How to Upload a File to SharePoint Using Excel VBA?
Automating file uploads to SharePoint using Excel VBA can save time, reduce manual steps, and ensure consistency when managing documents. Whether you’re handling reports, data sheets, or backup files, you can streamline the upload process directly from your Excel workbook.
In this step-by-step guide, we will explain how to upload a file to SharePoint using Excel VBA, including working examples, best practices, and troubleshooting tips.
Understanding How Excel VBA Interacts with SharePoint
When you upload a file to SharePoint from Excel, the process involves:
- Creating a connection to the SharePoint site
- Specifying the SharePoint document library
- Selecting the file to upload from your local drive
- Sending the file using HTTP requests (such as
WinHttpRequest
orXMLHTTP
) - Handling authentication and upload confirmation
Excel doesn’t offer a built-in SharePoint integration, so VBA uses Microsoft XML (MSXML) or WinHTTP Services to perform HTTP actions like file upload.
Prerequisites for Uploading a File to SharePoint Using Excel VBA
Before starting, ensure the following:
- You have access to the SharePoint Online site or document library
- You know the library’s full path
- Your SharePoint permissions allow file uploads
- The VBA References for XML and HTTP requests are enabled
Required VBA References
To upload files with VBA, enable the following libraries in the VBA Editor:
Reference Name | Library DLL File |
---|---|
Microsoft XML, v6.0 | msxml6.dll (usually in C:\Windows\System32 ) |
Microsoft WinHTTP Services, version 5.1 | winhttp.dll |
To enable:
- Open Excel and press Alt + F11
- Go to Tools > References
- Check the boxes next to:
- Microsoft XML, v6.0
- Microsoft WinHTTP Services, version 5.1
- Click OK
Step-by-Step: Upload File to SharePoint Using Excel VBA
Step 1: Prepare the File Details
Define the path of the file you want to upload and the file name:
Dim filePath As String
Dim fileName As String
fileName = "Report.xlsx"
filePath = "C:\Reports\" & fileName
Step 2: Set the SharePoint URL
Set the target SharePoint document library URL where you want to upload the file:
Dim sharePointURL As String
sharePointURL = "https://yourcompany.sharepoint.com/sites/YourSite/Shared Documents/" & fileName
Note: The URL must be properly encoded. Replace spaces with
%20
or use a function to encode URLs dynamically.
Step 3: Use WinHttpRequest to Upload the File
Use the following VBA code to send the file to SharePoint using the WinHttpRequest
object:
Sub UploadFileToSharePoint()
Dim filePath As String
Dim fileName As String
Dim sharePointURL As String
Dim binaryStream As Object
Dim httpRequest As Object
fileName = "Report.xlsx"
filePath = "C:\Reports\" & fileName
sharePointURL = "https://yourcompany.sharepoint.com/sites/YourSite/Shared%20Documents/" & fileName
' Read file as binary
Set binaryStream = CreateObject("ADODB.Stream")
With binaryStream
.Type = 1 ' Binary
.Open
.LoadFromFile filePath
End With
' Initialize WinHttpRequest
Set httpRequest = CreateObject("WinHttp.WinHttpRequest.5.1")
httpRequest.Open "PUT", sharePointURL, False
' Set necessary headers
httpRequest.setRequestHeader "Content-Type", "application/octet-stream"
' Send binary file data
httpRequest.Send binaryStream.Read
' Check result
If httpRequest.Status = 200 Or httpRequest.Status = 201 Then
MsgBox "File uploaded successfully!"
Else
MsgBox "Upload failed. Status: " & httpRequest.Status & " - " & httpRequest.StatusText
End If
' Clean up
binaryStream.Close
Set binaryStream = Nothing
Set httpRequest = Nothing
End Sub
What This Code Does:
- Reads the Excel file in binary mode using
ADODB.Stream
- Sends a PUT request to SharePoint using
WinHttpRequest
- Uploads the file to the specified SharePoint URL
- Displays a message based on the HTTP response status
Uploading to a Subfolder in SharePoint
To upload to a specific folder inside the document library:
sharePointURL = "https://yourcompany.sharepoint.com/sites/YourSite/Shared%20Documents/Reports/" & fileName
Make sure the folder “Reports” already exists in SharePoint. If not, create it manually or programmatically before uploading.
Upload Multiple Files with a Loop
If you have a list of files to upload, you can use a loop:
Sub UploadMultipleFiles()
Dim files As Variant
Dim i As Integer
files = Array("Report1.xlsx", "Report2.xlsx", "Report3.xlsx")
For i = LBound(files) To UBound(files)
Call UploadFile(files(i))
Next i
End Sub
Sub UploadFile(fileName As String)
Dim filePath As String
Dim sharePointURL As String
Dim binaryStream As Object
Dim httpRequest As Object
filePath = "C:\Reports\" & fileName
sharePointURL = "https://yourcompany.sharepoint.com/sites/YourSite/Shared%20Documents/" & fileName
Set binaryStream = CreateObject("ADODB.Stream")
With binaryStream
.Type = 1
.Open
.LoadFromFile filePath
End With
Set httpRequest = CreateObject("WinHttp.WinHttpRequest.5.1")
httpRequest.Open "PUT", sharePointURL, False
httpRequest.setRequestHeader "Content-Type", "application/octet-stream"
httpRequest.Send binaryStream.Read
If httpRequest.Status = 200 Or httpRequest.Status = 201 Then
Debug.Print fileName & " uploaded successfully."
Else
Debug.Print fileName & " upload failed. Status: " & httpRequest.Status
End If
binaryStream.Close
End Sub
This method is efficient for batch uploads using Excel.
Handling Authentication for SharePoint Online
Modern SharePoint Online may require modern authentication, especially if you’re using Multi-Factor Authentication (MFA) or your organization enforces strict policies.
VBA doesn’t natively support OAuth tokens, so in such cases, consider using:
- Microsoft Graph API with token-based authentication
- Power Automate flows triggered by Excel
- Or tools like PowerShell for more secure and scalable operations
However, for basic access, SharePoint Online may still prompt for credentials in a browser popup when WinHttpRequest
runs. If prompted, enter your SharePoint login credentials.
Tips to Successfully Upload a File to SharePoint
Best Practice | Description |
---|---|
Use correct file path | Make sure the local path exists and is accessible |
Encode URL properly | Replace spaces with %20 to avoid errors |
Check SharePoint permissions | You must have Contribute or Edit access |
Avoid invalid characters in file names | SharePoint restricts some characters like * , : , ? , " |
Set content-type header | Always set "Content-Type" to "application/octet-stream" for binary files |
Avoid very large files | Some SharePoint plans limit file sizes (check your plan) |
Common Errors and Troubleshooting
Issue | Cause | Solution |
---|---|---|
403 Forbidden | Insufficient permissions | Check SharePoint access level |
401 Unauthorized | Login required | Make sure credentials are entered when prompted |
404 Not Found | Wrong URL or folder | Double-check SharePoint path |
File not found on local path | Incorrect file path | Verify that the file exists locally |
Upload succeeds but file not visible | Cached view or wrong folder | Refresh document library and verify folder path |
Benefits of Using VBA for SharePoint Upload
- Time-saving automation of repetitive file uploads
- Integration with Excel workflows and reports
- Custom logic can be added to control file names, destinations, or conditions
- Batch uploads reduce manual errors
- Works as a lightweight alternative to SharePoint APIs for simple tasks
Final Thoughts
Uploading files to SharePoint from Excel VBA is a practical solution for many organizations managing routine reports, shared documents, and collaborative files. By using tools like WinHttpRequest or MSXML2.XMLHTTP, you can establish a connection to SharePoint and automate the upload process directly from your Excel files.
Remember to handle permissions, test file paths, and confirm that document libraries are correctly referenced. With this setup, you can efficiently manage SharePoint content without leaving Excel.
FAQs
Can I upload files to SharePoint using Excel VBA without mapping a drive?
Yes, you can upload files directly to SharePoint using Excel VBA by sending an HTTP PUT request to the document library URL. This does not require mapping SharePoint as a network drive.
Why is my VBA upload to SharePoint failing with a 401 Unauthorized error?
A 401 Unauthorized error usually means your session is not authenticated. Ensure you’re logged into SharePoint in your browser, and that your system has access via Windows authentication or valid credentials.
What VBA reference is needed to use XMLHTTP in Excel?
You need to enable the “Microsoft XML, v6.0” (or the latest available) reference in the VBA editor under Tools > References to use XMLHTTP for file uploads.
How do I handle spaces in SharePoint URLs when uploading with VBA?
Replace spaces in the SharePoint URL with %20
. For example, “Shared Documents/Monthly Reports” should be written as “Shared%20Documents/Monthly%20Reports”.
Is it better to use WebDAV mapping or HTTP PUT for uploading to SharePoint?
Using HTTP PUT is more suitable for automation without user interaction. WebDAV mapping is easier for manual file transfers but may be unstable or disconnected at times.
Can I upload multiple files to SharePoint using Excel VBA?
Yes, you can use a loop in VBA to iterate over multiple files in a folder and upload each one to SharePoint using the same method. You’ll need to update the file path and target URL dynamically in the loop.
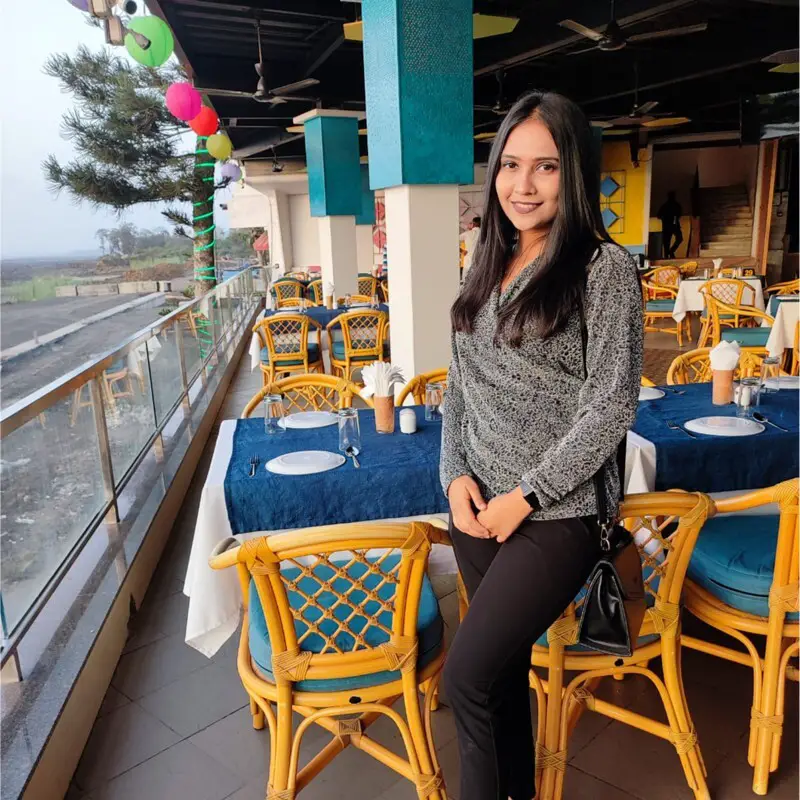
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.
By leveraging the code snippets provided in the first sources, error message as below: Path not Found.
my SharePointAddress = “https://mycompany.sharepoint.com/sites/TestDemo/Shared%20Documents/”
Sorry for late response. I was out of town for a few days.
To solve the “Path not found” error while uploading files to SharePoint using VBA, you need to ensure that the file path and SharePoint URL are correct. Try the below code to upload a file to SharePoint using VBA:
Sub UploadFileToSharePoint()
Dim filePath As String
Dim sharePointURL As String
Dim objHTTP As Object
‘ Set the file path and SharePoint URL
filePath = “C:\path\to\your\file.xlsx”
sharePointURL = “https://yourcompany.sharepoint.com/sites/YourSite/Shared%20Documents/Folder/file.xlsx”
‘ Create an instance of the MSXML2.XMLHTTP object
Set objHTTP = CreateObject(“MSXML2.XMLHTTP”)
‘ Open the HTTP request
objHTTP.Open “PUT”, sharePointURL, False
‘ Set the request headers
objHTTP.setRequestHeader “Content-Type”, “application/octet-stream”
objHTTP.setRequestHeader “If-None-Match”, “*”
‘ Send the file data
objHTTP.send CreateObject(“Scripting.FileSystemObject”).OpenTextFile(filePath, 1).ReadAll
‘ Check the response status
If objHTTP.Status = 200 Then
MsgBox “File uploaded successfully!”
Else
MsgBox “Error uploading file. Status: ” & objHTTP.Status
End If
‘ Clean up
Set objHTTP = Nothing
End Sub
Make sure to replace the following placeholders with your actual values:
– `”C:\path\to\your\file.xlsx”`: Replace with the actual file path of the file you want to upload.
– `”https://yourcompany.sharepoint.com/sites/YourSite/Shared%20Documents/Folder/file.xlsx”`: Replace with the actual SharePoint URL where you want to upload the file. Make sure to encode any special characters in the URL, such as spaces (replace them with `%20`).
Here are a few things to check if you encounter the “Path not found” error:
1. Verify that the local file path (`filePath`) is correct and that the file exists at that location.
2. Ensure that the SharePoint URL (`sharePointURL`) is valid and points to the correct location where you want to upload the file.
3. Check if you have the necessary permissions to upload files to the specified SharePoint location.
4. If you are using SharePoint Online, make sure you are properly authenticated and have the required credentials to access the SharePoint site.
If you still encounter issues, you may need to investigate further by checking the SharePoint logs or seeking assistance from your SharePoint administrator to ensure that there are no connectivity or permission-related issues.
I can’t find “Microsoft ActiveX Objects Library” anywhere. Do you know which dll it resides in so I can browse for it?
Thanks
Thanks for pointing that out! The โMicrosoft ActiveX Objects Libraryโ reference is outdated and not available in modern Excel versionsโitโs actually deprecated. Iโve now updated the blog post to use the latest supported methods (like XMLHTTP or WinHttpRequest) for uploading files to SharePoint. Thanks again for your feedback!