How to Select Visible Cells After Using AutoFilter in Excel VBA?
If you frequently work with large datasets in Microsoft Excel, you may find yourself using the AutoFilter feature to quickly filter your data based on specific criteria. However, after applying the filter, you might need to select only the visible cells for further processing or analysis. In this article, we will explore how to use Excel VBA to select visible cells after using AutoFilter.
Understanding AutoFilter and Visible Cells
Before we dive into the VBA code, let’s first understand what AutoFilter is and what we mean by visible cells.
What is AutoFilter?
AutoFilter is a built-in feature in Excel that allows you to filter your data based on specific criteria. It enables you to quickly display only the rows that meet your desired conditions, making it easier to analyze and work with large datasets.
AutoFilter works by adding drop-down arrows to the header row of your data range. When you click on these arrows, you can select specific values, apply custom filters, or even create complex criteria using wildcards and logical operators.
Some common scenarios where AutoFilter can be useful include:
- Filtering by category: If you have a column with categories like “Product Type” or “Region,” you can use AutoFilter to display only the rows that belong to a specific category.
- Filtering by value range: If you have numerical data, such as sales figures or ages, you can use AutoFilter to display only the rows that fall within a specific value range.
- Filtering by text: If you have text data, such as names or addresses, you can use AutoFilter to display only the rows that contain specific keywords or patterns.
What are Visible Cells?
After applying an AutoFilter, some rows may be hidden based on the filter criteria. The cells that remain visible are referred to as “visible cells.” These are the cells that are not hidden by the filter and are still displayed in the worksheet.
When you apply an AutoFilter, Excel automatically hides the rows that do not meet the specified criteria. This allows you to focus on the relevant data without being distracted by unnecessary information.
It’s important to note that the hidden rows are not deleted; they are simply hidden from view. You can easily remove the AutoFilter to display all the rows again.
Selecting Visible Cells Using Excel VBA
Now that we have a clear understanding of AutoFilter and visible cells, let’s explore how to use Excel VBA to select the visible cells after applying a filter.
Step 1: Applying AutoFilter
Before we can select the visible cells, we need to apply the AutoFilter to our data. Here’s an example of how to apply AutoFilter using VBA:
Sub ApplyAutoFilter()
'Assuming data is in Column A
Range("A1").AutoFilter Field:=1, Criteria1:="Your Criteria"
End Sub
In this example, we assume that the data is in Column A, and we apply the AutoFilter based on a specific criteria. Replace "Your Criteria"
with the actual criteria you want to use for filtering.
You can also apply AutoFilter to multiple columns by specifying different Field
numbers and criteria. For example:
Sub ApplyAutoFilter()
'Assuming data is in Columns A, B, and C
Range("A1").AutoFilter Field:=1, Criteria1:="Criteria for Column A"
Range("A1").AutoFilter Field:=2, Criteria1:="Criteria for Column B"
Range("A1").AutoFilter Field:=3, Criteria1:="Criteria for Column C"
End Sub
Step 2: Selecting Visible Cells
After applying the AutoFilter, we can now select the visible cells using VBA. Here’s the code to accomplish this:
Sub SelectVisibleCells()
'Assuming data is in Column A
Range("A1").AutoFilter Field:=1, Criteria1:="Your Criteria"
On Error Resume Next
'Select visible cells in Column A
Range("A2", Range("A" & Rows.Count).End(xlUp)).SpecialCells(xlCellTypeVisible).Select
On Error GoTo 0
End Sub
Let’s break down the code:
- We start by applying the AutoFilter, as explained in Step 1.
- We use
On Error Resume Next
to handle any potential errors that may occur if there are no visible cells after filtering. - We use the
Range
object to select the visible cells in Column A. We start from cell A2 (assuming the header is in A1) and go down to the last non-empty cell in the column usingRange("A" & Rows.Count).End(xlUp)
. - We use the
SpecialCells
method with thexlCellTypeVisible
parameter to select only the visible cells. - Finally, we use
On Error GoTo 0
to reset the error handling.
The SpecialCells
method is a powerful tool in VBA that allows you to select cells based on specific criteria. In this case, we use xlCellTypeVisible
to select only the cells that are visible after applying the AutoFilter.
You can also select visible cells across multiple columns by modifying the range reference. For example:
Sub SelectVisibleCells()
'Assuming data is in Columns A, B, and C
Range("A1").AutoFilter Field:=1, Criteria1:="Criteria for Column A"
Range("A1").AutoFilter Field:=2, Criteria1:="Criteria for Column B"
Range("A1").AutoFilter Field:=3, Criteria1:="Criteria for Column C"
On Error Resume Next
'Select visible cells in Columns A, B, and C
Range("A2:C" & Rows.Count).SpecialCells(xlCellTypeVisible).Select
On Error GoTo 0
End Sub
Step 3: Working with Selected Visible Cells
Once you have selected the visible cells, you can perform various operations on them, such as:
- Copying and pasting the data to another location
- Applying formatting to the selected cells
- Extracting values for further analysis
- Performing calculations on the selected data
Here’s an example of how you can copy the selected visible cells to another worksheet:
Sub CopyVisibleCells()
'Assuming data is in Column A
Range("A1").AutoFilter Field:=1, Criteria1:="Your Criteria"
On Error Resume Next
'Select visible cells in Column A
Range("A2", Range("A" & Rows.Count).End(xlUp)).SpecialCells(xlCellTypeVisible).Select
On Error GoTo 0
'Copy selected cells
Selection.Copy
'Paste to another worksheet
Worksheets("Destination").Range("A1").PasteSpecial xlPasteValues
End Sub
In this example, after selecting the visible cells, we use the Copy
method to copy the selected cells and then paste them to another worksheet using the PasteSpecial
method with the xlPasteValues
parameter.
You can also perform other operations on the selected visible cells, such as:
- Formatting: You can apply formatting to the selected cells using properties like
Font
,Interior
,Borders
, etc. For example:
Selection.Font.Bold = True
Selection.Interior.Color = RGB(255, 255, 0)
- Extracting Values: You can extract the values of the selected cells into an array for further analysis. For example:
Dim visibleData As Variant
visibleData = Selection.Value
- Performing Calculations: You can perform calculations on the selected data using VBA functions or by accessing the cell values directly. For example:
Dim totalSum As Double
totalSum = Application.WorksheetFunction.Sum(Selection)
Tips and Considerations
When working with AutoFilter and selecting visible cells using VBA, keep the following tips and considerations in mind:
- Header Row: Make sure to exclude the header row when selecting the visible cells. In our examples, we assumed the header is in row 1 and started selecting from row 2.
- Error Handling: Use error handling techniques, such as
On Error Resume Next
, to handle cases where there might be no visible cells after filtering. - Range References: Adjust the range references based on your specific data layout. In our examples, we assumed the data is in Column A, but you can modify the range references to match your data’s location.
- Performance: When working with large datasets, selecting visible cells using VBA can be faster than manually selecting them. However, be mindful of the performance impact when dealing with extremely large datasets.
- Clearing AutoFilter: After you have finished working with the filtered data, it’s a good practice to clear the AutoFilter to restore the original data view. You can do this using the following code:
ActiveSheet.AutoFilterMode = False
- Multiple Criteria: When applying AutoFilter with multiple criteria, you can use the
Operator
parameter to specify how the criteria should be combined. For example, you can usexlAnd
to display rows that meet all the criteria orxlOr
to display rows that meet any of the criteria. - Dynamic Range: If your data range is dynamic and may change over time, consider using a dynamic range reference to ensure that the AutoFilter is applied to the entire data set. You can use techniques like
UsedRange
orCurrentRegion
to determine the data range dynamically.
Final Thoughts
Selecting visible cells after applying an AutoFilter in Excel can be a tedious task when done manually. However, by leveraging the power of Excel VBA, you can automate this process and save time and effort. By following the steps outlined in this article, you can easily select visible cells and perform further operations on them.
Remember to adjust the code based on your specific data layout and requirements. With a little bit of VBA knowledge, you can streamline your workflow and efficiently work with filtered data in Excel.
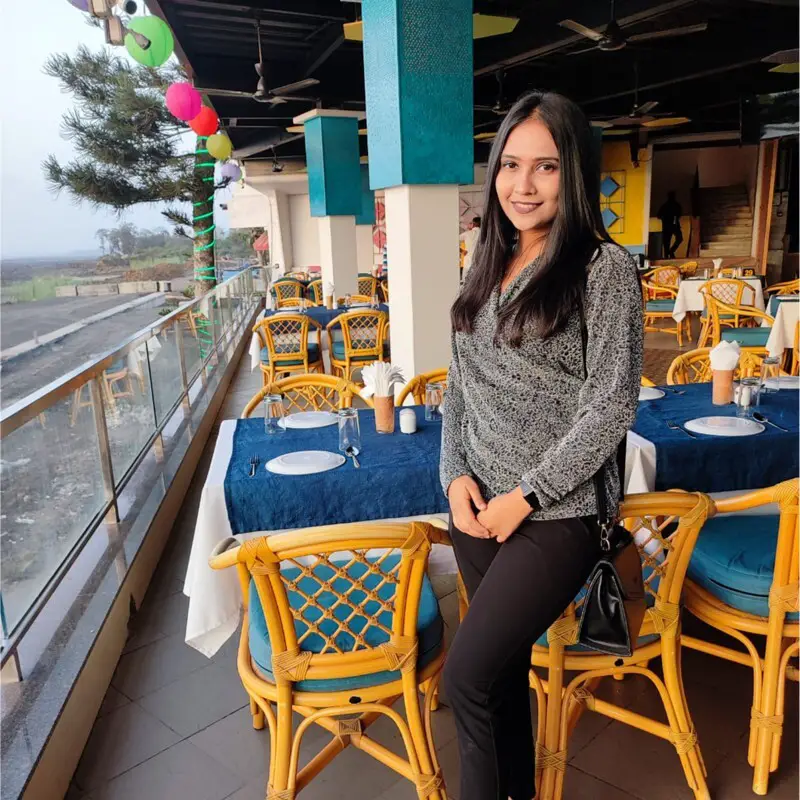
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.