Troubleshooting Excel VBA On Error Resume Next
Did you know that the use of On Error Resume Next in Excel VBA is often plagued with issues, with the code not resuming at the next line as expected? This surprising fact highlights the importance of understanding the common problems associated with this statement and finding solutions to ensure smooth execution of your VBA code.
Key Takeaways:
- On Error Resume Next is used to ignore runtime errors and continue code execution in VBA.
- Common issues with On Error Resume Next include nested error handling and improper closure of error handling blocks.
- Proper error handling practices, such as specific error handling blocks and informative error messages, are recommended.
- Troubleshoot On Error Resume Next issues by checking for nested error handling blocks and verifying VBA settings.
- Consider alternative error handling approaches based on the complexity and requirements of your code.
Understanding On Error Resume Next in VBA
The On Error Resume Next statement is a powerful tool for handling errors in VBA code. When an error occurs, this statement allows the code execution to continue from the next line instead of halting and displaying an error message. This can be particularly useful in situations where you want to bypass errors and proceed with the execution of your code.
With On Error Resume Next, you can effectively suppress errors and prevent abrupt termination of your program. Instead of stopping at the point of error, the code moves on to the next line, allowing you to gracefully handle errors and maintain the workflow of your program.
However, it is important to exercise caution when using On Error Resume Next. While it can be a convenient way to handle errors, it can also lead to unexpected behavior if used incorrectly. It is essential to understand its limitations and implement proper error handling techniques to ensure the stability and reliability of your VBA code.
Benefits and Use Cases
The On Error Resume Next statement offers several benefits and can be used in various scenarios:
- Error Ignoring: By using this statement, you can ignore specific errors and allow the code execution to continue without interruptions. This can be helpful when dealing with non-critical errors or when error handling is not necessary for a particular task.
- Bulk Operations: When performing large-scale operations, such as data processing or file operations, using On Error Resume Next can help you handle errors gracefully without disrupting the entire operation. This allows you to handle errors on a case-by-case basis and proceed with the operation.
- Legacy Code: In some cases, you may be working with legacy code or code that has evolved over time. Introducing proper error handling throughout the codebase may be time-consuming or impractical. On Error Resume Next can act as a temporary solution to keep the code running until a more comprehensive error handling strategy can be implemented.
While On Error Resume Next can be a useful feature, it is important to remember that it is not a substitute for proper error handling. It should be used as a complement to well-defined error handling routines to maintain code robustness and minimize unexpected behavior.
Pros and Cons of On Error Resume Next | |
---|---|
Pros | Cons |
|
|
Common Issues with On Error Resume Next
While the On Error Resume Next statement in Excel VBA is a powerful tool for handling errors, there are some common issues that may arise when using it. Understanding these issues can help you troubleshoot and resolve any problems you encounter.
Nested Error Handling
One common issue is when there is nested error handling, where one error handling block is inside another. In this case, the error handler inside the inner block will take precedence and the code will not resume at the next line. This can lead to unexpected behavior and make it difficult to identify and fix errors. It is important to carefully review your code and ensure that the error handling blocks are properly structured and nested.
Improper Error Handling Closure
Another issue occurs when the error handling is not properly closed off before the next line of code. The On Error Resume Next statement should always be followed by an On Error GoTo 0 statement to reset the error handling. If this is not done, the code may not resume at the next line and errors may not be handled as intended.
To fix this issue, make sure that every On Error Resume Next statement is followed by an On Error GoTo 0 statement to properly reset the error handling.
By addressing these common issues and ensuring proper error handling in your code, you can make the most of the On Error Resume Next statement and effectively handle errors in your Excel VBA applications.
Common Issues with On Error Resume Next | Solutions |
---|---|
Nested Error Handling | Review and restructure error handling blocks |
Improper Error Handling Closure | Always include On Error GoTo 0 statement after On Error Resume Next |
Best Practices for Error Handling in VBA
While utilizing the On Error Resume Next statement can be advantageous in specific scenarios, it is generally recommended to exercise caution and adhere to best practices for error handling in VBA. By following these best practices, you can ensure robust and reliable error handling in your code. Some key practices to consider include:
- Implementing specific error handling blocks for different types of errors: It is crucial to identify and handle different types of errors separately to provide appropriate responses and actions.
- Displaying informative error messages: Clear and concise error messages help users understand the issue, facilitating troubleshooting and resolving errors effectively.
- Logging errors for troubleshooting purposes: By logging errors, you can capture valuable information about the occurrence of errors, aiding in identifying patterns and potential areas for code improvement.
- Properly handling errors within functions and subroutines: Errors that occur within functions and subroutines should be handled explicitly to prevent any unexpected behavior or issues.
By embracing these best practices, you can enhance the error handling capabilities of your VBA code, leading to more reliable and maintainable solutions.
Error Handling Examples
Let’s take a look at a few examples to demonstrate how to implement best practices for error handling in VBA:
Error Type | Best Practice | Example Code |
---|---|---|
File Not Found | Display an error message and provide an option to choose a different file. | On Error GoTo FileNotFound ' Code to open the file Exit Sub FileNotFound: MsgBox "File not found. Please choose a different file.", vbExclamation ' Code to prompt user for another file |
Division by Zero | Inform the user about the error and provide guidance on resolving it. | On Error GoTo DivideByZero ' Code to perform division Exit Sub DivideByZero: MsgBox "Division by zero error. Please provide a non-zero denominator.", vbExclamation ' Code to handle division by zero |
Invalid Input | Validate user input and prompt for correct input if invalid. | On Error GoTo InvalidInput ' Code to process user input Exit Sub InvalidInput: MsgBox "Invalid input. Please enter a valid value.", vbExclamation ' Code to handle invalid input and prompt for correct input |
These examples showcase how specific error handling blocks can be implemented to handle various types of errors, providing informative feedback to users and facilitating seamless execution of code.
Troubleshooting On Error Resume Next Issues
If you are experiencing issues with the On Error Resume Next statement in your Excel VBA code not working as expected, there are a few troubleshooting steps you can take to resolve the problem.
Step 1: Check for Nested Error Handling Blocks
One common reason why On Error Resume Next may not work is when there are nested error handling blocks within your code. Ensure that there are no inner error handling blocks that take precedence over the outer one. If there are, make sure to properly close off the innermost error handling block before the next line of code.
Step 2: Verify VBA Error Handling Configuration
Another potential issue could be the VBA setting for error handling. In some cases, a specific setting can cause On Error statements to be ignored, resulting in the display of a dialog box instead. To use On Error Resume Next, make sure this setting is disabled.
Step | Action |
---|---|
Step 1 | Check for nested error handling blocks |
Step 2 | Verify VBA error handling configuration |
By following these troubleshooting steps, you can identify and resolve any issues you may be facing with the On Error Resume Next statement in Excel VBA.
Alternatives to On Error Resume Next
While On Error Resume Next can be useful in certain situations, there are alternative approaches to error handling in VBA that may be more appropriate depending on the specific requirements of your code. Here are some alternatives to consider:
- Structured Error Handling with On Error GoTo: This approach allows you to specify a specific label or line number to jump to when an error occurs. By using the On Error GoTo statement, you can have more control over how errors are handled and take appropriate action based on the specific error that occurred.
- Implementing Custom Error Handling Routines: Instead of relying solely on the default error handling behavior, you can create custom error handling routines to handle different types of errors in a more specific and controlled manner. This allows you to provide user-friendly error messages and perform additional actions, such as logging the error or notifying the user.
- Using Specific Error Handling Functions or Methods: Excel VBA provides various built-in functions and methods that can be used for error handling. For example, you can use the Err object to retrieve information about the most recent error that occurred, or the Err.Raise method to raise a specific error manually. These functions and methods offer more flexibility in handling errors based on your specific needs.
When choosing an error handling approach, it’s important to consider the complexity and requirements of your code. Analyze the specific scenarios where errors can occur and determine the most suitable alternative that provides sufficient error handling and control.
Conclusion
The On Error Resume Next statement is a powerful tool in Excel VBA that allows you to bypass runtime errors and continue with the execution of your code. However, it is crucial to use this statement judiciously and understand its limitations to avoid unexpected behavior in your code.
If you are encountering issues with On Error Resume Next not working as expected, it is recommended to follow the troubleshooting steps outlined in the previous sections. By identifying and resolving any underlying problems, you can ensure that your code resumes execution at the desired line.
In some cases, it may be beneficial to explore alternative error handling approaches that better align with your specific requirements. These alternatives, such as structured error handling with On Error GoTo or custom error handling routines, can provide more control and flexibility in managing errors in your VBA code.
By implementing effective error handling techniques and choosing the appropriate approach for your code, you can enhance the robustness and reliability of your Excel VBA projects. Remember, error handling is a critical aspect of software development, and investing time in proper error handling can save you valuable time in the long run.
FAQ
Why is On Error Resume Next not working in Excel VBA?
There could be several reasons why On Error Resume Next is not working as expected. One common issue is when there are nested error handling blocks within the code. Another issue could be that the error handling is not properly closed off before the next line of code. It is important to troubleshoot and resolve these issues to ensure the proper functionality of On Error Resume Next.
How can I troubleshoot issues with On Error Resume Next in Excel VBA?
To troubleshoot issues with On Error Resume Next, you can check for nested error handling blocks within your code and ensure that the innermost block is properly closed off. Additionally, verify the VBA setting for error handling to make sure it is correctly configured. Disabling a specific setting can cause On Error statements to be ignored and display a dialog box instead.
Is On Error Resume Next the best approach for error handling in VBA?
While On Error Resume Next can be useful in certain scenarios, there are alternative approaches to error handling in VBA that may be more appropriate depending on the specific requirements of your code. Some alternatives include using structured error handling with On Error GoTo, implementing custom error handling routines, or using specific error handling functions or methods provided by Excel VBA. It is important to choose the right error handling approach based on the complexity and requirements of your code.
What are some best practices for error handling in VBA?
Some best practices for error handling in VBA include using specific error handling blocks for different types of errors, displaying informative error messages, and logging errors for troubleshooting purposes. It is also important to properly handle errors within functions and subroutines to prevent unexpected behavior.
How can I ensure the robustness and reliability of my VBA code?
By implementing effective error handling techniques, such as using the appropriate error handling approach for your code, following best practices for error handling, and properly troubleshooting any issues with On Error Resume Next, you can ensure the robustness and reliability of your VBA code.
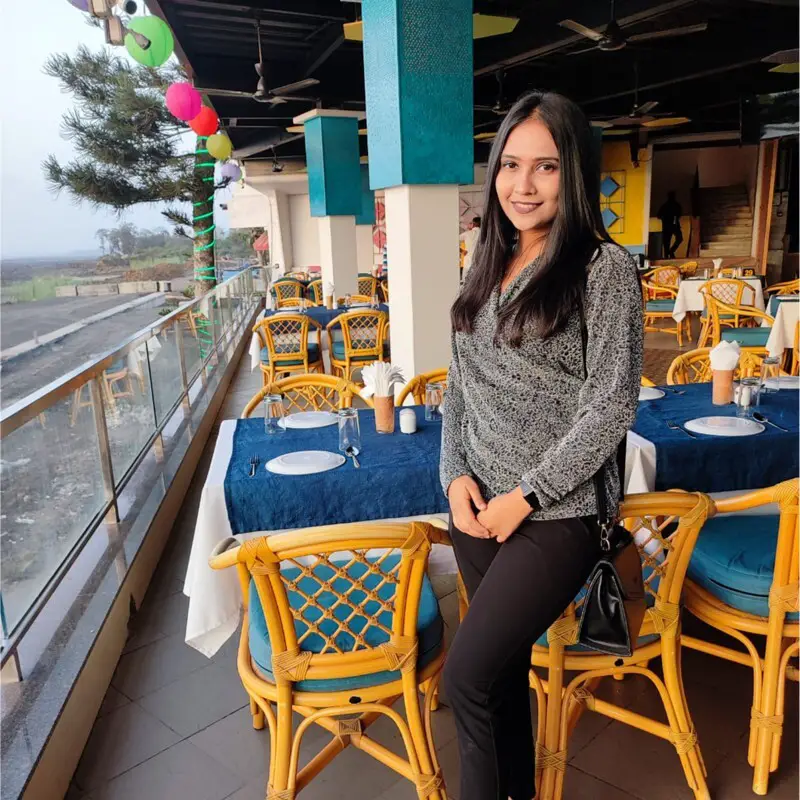
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.