 | C:\Documents\File2.xlsx |
File 3 | C:\Documents\File3.xlsx |
Using cell references, the VBA code can be modified to create shortcuts that link to the corresponding Excel files:
Sub CreateShortcuts()
Dim Shell As Object
Dim Shortcut As Object
Set Shell = CreateObject("Wscript.Shell")
' Shortcut 1
Set Shortcut = Shell.CreateShortcut("C:\Users\Username\Desktop\File1.lnk")
Shortcut.TargetPath = Range("B2").Value
Shortcut.Save
' Shortcut 2
Set Shortcut = Shell.CreateShortcut("C:\Users\Username\Desktop\File2.lnk")
Shortcut.TargetPath = Range("B3").Value
Shortcut.Save
' Shortcut 3
Set Shortcut = Shell.CreateShortcut("C:\Users\Username\Desktop\File3.lnk")
Shortcut.TargetPath = Range("B4").Value
Shortcut.Save
Set Shortcut = Nothing
Set Shell = Nothing
End Sub
In this example, the VBA code generates three shortcuts on the desktop, each linked to the respective Excel file specified in columns B. By modifying the cell references, users can easily add or remove shortcuts and update the file paths without modifying the VBA code.
Utilizing CleanName Function for Path Cleaning
When creating desktop shortcuts for Excel files using VBA, it’s essential to ensure the proper functioning of the VBA code. One crucial step in this process is to utilize the CleanName function to clean the paths and remove any invalid characters.
The CleanName function is specifically designed to eliminate characters that are not allowed in file or folder names, such as slashes and asterisks. By incorporating this function into the VBA code, you can ensure that the generated shortcuts have valid and clean paths.
This is particularly important as having invalid characters in the path can lead to errors or issues when creating and accessing the shortcuts on the desktop. By cleaning the path before creating the shortcut, you can prevent any potential disruptions to your automated workflow.
The CleanName function can be easily integrated into your VBA code. Simply call the function and pass the path as a parameter. The function will then clean the path by removing any prohibited characters, ensuring the path is valid and safe to use.
Here’s an example of how the CleanName function can be used in VBA code:
Function CleanName(ByVal inputName As String) As String
' Remove invalid characters from the inputName
' CleanedName = ...
' ...
' Return the cleaned name
CleanName = CleanedName
End Function
By incorporating the CleanName function into your VBA code, you can guarantee that the paths used for creating desktop shortcuts are sanitized and free from any invalid characters.
CleanName Function | Description |
---|---|
Function Name | CleanName |
Parameters | inputName: The original path string that needs to be cleaned |
Return Value | A clean and sanitized version of the inputName path |
Example | cleanedPath = CleanName("C:\Folder\My*File.xlsx") |
With the CleanName function in place, you can confidently create desktop shortcuts for your Excel files using VBA, knowing that the paths are clean and valid.
Further Enhancements and Error Handling
While the basic functionality of creating shortcuts using VBA code is already impressive, users can take it a step further by enhancing the code to meet their specific needs. One important aspect to consider is error handling. By incorporating error handling mechanisms, users can gracefully manage any potential errors that may occur during the shortcut creation process.
Error handling allows the VBA code to anticipate and handle various error scenarios, ensuring a smooth and reliable execution. Whether it’s a file not found error or an invalid path, error handling can provide feedback to the user and prevent any disruptions in the automation workflow.
Aside from error handling, users can also explore additional parameters and customization options to make the code even more versatile. This could involve incorporating user input for shortcut names, enabling users to specify the target folder dynamically, or adding features like shortcut icons.
By continuously improving and refining the VBA code, users can create a comprehensive solution for automating desktop shortcut creation for Excel files. With error handling in place and customized parameters, the code becomes more robust and reliable, ensuring a seamless automation experience for users.
FAQ
What is Excel VBA?
How can I create a desktop shortcut for an Excel file using VBA?
Why am I encountering a macro security warning prompt when opening an Excel file through a VBA-created shortcut?
How can I customize the shortcut creation process with cell references?
What is the CleanName function and how can it be used in shortcut creation?
Can I further enhance the VBA code for shortcut creation?
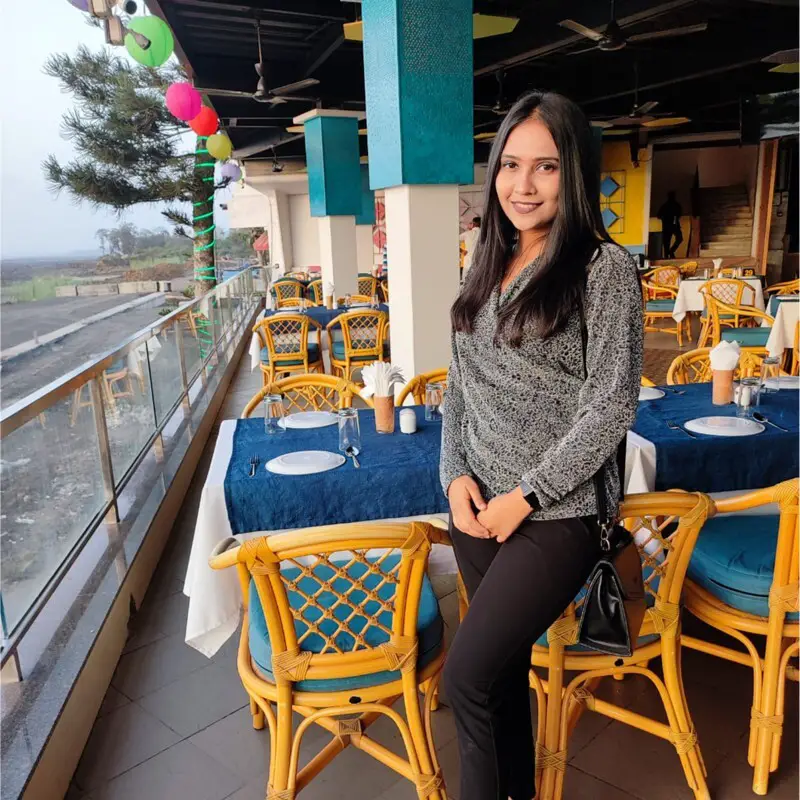
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.