Excel VBA Copy Data from Multiple Workbooks
Did you know that Excel VBA (Visual Basic for Applications) can help you copy data from multiple workbooks with just a few lines of code? This powerful feature allows you to save time and effort by automating the copying process, leaving you more time to focus on analyzing and interpreting your data.
Key Takeaways:
- Excel VBA enables you to copy data from multiple workbooks into one master workbook.
- By creating a VBA code, you can specify the source and destination ranges and automate the copying process.
- Consider using Paste Special options to control how the data is pasted, such as values, formulas, and formats.
- Handle variable ranges in VBA code to accommodate varying data sizes in source and destination workbooks.
- Follow best practices when using VBA macros for copying data, such as using error handling and optimizing code efficiency.
Steps to Copy Data from Multiple Workbooks
To copy data from multiple workbooks using Excel VBA, follow these steps:
- Open the VBA editor in Excel by pressing Alt + F11.
- Create a new module by clicking on Insert and selecting Module.
- Write the VBA code to open each workbook and specify the source and destination ranges.
- Use the
Workbooks.Open
method to open each workbook. - Use the
Set
keyword to assign the workbook to a variable. - Specify the source range using the
Range
property. - Specify the destination range using the
Range
property in the master workbook. - Use the
Copy
method to copy the data from the source range to the destination range.
- Use the
- Repeat step 3 for each workbook you want to copy data from.
- Close the VBA editor.
- Run the VBA code by pressing F5 or clicking on the Run button.
Example:
Here is an example of VBA code that copies data from multiple workbooks:
Sub CopyDataFromWorkbooks()
Dim MasterWorkbook As Workbook
Dim SourceWorkbook As Workbook
Dim SourceRange As Range
Dim DestinationRange As Range
' Set the master workbook
Set MasterWorkbook = ThisWorkbook
' Open the source workbook
Set SourceWorkbook = Workbooks.Open("C:\Path\to\SourceWorkbook.xlsx")
' Set the source range
Set SourceRange = SourceWorkbook.Worksheets("Sheet1").Range("A1:B10")
' Set the destination range in the master workbook
Set DestinationRange = MasterWorkbook.Worksheets("Sheet1").Range("D1:E10")
' Copy the data from the source range to the destination range
SourceRange.Copy DestinationRange
' Close the source workbook without saving changes
SourceWorkbook.Close SaveChanges:=False
' Repeat the above steps for each additional workbook
End Sub
By following these steps and using the provided VBA code, you can easily copy data from multiple workbooks into one master workbook using Excel VBA. This can save you time and effort, especially when dealing with large amounts of data.
Writing the VBA Code
To copy data from multiple workbooks using VBA code, you need to follow a specific syntax. Here’s how you can write the VBA code:
- Open the Visual Basic for Applications (VBA) editor by pressing Alt + F11 in Excel.
- Create a new module by clicking on Insert > Module.
- Define your variables to store workbook and worksheet references.
- Use the
Workbooks.Open
method to open each workbook that you want to copy data from. Specify the file path and name of each workbook. - Set your source and destination ranges using the
Range
object. - Use the
Range.Copy
method to copy the data from the source range. - Use the
Range.PasteSpecial
method to paste the data into the destination range. You can specify the paste type as values, formulas, formats, etc. - Close the source workbooks using the
Workbook.Close
method. - Repeat the above steps for each workbook you want to copy data from.
- Save and run the VBA code to copy the data from multiple workbooks.
By following this syntax, you can automate the process of copying data from multiple workbooks using VBA code.
Using the provided image, you can see an example of VBA code for copying data from multiple workbooks. Make sure to tailor the code according to your specific workbook names, ranges, and objectives.
Next, let’s explore some considerations to keep in mind when copying data from multiple workbooks using VBA macros.
Considerations When Copying Data
When copying data from multiple workbooks using VBA macros in Excel, there are a few important considerations to keep in mind:
- File paths: Ensure that the file paths of the source workbooks are correct and accessible from the destination workbook. This will ensure that the VBA code can locate and open all the necessary workbooks.
- Data range: Determine the range or ranges of data that you want to copy from each workbook. This could be a specific worksheet, a range of cells, or multiple worksheets with different ranges. Make sure to define the correct range in the VBA code to avoid any issues with data extraction.
- Data structure: Consider the structure of the data in the source workbooks and how it will be organized in the destination workbook. Ensure that the destination workbook has the necessary worksheets and columns to accommodate the copied data.
- Data formatting: Take into account any formatting applied to the data in the source workbooks, such as number formats, cell colors, and font styles. Decide whether you want to preserve or modify the formatting when copying the data to the destination workbook.
- Error handling: Implement error handling mechanisms in your VBA code to handle any unexpected issues that may arise during the copying process. This will help prevent crashes and ensure a smoother execution of the macro.
By considering these factors when copying data from multiple workbooks using VBA macros in Excel, you can ensure a more efficient and accurate data transfer process.
Paste Special Options
When pasting data from multiple workbooks using VBA, you have the option to use Paste Special to control how the data is pasted. This feature allows you to customize the copying process according to your needs. By specifying the PasteSpecial method in your VBA code, you can choose to paste values, formulas, formats, and more.
Here are some of the options you can utilize with Paste Special:
- Values: This option allows you to paste only the values from the source workbook, removing any formulas or formatting. It is useful when you want to retain the data but eliminate any underlying calculations.
- Formulas: With this option, you can paste the formulas from the source workbook into the destination workbook, preserving the dynamic nature of the data.
- Formats: This option enables you to copy the formatting properties along with the data. It ensures that the destination workbook maintains the same appearance as the source workbook.
- Column Widths: By selecting this option, you can copy the column widths from the source workbook to the destination workbook, ensuring consistent column sizes.
- Transpose: The Transpose option allows you to switch the orientation of the data by copying it vertically instead of horizontally, or vice versa.
To specify the desired Paste Special option in your VBA code, use the PasteSpecial method followed by the specific option. For example:
Worksheets("Sheet1").Range("A1").PasteSpecial Paste:=xlPasteValues
By incorporating Paste Special options into your VBA macros, you can have greater control over the data copying process, resulting in more efficient and tailored outcomes.
Handling Variable Ranges
If the size of your data ranges in the source and destination workbooks varies each time you run the macro, you can use VBA code to handle variable ranges. By finding the last used row in both the source and destination sheets, you can copy and paste the data below the existing data in the destination sheet.
To handle variable ranges in Excel VBA, follow these steps:
- Identify the last used row in the source sheet using the
LastRow
variable. - Specify the source range using the identified last row.
- Identify the last used row in the destination sheet using the
LastRowDest
variable. - Specify the destination range using the identified last row in the destination sheet.
- Copy the data from the source range to the destination range using the
Copy
method.
Here’s an example of how the VBA code would look:
Sub CopyData()
Dim LastRow As Long
Dim LastRowDest As Long
LastRow = Worksheets("SourceSheet").Cells(Rows.Count, 1).End(xlUp).Row
LastRowDest = Worksheets("DestinationSheet").Cells(Rows.Count, 1).End(xlUp).Row + 1
Worksheets("SourceSheet").Range("A1:B" & LastRow).Copy _
Destination:=Worksheets("DestinationSheet").Range("A" & LastRowDest)
End Sub
The code above finds the last used row in both the source sheet and the destination sheet. It then copies the data from the range A1:B in the source sheet and pastes it below the last used row in the destination sheet.
Using this VBA code, you can easily handle variable ranges and copy data from multiple workbooks into one master workbook. This saves you time and effort by automating the data copying process.
Additional Tips and Best Practices
When working with VBA macros to copy data from multiple workbooks, it’s important to follow some best practices to ensure the smooth execution of your code and achieve optimal results.
1. Error Handling: Incorporate error handling into your VBA code to anticipate and handle any potential errors or exceptions that may occur during the copying process. This will help prevent unexpected crashes and ensure the integrity of your data.
2. Code Optimization: Take the time to optimize your VBA code for efficiency. Avoid unnecessary loops or redundant lines of code that can slow down the execution time. Use variables to store values and references, and consider using arrays for better performance when dealing with large datasets.
3. Data Validation: Before copying the data, perform data validation to ensure its accuracy and integrity. Check for inconsistencies, invalid values, or missing information that may affect the quality of the data in the destination workbook. Implement appropriate validation checks or error handling routines to address these issues.
4. Testing and Debugging: Test your VBA macros thoroughly before deploying them in a production environment. Use debug tools such as breakpoints and watches to step through the code and identify any errors or unexpected behavior. Validate the copied data against the source workbooks to ensure its accuracy and completeness.
By following these tips and best practices, you can enhance the reliability and efficiency of your VBA macros for copying data from multiple workbooks. Incorporating error handling, optimizing your code, validating the data, and testing thoroughly will help you achieve smooth and accurate data transfers, saving you time and effort in your data management tasks.
FAQ
Can I use Excel VBA to copy data from multiple workbooks into one master workbook?
Yes, you can use Excel VBA to automate the process of copying data from multiple workbooks into one master workbook.
How do I copy data from multiple workbooks using Excel VBA?
To copy data from multiple workbooks using Excel VBA, follow these steps:
What syntax do I need to use when writing the VBA code for copying data from multiple workbooks?
To write the VBA code for copying data from multiple workbooks, you will need to use the following syntax:
What should I consider when copying data from multiple workbooks using VBA macros?
When copying data from multiple workbooks using VBA macros, there are a few things to consider:
Can I control how the data is pasted when copying from multiple workbooks using VBA?
Yes, when pasting data from multiple workbooks using VBA, you have the option to use Paste Special to control how the data is pasted.
How do I handle variable ranges when copying data from multiple workbooks using VBA code?
If the size of your data ranges in the source and destination workbooks varies each time you run the macro, you can use VBA code to handle variable ranges.
Do you have any additional tips and best practices for copying data from multiple workbooks using VBA macros?
Yes, here are some additional tips and best practices for copying data from multiple workbooks using VBA macros:
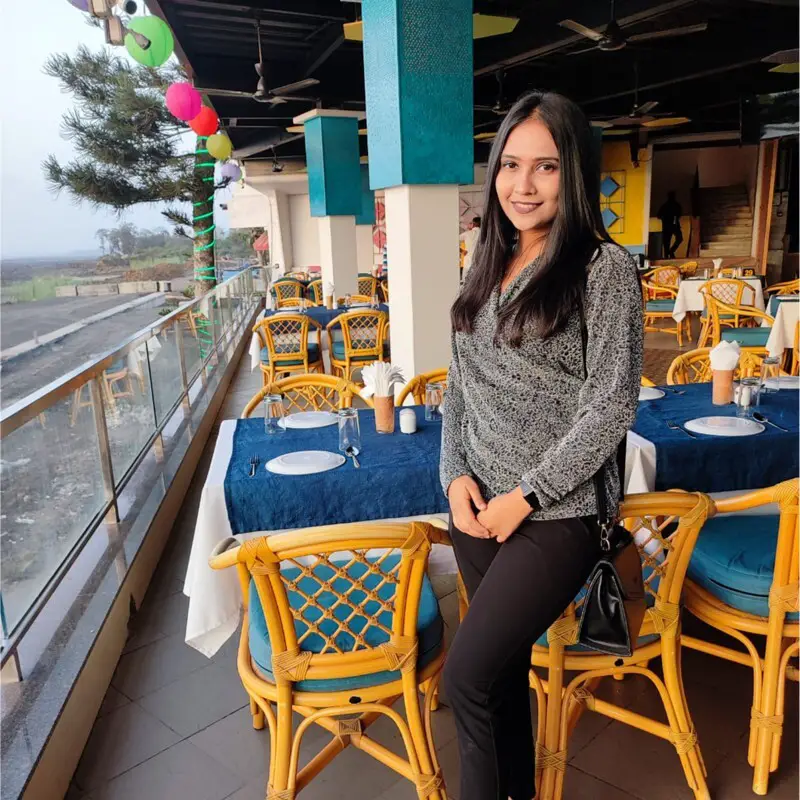
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.