Excel VBA: Ensure Queries Finish Before Proceeding
Did you know that in Excel VBA, the data accuracy and consistency of subsequent code execution can be compromised if queries are not given enough time to finish refreshing? It’s crucial to ensure that queries are fully refreshed before proceeding to prevent any inconsistencies in your data analysis or reporting.
In this article, we will explore various techniques to ensure that queries finish refreshing in Excel VBA. Whether you need to refresh all queries in the workbook, refresh specific queries, or wait for SQL queries to complete, we’ve got you covered with practical solutions and code examples.
Key Takeaways:
- Ensuring that queries finish refreshing before proceeding is crucial for data accuracy in Excel VBA.
- The ‘RefreshAll’ method can refresh all queries in the workbook simultaneously.
- The ‘Refresh’ method allows you to refresh specific queries in a controlled order.
- The ‘QueryTable.Refresh’ method is used for refreshing SQL queries and can be combined with the ‘BackgroundQuery’ property to wait for data fetch completion.
- Disabling background refresh for all queries or utilizing additional techniques like introducing delays or processing pending events can also help make VBA wait for query refresh.
RefreshAll method
The RefreshAll
method is a powerful tool in Excel VBA for quickly refreshing all queries in a workbook at once. By using this method, you can ensure that all data sources are updated before moving on to the next steps in your code.
When you call the RefreshAll
method, VBA will iterate through all the queries in the workbook and initiate a refresh. This means that the data from each query will be fetched from its source and updated in the worksheet. This ensures that all the queries are refreshed and ready for further processing.
It is important to be aware that the execution time of the RefreshAll
method can vary depending on the number and complexity of the queries in your workbook. If you have a large number of queries or queries with complex data sources, the refresh process may take some time to complete.
Here is an example of the VBA code for refreshing all queries in Excel:
Sub RefreshAllQueries()
ThisWorkbook.RefreshAll
End Sub
Method | Description |
---|---|
RefreshAll | Refreshes all queries in the workbook simultaneously |
Refresh method for specific queries
If you want to specifically refresh certain queries in Excel VBA, you can use the Connections(name).Refresh
method. The “name” parameter represents the name of the query connection that you want to refresh. By calling this method for each individual query, you have the flexibility to control the order in which the queries are refreshed. VBA will wait for each query to finish refreshing before moving on to the next one, ensuring that the data from each query is up to date before proceeding with the rest of the code execution.
When refreshing specific queries, it’s important to note that the refresh process may take some time, especially for queries with complex data sources or large data sets. Therefore, it’s recommended to consider the potential impact on the overall execution time of your code and optimize it accordingly.
Here’s an example of VBA code that demonstrates the use of the Connections(name).Refresh
method to refresh a specific query:
Sub RefreshSpecificQuery()
Dim conn As WorkbookConnection
Set conn = ThisWorkbook.Connections("Query1") ' Replace "Query1" with the actual name of your query connection
conn.Refresh
End Sub
By customizing the query name within the code above, you can refresh the specific query that matches the provided name. This code ensures that the data from the selected query is refreshed before proceeding with the rest of the VBA code execution.
The example image above showcases a relevant scenario where a specific query is being refreshed using the Connections(name).Refresh
method.
QueryTable.Refresh method for SQL queries
If your queries are based on SQL queries, the QueryTable.Refresh
method in Excel VBA can be used to refresh the tables generated from these queries. By setting the BackgroundQuery
property of the table’s QueryTable
object to False
, VBA will wait until the data has been fully fetched before proceeding. This ensures that the table is refreshed and up to date before further code execution.
Here is an example of VBA code for refreshing an SQL query-based table:
Sub RefreshSQLQueryTable()
Dim table As ListObject
Set table = Worksheets("Sheet1").ListObjects("Table1")
table.QueryTable.Refresh BackgroundQuery:=False
End Sub
In the above code, “Sheet1” represents the worksheet containing the table, and “Table1” is the name of the table. Replace these with the actual sheet and table names you are working with. By setting the BackgroundQuery
property to False
, VBA will wait for the query to finish refreshing before moving on to the next line of code.
It is important to note that the QueryTable.Refresh
method only works for tables that are based on SQL queries, not for other types of data sources.
By using the QueryTable.Refresh
method in Excel VBA, you can ensure that your SQL query-based tables are always up to date before proceeding with any further processing or analysis.
Disabling background refresh for all queries
Another effective method to ensure that queries finish before proceeding in Excel VBA is to disable the background refresh option for all queries in the workbook. By unchecking the “Enable background refresh” checkbox in the query properties, VBA will wait for each query to finish refreshing before moving on, as the background refresh option is disabled.
This approach is particularly useful when you have a large number of queries and want to refresh them all at once. Disabling the background refresh option ensures that VBA waits for each query to complete before moving on to the next one, guaranteeing accurate and up-to-date data throughout your code execution.
However, it is important to note that disabling background refresh may increase the overall execution time of your code. VBA will wait for each query to finish refreshing before proceeding to the next step, which may introduce a slight delay. Therefore, it’s recommended to consider the trade-off between data accuracy and the potential impact on code execution time.
- Select the query you want to modify.
- Go to the “Data” tab in the Excel ribbon.
- Click on the “Connections” button in the “Connections” group.
- In the “Workbook Connections” dialog box, select the query you want to modify and click on the “Properties” button.
- In the “Connection Properties” dialog box, go to the “Usage” tab.
- Uncheck the “Enable background refresh” checkbox.
- Click “OK” to save the changes.
By following these steps, you can disable background refresh for all queries in Excel VBA. This ensures that VBA will wait for each query to finish refreshing, guaranteeing accurate results and preventing any potential data inconsistencies.
Additional techniques for waiting for query refresh
In addition to the techniques mentioned above, there are other ways to make VBA wait for a query to finish refreshing. These techniques can be useful in scenarios where you need more control over the wait time or when the query refresh process triggers other events that need to be processed before proceeding with the code execution.
One option is to use the Application.Wait
method to introduce a desired delay in the code execution. By specifying a wait time, VBA will pause the code execution for the specified duration, allowing the query to finish refreshing. However, it’s important to note that this method introduces a fixed delay, regardless of how long the query actually takes to refresh. Therefore, you may need to experiment with different wait times to ensure that the query has enough time to fully refresh.
Another option is to use the DoEvents
function, which allows VBA to process any pending events before moving on. This can be particularly useful when the query refresh process triggers other events or updates that need to be processed before proceeding with the code execution. By using DoEvents
, VBA will give priority to processing these events, ensuring that the query refresh completes before moving on.
It’s important to assess your specific scenario and choose the technique that best suits your needs. Whether it’s introducing a delay with Application.Wait
or prioritizing pending events with DoEvents
, these additional techniques provide flexibility in ensuring that VBA code waits for the query to finish refreshing before proceeding.
FAQ
How can I ensure that queries finish refreshing before proceeding with further code execution in VBA?
There are several techniques you can use to ensure that queries finish refreshing in VBA. One option is to use the `RefreshAll` method to refresh all queries in the workbook simultaneously. Another option is to refresh a specific query using the `Refresh` method. Additionally, you can use the `QueryTable.Refresh` method to refresh tables based on SQL queries. Disabling the background refresh option for all queries is another approach. You can also introduce a delay using the `Application.Wait` method or use the `DoEvents` function to process pending events.
How do I refresh all queries in VBA?
To refresh all queries in VBA, you can use the `RefreshAll` method. This method will iterate through all the queries in the workbook and update their data sources. It is important to note that the execution time of this method may vary depending on the complexity and number of queries in the workbook.
How can I refresh a specific query in VBA?
If you only want to refresh a specific query in VBA, you can use the `Connections(name).Refresh` method, where “name” is the name of the query connection. This allows you to control which queries are refreshed and in what order. By calling this method for each individual query, VBA will wait for each query to finish refreshing before moving on to the next one.
How do I refresh tables based on SQL queries in VBA?
To refresh tables that are based on SQL queries in VBA, you can use the `QueryTable.Refresh` method. By setting the `BackgroundQuery` property of the table’s `QueryTable` object to False, VBA will wait until the data has been fully fetched before moving on.
How can I disable background refresh for all queries in VBA?
To disable background refresh for all queries in VBA, you can uncheck the “Enable background refresh” checkbox in the query properties. By doing this, VBA will wait for each query to finish refreshing before moving on. However, it is important to note that this method may increase the overall execution time of the code.
Are there any additional techniques for waiting for query refresh in VBA?
Yes, there are other techniques you can use to make VBA wait for a query to finish refreshing. One option is to introduce a delay using the `Application.Wait` method. Another option is to use the `DoEvents` function, which allows VBA to process any pending events before moving on. These techniques can be useful in specific scenarios where you need to synchronize the code execution with the query refresh process.
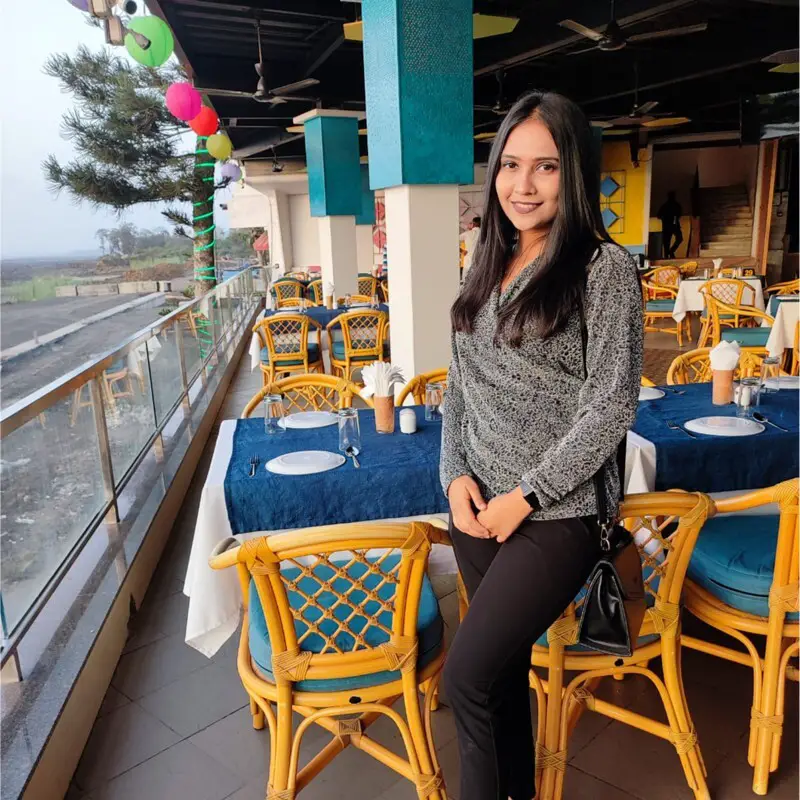
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.