Understanding and Fixing Runtime Error 1004 in Excel VBA
If you’re working with Excel VBA and encountering Error 1004, this comprehensive guide will help you understand what causes this error and provide step-by-step solutions to fix it. Error 1004 is a common issue that can occur in various situations when using Excel VBA, such as when trying to open, save, or access workbooks and worksheets.
What is Excel VBA Error 1004?
Excel VBA Error 1004 is a run-time error that occurs when a VBA macro encounters a problem while executing a specific command or operation. The error message typically reads:
“Application-defined or object-defined error”
This error can be triggered by several factors, including:
- Incorrect file paths or file names
- Attempting to access a non-existent or protected workbook or worksheet
- Insufficient permissions to perform the requested action
- Conflicts with other open workbooks or applications
Understanding the root cause of the error is crucial for finding the appropriate solution.
Common Scenarios That Trigger Error 1004
Scenario 1: Incorrect File Path or File Name
One of the most common reasons for Error 1004 is specifying an incorrect file path or file name when attempting to open, save, or access a workbook. This can happen if:
- The file path is misspelled or contains invalid characters
- The file has been moved or renamed without updating the VBA code
- The file extension is missing or incorrect
To fix this issue:
- Double-check the file path and file name in your VBA code
- Ensure that the file exists in the specified location
- Verify that the file extension is correct (.xlsx, .xlsm, etc.)
- Use the
Dir
function to check if the file exists before attempting to access it
Example:
If Dir("C:\MyFolder\MyWorkbook.xlsx") <> "" Then
Workbooks.Open "C:\MyFolder\MyWorkbook.xlsx"
Else
MsgBox "The specified file does not exist."
End If
Additional Tips for Handling File Paths and Names
- Use constants or variables to store file paths and names, making it easier to update them if needed
- Implement file dialog boxes to allow users to select files dynamically
- Use the
FileSystemObject
to work with files and folders more efficiently
Example:
Const FILE_PATH As String = "C:\MyFolder\MyWorkbook.xlsx"
If FileExists(FILE_PATH) Then
Workbooks.Open FILE_PATH
Else
MsgBox "The specified file does not exist."
End If
Function FileExists(ByVal FilePath As String) As Boolean
Dim FSO As Object
Set FSO = CreateObject("Scripting.FileSystemObject")
FileExists = FSO.FileExists(FilePath)
Set FSO = Nothing
End Function
Scenario 2: Accessing a Non-Existent or Protected Workbook or Worksheet
Another common cause of Error 1004 is attempting to access a workbook or worksheet that doesn’t exist or is protected. This can occur if:
- The workbook or worksheet has been deleted or renamed
- The workbook or worksheet is protected and requires a password to access
- The specified sheet name or index is incorrect
To resolve this issue:
- Verify that the workbook and worksheet exist and are accessible
- If the workbook or worksheet is protected, provide the correct password using the
Workbooks.Open
orWorksheets.Unprotect
method - Double-check the sheet name or index in your VBA code
Example:
On Error Resume Next
Workbooks("MyWorkbook.xlsx").Worksheets("Sheet1").Activate
If Err.Number = 1004 Then
MsgBox "The specified worksheet does not exist."
End If
On Error GoTo 0
Handling Protected Workbooks and Worksheets
If you encounter Error 1004 due to a protected workbook or worksheet, you can try the following:
- Use the
Workbooks.Open
method with thePassword
argument to open a protected workbook - Use the
Worksheets.Unprotect
method with the correct password to unprotect a worksheet
Example:
Const PASSWORD As String = "MyPassword"
Workbooks.Open "C:\MyFolder\ProtectedWorkbook.xlsx", Password:=PASSWORD
Workbooks("ProtectedWorkbook.xlsx").Worksheets("Sheet1").Unprotect Password:=PASSWORD
Scenario 3: Insufficient Permissions
Error 1004 can also occur when the user running the VBA macro lacks sufficient permissions to perform the requested action. This may happen when:
- The user doesn’t have read/write access to the specified file or folder
- The workbook or worksheet is marked as read-only
- The VBA macro is attempting to modify a protected workbook or worksheet
To overcome this issue:
- Ensure that the user has the necessary permissions to access and modify the files
- Check if the workbook or worksheet is marked as read-only and remove the read-only attribute if needed
- Unprotect the workbook or worksheet before attempting to modify it
Example:
If ActiveWorkbook.ReadOnly Then
MsgBox "The workbook is read-only. Please save a copy and try again."
Else
' Perform desired actions
End If
Granting Necessary Permissions
To grant the necessary permissions for a user to access and modify files:
- Right-click the file or folder and select “Properties”
- Go to the “Security” tab and click “Edit”
- Add the user or group and grant the appropriate permissions (e.g., Read, Write, Modify)
- Click “Apply” and “OK” to save the changes
Alternatively, you can use the FileSecurity
object in VBA to programmatically manage file permissions.
Scenario 4: Conflicts with Other Open Workbooks or Applications
Error 1004 can sometimes be caused by conflicts with other open workbooks or applications. This may occur when:
- Multiple workbooks with the same name are open simultaneously
- Another application is using the desired workbook or worksheet
- The VBA macro is attempting to access a workbook or worksheet that is already open in exclusive mode
To address this issue:
- Close any unnecessary workbooks or applications that may be interfering with the VBA macro
- Use the
Workbooks.Open
method with theReadOnly:=True
argument to open the workbook in read-only mode if it’s being used by another application - Implement error handling to gracefully handle conflicts and provide informative error messages
Example:
On Error Resume Next
Workbooks.Open "C:\MyFolder\MyWorkbook.xlsx", ReadOnly:=True
If Err.Number = 1004 Then
MsgBox "The workbook is currently in use by another application. Please try again later."
End If
On Error GoTo 0
Avoiding Conflicts with Other Applications
To minimize conflicts with other applications:
- Close unnecessary applications before running the VBA macro
- Use the
Application.DisplayAlerts
property to suppress prompts and alerts that may interrupt the macro execution - Implement a retry mechanism with a delay to allow time for other applications to release the workbook
Example:
Const MAX_RETRIES As Integer = 3
Const RETRY_DELAY As Integer = 5 ' Seconds
Dim Retries As Integer
Retries = 0
Do
On Error Resume Next
Workbooks.Open "C:\MyFolder\MyWorkbook.xlsx"
If Err.Number = 1004 Then
Retries = Retries + 1
If Retries <= MAX_RETRIES Then
Application.Wait (Now + TimeValue("0:00:" & RETRY_DELAY))
Else
MsgBox "The workbook is currently in use by another application. Please try again later."
Exit Sub
End If
Else
Exit Do
End If
On Error GoTo 0
Loop
Debugging Techniques for Error 1004
When encountering Error 1004, it’s essential to use proper debugging techniques to identify the source of the problem and find a suitable solution. Here are some helpful debugging tips:
- Use the
On Error GoTo
statement to redirect the code execution to an error-handling routine when an error occurs - Implement the
Err.Number
,Err.Source
, andErr.Description
properties to gather information about the error - Use breakpoints and the VBA debugger to step through the code and identify the exact line causing the error
- Print variable values using the
Debug.Print
statement or message boxes to ensure they contain the expected values - Consult Excel VBA documentation and online forums for additional guidance and solutions
Example:
Sub DebugError1004()
On Error GoTo ErrorHandler
' Code that may trigger Error 1004
Workbooks.Open "C:\MyFolder\NonExistentWorkbook.xlsx"
Exit Sub
ErrorHandler:
If Err.Number = 1004 Then
Debug.Print "Error 1004 occurred in " & Err.Source
Debug.Print "Error Description: " & Err.Description
' Handle the error appropriately
Else
' Handle other errors
End If
End Sub
Additional Debugging Tips
- Use the
Stop
statement to pause code execution and enter break mode - Utilize the “Immediate” window to execute VBA statements and check variable values during debugging
- Implement logging mechanisms to record important information and error details for later analysis
- Break complex procedures into smaller, more manageable units for easier debugging and maintenance
Best Practices to Prevent Error 1004
To minimize the occurrence of Error 1004 in your Excel VBA projects, follow these best practices:
- Use correct and up-to-date file paths and file names
- Implement error handling to gracefully handle potential errors
- Verify the existence and accessibility of workbooks and worksheets before attempting to access them
- Ensure that the user has the necessary permissions to perform the desired actions
- Close unnecessary workbooks and applications to avoid conflicts
- Use meaningful variable and procedure names to enhance code readability and maintainability
- Regularly test and debug your VBA code to identify and fix potential issues
By adhering to these best practices, you can significantly reduce the chances of encountering Error 1004 and create more robust and reliable Excel VBA solutions.
Additional Best Practices
- Use version control systems to track changes and collaborate with others
- Implement automated testing to ensure code quality and catch potential issues early
- Follow coding standards and guidelines to maintain consistency and readability
- Regularly refactor and optimize your code to improve performance and maintainability
- Stay updated with the latest Excel VBA features, best practices, and security patches
Summary
Excel VBA Error 1004 is a common run-time error that can occur due to various reasons, such as incorrect file paths, attempting to access non-existent or protected workbooks and worksheets, insufficient permissions, or conflicts with other open workbooks or applications.
To fix Error 1004, you should:
- Verify file paths and file names
- Ensure workbooks and worksheets exist and are accessible
- Provide necessary permissions and passwords
- Close unnecessary workbooks and applications
- Implement error handling and debugging techniques
FAQs
What is runtime error 1004 in Excel VBA?
What are the common causes of runtime error 1004?
How can I fix runtime error 1004 caused by an incorrect file path?
Dir
function to check if the file exists before attempting to access it.What should I do if runtime error 1004 occurs due to insufficient permissions?
How can I prevent runtime error 1004 in my Excel VBA projects?
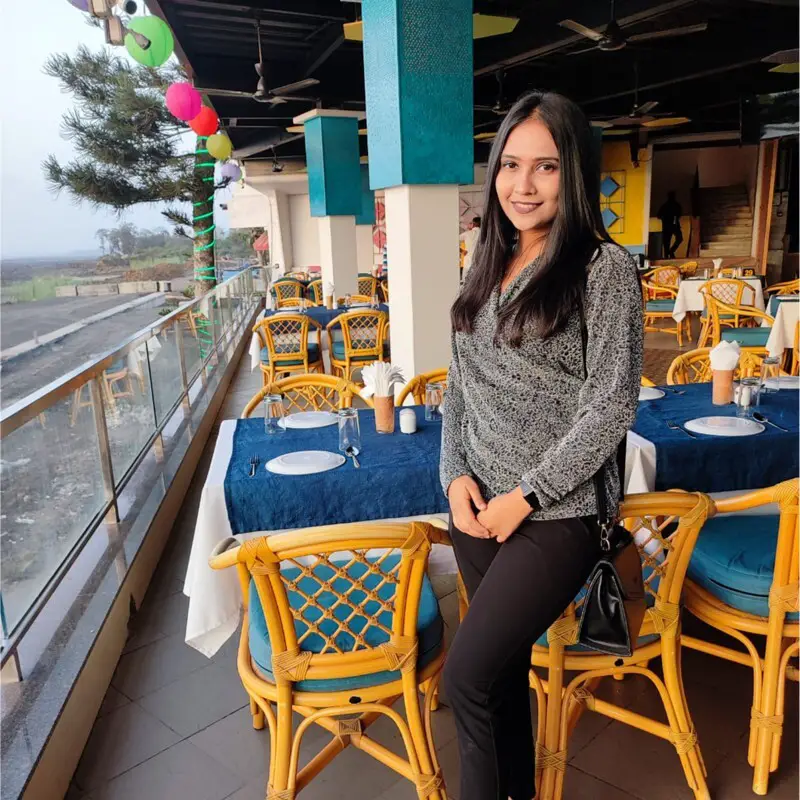
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.