Excel VBA: Populate ListBox from Table – A Complete Guide
If you’re working with Excel VBA and want to populate a ListBox from a table in an efficient manner, you’re in the right place. In this guide, we will walk you through the steps needed to achieve this, ensuring your ListBox dynamically reflects the data in your table. Whether you’re building a user interface or managing data, this method will streamline your tasks and improve the interactivity of your Excel applications.
What You’ll Learn
- How to populate a ListBox from a table using Excel VBA.
- Understanding the key concepts of VBA and ListBox.
- Step-by-step implementation guide.
- Tips to optimize your VBA code for better performance.
Let’s get started by understanding the basics of a ListBox and how it interacts with tables in Excel VBA.
Understanding ListBox in Excel VBA
A ListBox is a control element in Excel that allows users to select one or more items from a list. It is widely used in user forms or can be embedded directly into Excel sheets. By populating a ListBox from a table, you can create dynamic, interactive forms that are easy to update and manage.
Why Populate a ListBox from a Table?
- Dynamic Data Management: As the table updates, the ListBox automatically reflects the changes.
- Enhanced User Experience: Provides users with a clear, concise way to select from predefined options.
- Ease of Use: Simplifies the process of managing large datasets.
Step-by-Step Guide to Populate ListBox from Table Using Excel VBA
1) Setting Up Your Excel Environment
Before we start writing VBA code, ensure your Excel workbook is ready. Here’s what you need to do:
- Create a Table: Ensure your data is structured as a table in Excel. To convert a range of cells into a table, select the range and press
Ctrl + T
. - Insert a ListBox: Go to the Developer tab, click on Insert, and choose ListBox under Form Controls. Place the ListBox on your worksheet or in a user form.
2) Writing the VBA Code
Now, let’s write the VBA code to populate the ListBox from the table. We will use the List
property of the ListBox to fill it with data from the table.
Step 1: Access the VBA Editor
Press Alt + F11
to open the VBA editor. Insert a new module by going to Insert > Module.
Step 2: Define Your VBA Macro
Below is a simple VBA macro to populate a ListBox from a table:
Sub PopulateListBoxFromTable()
Dim ws As Worksheet
Dim tbl As ListObject
Dim ListBox As Object
Dim r As Range
Dim i As Long
' Set the worksheet and table references
Set ws = ThisWorkbook.Sheets("Sheet1")
Set tbl = ws.ListObjects("Table1")
Set ListBox = ws.ListBoxes("ListBox1")
' Clear the ListBox
ListBox.Clear
' Loop through each row in the table and add it to the ListBox
For i = 1 To tbl.ListRows.Count
ListBox.AddItem tbl.ListRows(i).Range.Cells(1, 1).Value
Next i
End Sub
Explanation:
- ws: Refers to the worksheet containing the table and ListBox.
- tbl: Refers to the table from which data will be populated.
- ListBox: Refers to the ListBox that will display the table data.
- For Loop: Iterates through each row of the table, adding the first column’s data to the ListBox.
3) Running the VBA Code
To execute the macro, return to Excel, press Alt + F8
, select PopulateListBoxFromTable
, and click Run. Your ListBox should now be populated with data from the first column of your table.
4) Populating ListBox with Multiple Columns
If you want to display multiple columns from the table in the ListBox, modify the code slightly:
Sub PopulateListBoxWithMultipleColumns()
Dim ws As Worksheet
Dim tbl As ListObject
Dim ListBox As Object
Dim r As Range
Dim i As Long
' Set the worksheet and table references
Set ws = ThisWorkbook.Sheets("Sheet1")
Set tbl = ws.ListObjects("Table1")
Set ListBox = ws.ListBoxes("ListBox1")
' Configure ListBox for multiple columns
ListBox.ColumnCount = tbl.ListColumns.Count
' Clear the ListBox
ListBox.Clear
' Loop through each row in the table and add the entire row to the ListBox
For i = 1 To tbl.ListRows.Count
ListBox.AddItem
For j = 1 To tbl.ListColumns.Count
ListBox.List(i - 1, j - 1) = tbl.ListRows(i).Range.Cells(1, j).Value
Next j
Next i
End Sub
5) Optimizing the VBA Code for Better Performance
To ensure that your ListBox loads quickly and efficiently, consider these optimization tips:
- Disable Screen Updating: This prevents Excel from refreshing the screen during code execution, speeding up the process.
Application.ScreenUpdating = False
- Use With Statements: Simplify repetitive code by using
With
statements.
With ListBox
.Clear
' Additional code here
End With
- Limit Data Loading: If your table is large, consider loading only the necessary data into the ListBox.
Handling Common Issues While Populating ListBox from Table
1) ListBox Not Populating
If your ListBox isn’t populating as expected, check the following:
- Table Name: Ensure the table name in your code matches the table name in Excel.
- ListBox Name: Verify the ListBox name in the code matches the ListBox name in Excel.
- Range References: Double-check that the range references in your code are correct.
2) Populating ListBox from a Dynamic Table
If your table changes size frequently, adjust your code to handle dynamic ranges. Use the Resize
method to dynamically set the table range.
Set r = tbl.DataBodyRange.Resize(tbl.ListRows.Count, tbl.ListColumns.Count)
3) Adding a Search Functionality to the ListBox
You can enhance your ListBox by adding a search feature. Here’s how to do it:
- Add a TextBox above the ListBox.
- Write a macro to filter the ListBox items based on the TextBox input.
Private Sub TextBox1_Change()
Dim i As Long
Dim strSearch As String
strSearch = TextBox1.Text
ListBox1.Clear
For i = 1 To tbl.ListRows.Count
If InStr(1, tbl.ListRows(i).Range.Cells(1, 1).Value, strSearch, vbTextCompare) > 0 Then
ListBox1.AddItem tbl.ListRows(i).Range.Cells(1, 1).Value
End If
Next i
End Sub
Summary
Populating a ListBox from a table using Excel VBA is a powerful way to create dynamic and interactive Excel applications. With the steps outlined above, you can quickly set up a ListBox to display data directly from a table, ensuring your data is always up-to-date and easily accessible to users.
Key Takeaways
- Use the
List
property to efficiently populate a ListBox. - Modify the code to handle multiple columns if necessary.
- Optimize the VBA code for better performance.
- Troubleshoot common issues by checking ListBox and table references.
- Enhance your ListBox by adding features like search functionality.
FAQs
How do I populate a ListBox from a table using Excel VBA?
You can populate a ListBox from a table in Excel VBA by using the ListBox’s List
property and looping through the rows of your table to add each row’s data to the ListBox. The article provides a detailed step-by-step guide on how to do this.
How can I populate a ListBox with multiple columns from a table?
To populate a ListBox with multiple columns from a table, you need to set the ColumnCount
property of the ListBox to match the number of columns in your table. The VBA code in the article demonstrates how to loop through each row and column to populate the ListBox with multiple columns.
Why is my ListBox not populating with data?
If your ListBox is not populating, check that the table and ListBox names in your VBA code match those in your Excel workbook. Also, ensure that the table range and references are correct. The article covers common troubleshooting steps for these issues.
How do I optimize my VBA code for faster ListBox population?
To optimize your VBA code for faster ListBox population, you can disable screen updating, use With
statements to simplify your code, and limit the amount of data being loaded into the ListBox. These tips are explained in detail in the article.
Can I add search functionality to a ListBox in Excel VBA?
Yes, you can add search functionality to a ListBox in Excel VBA by using a TextBox control to filter the ListBox items as the user types. The article includes a sample VBA macro that demonstrates how to implement this feature.
How do I handle dynamic tables when populating a ListBox?
To handle dynamic tables, you can adjust your VBA code to accommodate changes in the table size by using the Resize
method. This ensures that your ListBox remains accurate even as the table grows or shrinks. The article provides a detailed explanation of how to do this.
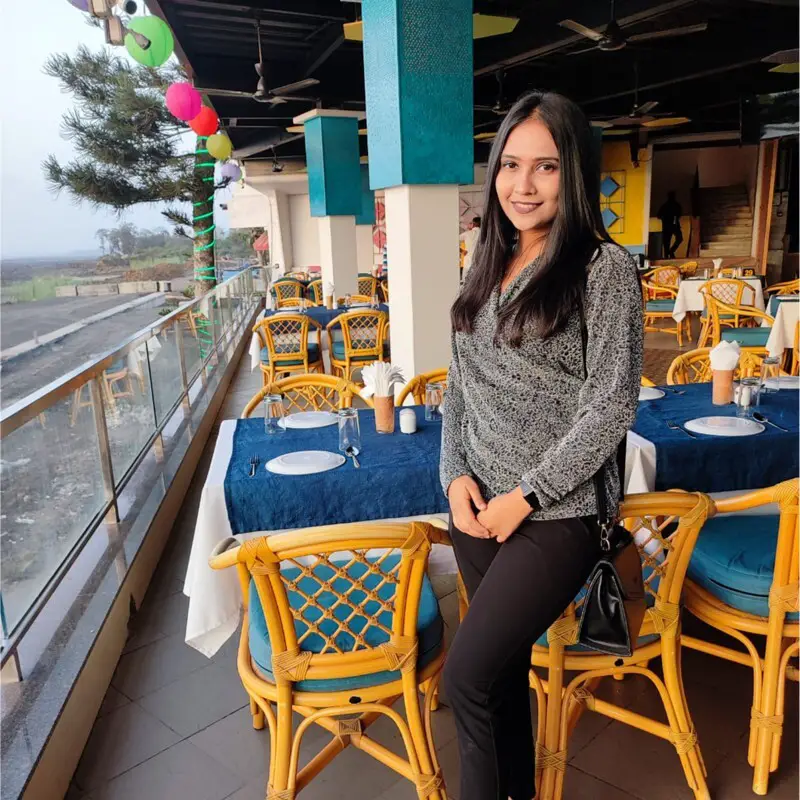
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.
It is very interesting that your explanation and examples never actually show how to populate a listbox from a TABLE, as the title of this article suggests. Do you know what a TABLE is? It is not a list hard coded into the VBA script. It suggests that a table or named range is populated with data and somehow it is possible to pull that data into the listbox for use at runtime. If the data in the named range changes, the resulting items in the listbox would change also, but that is not what you are discussing, is it? Thanks for trying to help here but adding items to a listbox in DEVELOPMENT is far different from adding items to a listbox dynamically at runtime.
This post was long overdue for updation (as our VBA developer was on medical leave). I have updated the post to answer your queries. Thanks for commenting!