Master Excel VBA on Mac: Tips & Tricks
Did you know that Excel VBA on Mac has the potential to revolutionize your spreadsheet tasks? With its powerful automation capabilities and performance-enhancing features, Excel VBA on Mac can take your productivity to new heights.
In this article, we will delve into the world of Excel VBA on Mac and provide you with expert tips and tricks to optimize your spreadsheet tasks. Whether you want to automate processes or improve the performance of your macros, we have got you covered.
Key Takeaways:
- Excel VBA on Mac offers powerful automation capabilities.
- Optimizing your Excel VBA macros can significantly improve performance.
- Working with ranges and arrays can increase the efficiency of your code.
- Following best practices is essential for maintaining optimal performance.
Understanding Excel VBA on Mac
Excel VBA on Mac is a powerful tool that can greatly enhance your productivity. With its extensive range of tools and functions, you can streamline your workflow and automate repetitive tasks. In this section, we will delve into the basics of Excel VBA on Mac and provide valuable insights on how to develop sub procedures and effectively call them.
Excel VBA on Mac offers a robust set of tools and functions that allow you to manipulate data, format worksheets, and perform complex calculations. By mastering these tools, you can create dynamic and efficient macros that save you time and effort.
Developing Sub Procedures
Sub procedures are the building blocks of Excel VBA on Mac. They are used to group a set of instructions and execute specific tasks. To develop sub procedures, you need to understand the syntax and structure of VBA code, such as variables, loops, and conditional statements.
When developing sub procedures, it is crucial to break down your tasks into smaller, manageable chunks. This will make your code more organized and easier to maintain. Additionally, using meaningful and descriptive names for your sub procedures will enhance readability and make your code more understandable for yourself and other users.
Calling Sub Procedures
Once you have developed sub procedures, you can call them within your main code or from other sub procedures. Calling a sub procedure involves using its name followed by parentheses. You can also pass arguments to the sub procedure by including them within the parentheses.
By effectively calling sub procedures, you can create modular and reusable code. This not only improves the organization of your code but also allows you to efficiently handle repetitive tasks without duplicating code.
To summarize, understanding the fundamentals of Excel VBA on Mac, including the tools and functions it offers, as well as developing and calling sub procedures, is key to harnessing the full potential of Excel VBA on Mac. In the next section, we will share valuable tips to further optimize the performance of your Excel VBA macros on Mac.
Tips to Improve Excel VBA Performance on Mac
In this section, we will share valuable tips to enhance the performance of your Excel VBA macros on Mac. By implementing these techniques, you can speed up your macros and optimize their efficiency. Let’s dive into the details:
1. Turn Off Unnecessary Features
To boost the speed of your Excel VBA macros, consider disabling unnecessary features that consume resources. For example, animations, screen updating, and automatic calculations can significantly slow down your macros. By turning off these features, you can improve the execution time of your code. Take a closer look at the following table to identify the features that you can disable:
Feature | Impact on Performance |
---|---|
Animations | Can cause delays and reduce macro execution speed. |
Screen Updating | Refreshing the screen after each action can slow down macros. |
Automatic Calculations | Recalculating formulas every time a change is made can affect performance. |
2. Remove Unnecessary Selects
When working with ranges or objects, it’s common to use the Select method. However, unnecessary selects can slow down your macros. Consider using direct references instead of selecting the object. This eliminates the need to activate or select a specific range, resulting in faster execution. By removing unnecessary selects, you can optimize your code and improve performance.
3. Utilize the With Statement
The With statement allows you to perform multiple actions on an object without repeatedly specifying the object’s name. This technique minimizes the overhead of repeatedly referencing the object, resulting in faster execution. By using the With statement, you can streamline your code and improve the performance of your Excel VBA macros on Mac.
Example:
“`vba
Sub OptimizeCode()
‘ Before optimization
Range(“A1”).Select
ActiveCell.Value = “Hello”
ActiveCell.Font.Bold = True
ActiveCell.Interior.Color = RGB(255, 0, 0)
‘ After optimization
With Range(“A1”)
.Value = “Hello”
.Font.Bold = True
.Interior.Color = RGB(255, 0, 0)
End With
End Sub
“`
By applying these tips, you can enhance the performance of your Excel VBA macros on Mac and execute your tasks more efficiently. Stay tuned for the next section where we will explore advanced techniques for working with ranges and arrays in Excel VBA on Mac.
Working with Ranges and Arrays in Excel VBA on Mac
Working with ranges and arrays in Excel VBA on Mac can significantly improve the efficiency of your code. By reducing the number of times data is transferred between VBA and Excel, you can greatly increase the performance of your macros.
In this section, we will explain how to read and write data to ranges using arrays, instead of individual cell operations. This technique will help you process data more efficiently in Excel VBA on Mac.
Reading Data from Ranges Using Arrays
When working with large sets of data in Excel VBA on Mac, it is common to loop through each cell in a range to read its value. However, this approach can be time-consuming, especially when dealing with thousands of cells.
An efficient alternative is to read the entire range into an array and then process the data in the array. This reduces the number of data transfers between VBA and Excel, resulting in faster execution times. Here’s an example:
“`vba
Dim dataRange As Range
Dim dataArray As Variant
Dim i As Long
‘ Set the data range
Set dataRange = Range(“A1:A1000”)
‘ Read the data into an array
dataArray = dataRange.Value
‘ Process the data in the array
For i = 1 To UBound(dataArray, 1)
‘ Do something with dataArray(i, 1)
Next i
“`
In the example above, we first set the data range to be read (in this case, cells A1:A1000). We then use the `Value` property of the range to read the data into a variant array called `dataArray`.
We can then loop through the elements of the array using the `UBound` function and process the data as needed. This approach eliminates the need for individual cell operations, leading to significant performance improvements in your Excel VBA macros on Mac.
Writing Data to Ranges Using Arrays
Similar to reading data, writing data to ranges using arrays can greatly enhance the efficiency of your Excel VBA macros on Mac. Instead of writing data to each cell individually, you can write the entire array to the range in one go.
Here’s an example:
“`vba
Dim dataRange As Range
Dim dataArray(1 To 1000, 1 To 1) As Variant
Dim i As Long
‘ Generate data for the array
For i = 1 To 1000
dataArray(i, 1) = i
Next i
‘ Set the data range
Set dataRange = Range(“A1:A1000”)
‘ Write the data from the array to the range
dataRange.Value = dataArray
“`
In the example above, we generate data for the array using a loop and assign it to the `dataArray`. We then set the data range to which the array will be written (in this case, cells A1:A1000) and use the `Value` property to write the entire array to the range.
By using this approach, you can reduce the data transfer between VBA and Excel, resulting in faster execution times and improved performance of your Excel VBA macros on Mac.
By leveraging ranges and arrays in Excel VBA on Mac, you can effectively reduce data transfer and increase the efficiency of your code. Whether you are reading or writing data, using arrays can significantly improve the performance of your Excel VBA macros on Mac.
Stay tuned for the next section where we will share best practices for Excel VBA on Mac to further optimize your code and enhance your skills.
Best Practices for Excel VBA on Mac
When it comes to mastering Excel VBA on Mac, following best practices is crucial. By incorporating these practices into your code, you can optimize performance and ensure maintainability in your Excel VBA projects.
One important practice is using Option Explicit
to catch undeclared variables. This forces you to declare all variables explicitly, preventing potential errors and enhancing the readability of your code. Additionally, it is recommended to use the .Value2
property instead of .Text
or .Value
when working with cell values. This can improve the speed of your macros and prevent unexpected behavior.
Another best practice is to bypass the clipboard when copying and pasting data. Instead of using the clipboard, you can directly transfer data between ranges or arrays in your code. This eliminates unnecessary steps and reduces processing time, resulting in faster and more efficient macros.
By implementing these best practices, you can optimize your Excel VBA code, enhance performance, and create more robust solutions. Whether you are automating processes or improving the functionality of your spreadsheets, following these best practices will elevate your skills and make your Excel VBA projects on Mac even more impactful.
FAQ
How can Excel VBA on Mac help me automate processes and improve performance?
Excel VBA on Mac offers a wide array of tools and functions that can enhance your productivity. By leveraging its power, you can automate processes and optimize your spreadsheet tasks.
What are the basics of Excel VBA on Mac?
In Excel VBA on Mac, you can develop sub procedures and effectively call them to streamline your workflow. Understanding these fundamentals will empower you to make the most of Excel VBA on Mac.
How can I improve the performance of my Excel VBA macros on Mac?
To speed up your macros, you can turn off unnecessary features like animations, screen updating, and automatic calculations. Additionally, removing unnecessary selects and using the With statement can optimize your code for efficiency.
How can I work with ranges and arrays in Excel VBA on Mac?
By working with ranges and arrays, you can significantly improve the efficiency of your code. This involves reading and writing data to ranges using arrays, rather than individual cell operations, which can process data more efficiently in Excel VBA on Mac.
What are the best practices for Excel VBA on Mac?
It is important to follow best practices in Excel VBA on Mac to ensure optimal performance and maintainability. These include using Option Explicit to catch undeclared variables, using .Value2 instead of .Text or .Value, and bypassing the clipboard when copying and pasting data.
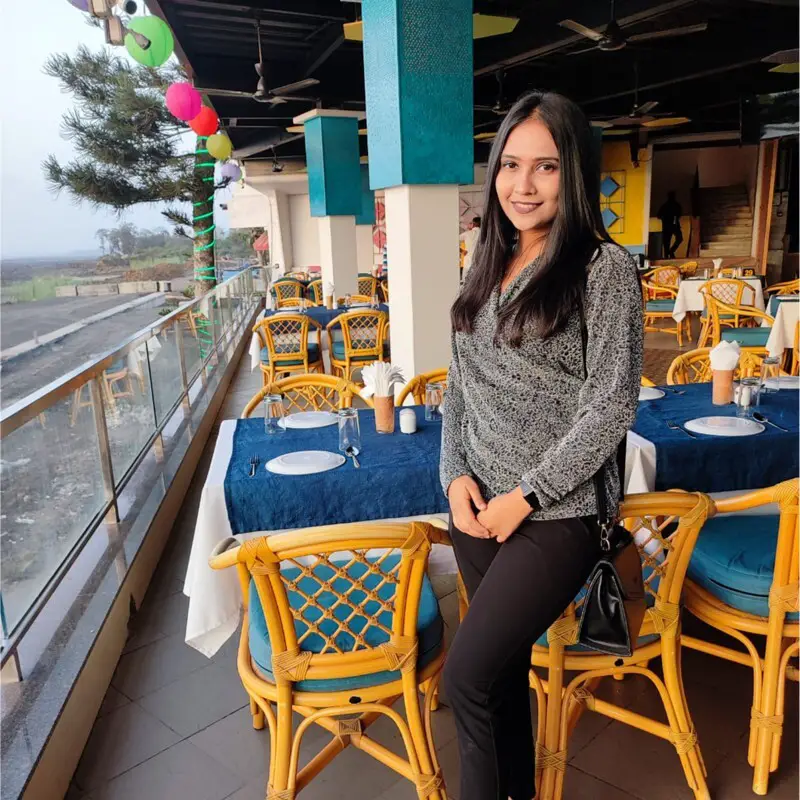
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.