Why Does VBA Excel Keep Changing My Date Format to US Style?
If you’ve ever tried to work with dates in Excel using VBA (Visual Basic for Applications), you may have run into a frustrating issue – your carefully formatted international dates keep getting changed to US date format (month first) when manipulated through VBA code. This can lead to incorrect dates, calculation errors, and general confusion. In this article, we’ll explain why this happens and provide several solutions to prevent Excel VBA from changing your date formats unexpectedly.
Understanding Excel’s Date System
To grasp why VBA causes dates to change format, it’s important to understand how Excel handles dates under the hood:
- Excel stores dates as sequential serial numbers so that they can be used in calculations.
- By default, January 1, 1900 is serial number 1, and January 1, 2008 is serial number 39448 because it is 39,448 days after January 1, 1900.
- Dates before 1900 are not supported.
This serial number system allows dates to be added, subtracted, and used in formulas just like regular numbers. For example, if you have a date in cell A1, you can add 7 to it to get the date a week later.
For display purposes, these serial numbers are then formatted as dates according to the cell formatting or system locale settings. This is why the same underlying serial number can display as “8/13/2023”, “13/08/2023”, “13-Aug-2023”, or other formats depending on your Excel settings.
Why VBA Changes Date Formats
The crux of the problem is that VBA uses the US date order (month-day-year) regardless of your Windows Regional Settings. So when you write VBA code to input a date, it will treat the date as being in month-first format.
For example, let’s say your computer is set to UK date format (day-month-year). In your worksheet, you enter the date 13/08/2023, which Excel interprets as August 13, 2023. But if you read that cell value into VBA using Range("A1").Value
, VBA will interpret it as a US-style date and think you mean the 8th day of the 13th month. Since there is no 13th month, VBA throws a “Type mismatch” error.
The same issue occurs when writing dates from VBA to a worksheet. VBA will always use the US date order, so it ends up changing dates to the wrong value when it puts them in international date formatted cells.
This behavior can cause a lot of problems if your workbook uses non-US date formats. Imagine trying to calculate age from a birthdate or determine the number of days between two dates – if VBA is misinterpreting your date values, your formulas will return incorrect results.
Solutions to Prevent Date Formatting Issues
Thankfully, there are a few ways to get around this frustrating date conversion issue in VBA:
1. Use Unambiguous Date Formats
The simplest solution is to avoid ambiguity by always specifying dates in an unambiguous format, either with a 4-digit year or using named months:
- 4-digit years: Use formats like
YYYY-MM-DD
(2023-08-13) orDD-MMM-YYYY
(13-Aug-2023). These cannot be misinterpreted regardless of locale settings. - Named months: Use the
Format()
function to convert dates to a named month format that VBA will interpret correctly regardless of system settings:
Format(myDate, "dd-mmm-yyyy") '13-Aug-2023
Format(myDate, "dd/mmmm/yyyy") '13/August/2023
By using these unambiguous formats in your VBA code, you ensure that dates are always interpreted correctly.
2. Use DateSerial to Construct Dates
Instead of passing ambiguous date strings to VBA, use the DateSerial
function to explicitly define the year, month and day parts:
DateSerial(2023, 8, 13) 'returns August 13, 2023
DateSerial
takes three arguments – year, month, and day – and returns a valid Excel date serial number. This avoids any issues with string interpretation.
3. Convert String Dates Using DateValue
If you have a date in string format that you need to convert to a date value, use the DateValue
function. This will convert the string to a date using the current system’s short date format.
DateValue("13/08/2023") 'returns August 13, 2023 on a UK system
DateValue("08/13/2023") 'returns August 13, 2023 on a US system
Be aware that DateValue
will throw an error if the string is not in a format that matches the locale setting. To be safe, you can first convert strings to unambiguous formats:
DateValue(Format("13/08/2023", "yyyy-mm-dd"))
This ensures that DateValue
will work correctly regardless of the system locale.
4. Write VBA to Detect System Date Order
For more complex international applications, you can make your VBA code more robust by detecting the current locale’s date order at runtime and adjusting your code accordingly.
Here’s a simple function to determine if the system is using month-first date format:
Function IsMonthFirstFormat() As Boolean
Dim testDate As Date
testDate = DateSerial(2000, 1, 2)
If Format(testDate, "short date") Like "##/##/####" Then
IsMonthFirstFormat = True
Else
IsMonthFirstFormat = False
End If
End Function
This function creates a known date (January 2, 2000) and checks how it’s formatted in the system’s short date format. If the month comes first, it returns True
, else it returns False
.
You can then use this function to write conditional code that handles dates differently based on the system settings:
If IsMonthFirstFormat() Then
'handle dates in US format
Else
'handle dates in international format
End If
By incorporating this kind of locale awareness into your VBA code, you can create applications that work correctly with dates no matter where they’re run.
Final Thoughts
Excel’s US-centric VBA date handling is an unfortunate quirk that can throw a wrench in your international workflows. Misinterpreted dates can lead to calculation errors, data corruption, and hours of frustrating debugging.
However, by understanding how VBA treats dates, using unambiguous formats, and writing locale-aware code, you can avoid these date headaches. The key is to be explicit in your date handling, use the Format
, DateSerial
and DateValue
functions strategically, and consider the current system settings when processing date values.
FAQs
Why does VBA change my date formats to US style?
VBA uses the US date order (month-day-year) regardless of your Windows Regional Settings. When you read or write dates using VBA, it interprets them as US-style dates, which can lead to incorrect dates if your system uses a different format.
How can I prevent VBA from changing my date formats?
You can prevent VBA from changing your date formats by using unambiguous date formats (like YYYY-MM-DD), using named months (like dd-mmm-yyyy), constructing dates with the DateSerial function, or converting string dates using the DateValue function.
What happens if I read an international date into VBA?
If you read an international date (like 13/08/2023) into VBA, it will interpret it as a US-style date (August 13, 2023). If this date doesn’t exist (like 13/13/2023), VBA will throw a “Type mismatch” error.
Can I make my VBA code work with different regional settings?
Yes, you can write VBA code that detects the current system’s date order and adjusts accordingly. By using functions to check the system settings, you can make your VBA code handle dates correctly no matter where it’s run.
What is the best way to specify dates in VBA?
The best way to specify dates in VBA is to use unambiguous formats like YYYY-MM-DD or to construct dates using the DateSerial function. This ensures that your dates will be interpreted correctly regardless of the system settings.
How can I convert a string to a date in VBA?
You can convert a string to a date in VBA using the DateValue function. However, be aware that DateValue expects the string to be in the current system’s date format. To avoid issues, you can first convert the string to an unambiguous format using the Format function, like this: DateValue(Format(“13/08/2023”, “yyyy-mm-dd”)).
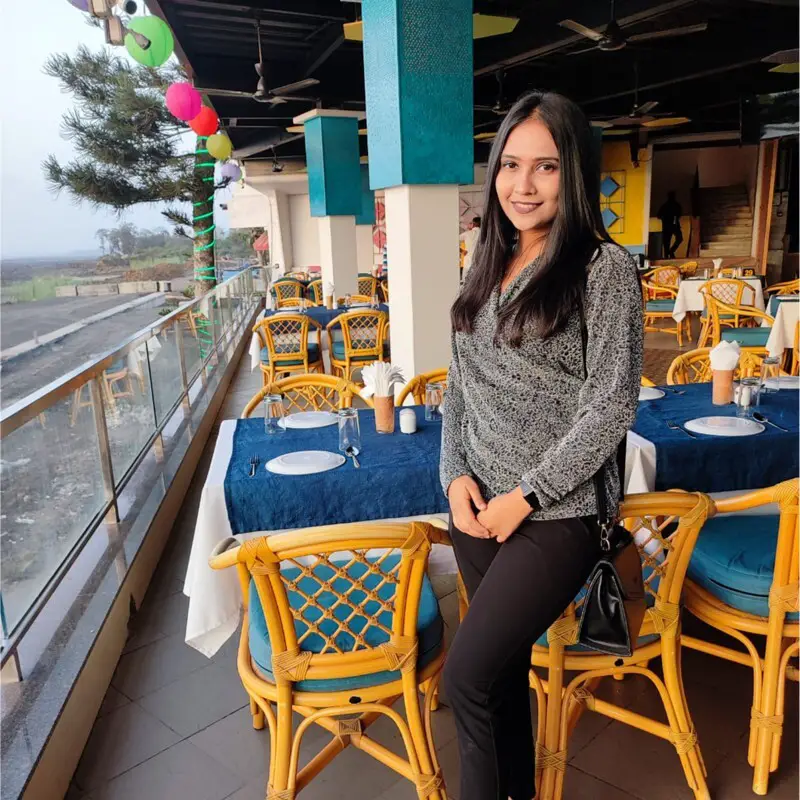
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.