How to Create an Excel VBA Prompt for Users to Select from a List?
When working with Excel VBA (Visual Basic for Applications), you may want to prompt the user to select an option from a predefined list. This can be useful for creating interactive spreadsheets, gathering user input, or building custom Excel applications. In this article, we’ll explore different methods to create an Excel VBA prompt that allows users to choose from a list of options.
Understanding the VBA InputBox Function
The simplest way to prompt users for input in Excel VBA is by using the built-in InputBox
function. The InputBox
function displays a dialog box with a message and an input field, allowing users to enter a value. However, the basic InputBox
function does not provide a dropdown list for users to select from.
Here’s the syntax for the InputBox
function:
InputBox(prompt, [title], [default], [xpos], [ypos], [helpfile], [context])
prompt
: The message displayed in the dialog box.title
(optional): The title of the dialog box.default
(optional): The default value displayed in the input field.xpos
andypos
(optional): The x and y coordinates of the dialog box.helpfile
andcontext
(optional): Help file and context information.
While the InputBox
function is straightforward to use, it lacks the functionality to present a list of options for users to choose from. Let’s explore alternative methods to achieve this.
Method 1: Using a Custom UserForm
One approach to create an Excel VBA prompt with a list of options is by designing a custom UserForm. A UserForm allows you to create a graphical user interface (GUI) with various controls, including listboxes, comboboxes, and buttons.
Here’s a step-by-step guide to create a UserForm with a listbox:
- In the Visual Basic Editor (VBE), go to Insert > UserForm to create a new UserForm.
- From the Toolbox, drag and drop a ListBox control onto the UserForm.
- Right-click the ListBox and select Properties to customize its appearance and behavior.
- Set the
RowSource
property to a range containing the list of options or populate the list programmatically using theAddItem
method. - Add a CommandButton to the UserForm for the user to confirm their selection.
- Write the necessary VBA code to handle the button click event and retrieve the selected value from the ListBox.
Here’s a code example that demonstrates the process:
Private Sub UserForm_Initialize()
'Populate the ListBox with options
ListBox1.AddItem "Option 1"
ListBox1.AddItem "Option 2"
ListBox1.AddItem "Option 3"
End Sub
Private Sub CommandButton1_Click()
'Retrieve the selected value from the ListBox
Dim selectedValue As String
selectedValue = ListBox1.Value
'Do something with the selected value
MsgBox "You selected: " & selectedValue
'Close the UserForm
Unload Me
End Sub
Using a custom UserForm provides flexibility in designing the user interface and allows for more complex interactions beyond a simple list selection.
Method 2: Using the Application.InputBox Method
Another approach to prompt users with a list of options is by leveraging the Application.InputBox
method in Excel VBA. This method is an enhanced version of the regular InputBox
function and supports a Type
parameter that allows you to specify the type of input expected from the user.
Here’s the syntax for the Application.InputBox
method:
Application.InputBox(prompt, [title], [default], [left], [top], [helpfile], [helpcontextID], [type])
The type
parameter is of particular interest here. By setting type
to 8
, you can display a dropdown list for the user to select from. The list of options is provided as a comma-separated string in the default
parameter.
Here’s an example of how to use the Application.InputBox
method with a dropdown list:
Sub PromptWithList()
Dim options As String
Dim selectedValue As String
options = "Option 1,Option 2,Option 3"
selectedValue = Application.InputBox("Select an option:", "Dropdown List", options, , , , , 8)
If selectedValue <> "False" Then
MsgBox "You selected: " & selectedValue
Else
MsgBox "No option selected."
End If
End Sub
In this example, the options
variable holds a comma-separated string of the list options. The Application.InputBox
method is called with the type
parameter set to 8
, indicating a dropdown list. The selected value is stored in the selectedValue
variable, which can be further processed as needed.
Method 3: Using the VBA ListBox Function
Excel VBA provides a built-in ListBox
function that allows you to create a listbox directly within a cell. The ListBox
function returns the selected value from the list.
Here’s the syntax for the ListBox
function:
ListBox(choices, [multi_select], [title], [default], [cancel], [width], [height])
choices
: An array or range containing the list of options.multi_select
(optional): Specifies whether multiple selections are allowed.title
(optional): The title of the listbox.default
(optional): The default item selected in the listbox.cancel
(optional): Specifies the action when the user cancels the listbox.width
andheight
(optional): The width and height of the listbox in points.
Here’s an example of how to use the ListBox
function:
Sub PromptWithListBox()
Dim options As Variant
Dim selectedValue As String
options = Array("Option 1", "Option 2", "Option 3")
selectedValue = Application.WorksheetFunction.ListBox(options, , "Select an Option")
If selectedValue <> "" Then
MsgBox "You selected: " & selectedValue
Else
MsgBox "No option selected."
End If
End Sub
In this example, the options
array holds the list of options. The ListBox
function is called with the options
array as the choices
argument. The selected value is stored in the selectedValue
variable.
Best Practices and Considerations
When creating an Excel VBA prompt for users to select from a list, consider the following best practices and considerations:
- Provide clear instructions: Ensure that the prompt message clearly communicates what the user is expected to do and how to interact with the list.
- Handle user cancellation: Implement appropriate error handling to gracefully handle scenarios where the user cancels the prompt or closes the dialog box without making a selection.
- Validate user input: If applicable, validate the user’s selection to ensure it meets any required criteria or constraints.
- Consider the user experience: Design the prompt in a way that enhances the user experience and aligns with the overall look and feel of your Excel application.
- Test thoroughly: Test your VBA code extensively to ensure it functions as expected across different scenarios and user interactions.
Final Thoughts
Creating an Excel VBA prompt for users to select from a list offers a convenient way to gather user input and enhance the interactivity of your Excel applications. Whether you choose to use a custom UserForm, the Application.InputBox
method, or the ListBox
function, each approach has its own advantages and use cases.
Remember to consider the specific requirements of your project, the level of customization needed, and the ease of implementation when choosing the most suitable method for your Excel VBA prompt. With the power of VBA and the ability to prompt users for list selections, you can create robust and interactive Excel applications that cater to your users’ needs.
FAQs
What is the simplest way to prompt users for input in Excel VBA?
InputBox
function. However, the basic InputBox
function does not provide a dropdown list for users to select from.How can I create a custom UserForm to prompt users with a list of options?
- In the Visual Basic Editor (VBE), go to Insert > UserForm to create a new UserForm.
- From the Toolbox, drag and drop a ListBox control onto the UserForm.
- Right-click the ListBox and select Properties to customize its appearance and behavior.
- Set the
RowSource
property to a range containing the list of options or populate the list programmatically using theAddItem
method. - Add a CommandButton to the UserForm for the user to confirm their selection.
- Write the necessary VBA code to handle the button click event and retrieve the selected value from the ListBox.
Can I use the Application.InputBox method to prompt users with a dropdown list?
Application.InputBox
method in Excel VBA to prompt users with a dropdown list. By setting the type
parameter to 8
, you can display a dropdown list for the user to select from. The list of options is provided as a comma-separated string in the default
parameter.Is there a built-in VBA function to create a listbox within a cell?
ListBox
function that allows you to create a listbox directly within a cell. The ListBox
function returns the selected value from the list.What are some best practices to consider when creating an Excel VBA prompt for users to select from a list?
- Provide clear instructions: Ensure that the prompt message clearly communicates what the user is expected to do and how to interact with the list.
- Handle user cancellation: Implement appropriate error handling to gracefully handle scenarios where the user cancels the prompt or closes the dialog box without making a selection.
- Validate user input: If applicable, validate the user’s selection to ensure it meets any required criteria or constraints.
- Consider the user experience: Design the prompt in a way that enhances the user experience and aligns with the overall look and feel of your Excel application.
- Test thoroughly: Test your VBA code extensively to ensure it functions as expected across different scenarios and user interactions.
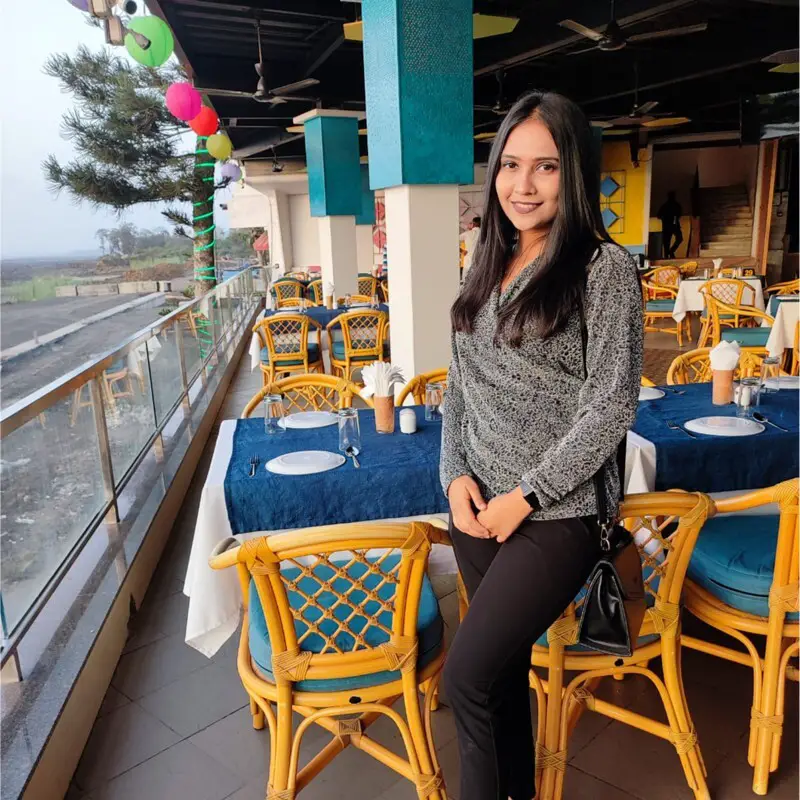
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.