How to Find All Rows with a Specific Value in Excel VBA?
In this article, we’ll explain how to use Excel VBA to find all rows that contain a specific value in a worksheet. This is a common task when working with large datasets in Excel. Using VBA code, you can quickly search through your data and locate the rows you need, saving time and effort compared to manually scanning through the spreadsheet.
Understanding the VBA Find Method
At the core of finding rows with a specific value in Excel VBA is the versatile Find method. This method enables you to search for a particular value within a specified range of cells. Here’s the full syntax of the Find method:
range.Find(What, [After], [LookIn], [LookAt], [SearchOrder], [SearchDirection], [MatchCase], [MatchByte], [SearchFormat])
While the What parameter is mandatory and specifies the value to search for, the other parameters are optional and provide additional control over the search behavior:
Parameter | Description |
---|---|
After | The cell after which to start the search |
LookIn | Specifies whether to search in cell values, formulas, or comments |
LookAt | Determines whether to match the whole cell content or just part of it |
SearchOrder | Specifies whether to search by rows or columns |
SearchDirection | Indicates whether to search from the start or end of the range |
MatchCase | Determines whether to match the case of the search value |
MatchByte | Used for double-byte character sets |
SearchFormat | Specifies whether to search for cell formats in addition to values |
In most cases, you’ll only need to use a subset of these parameters to find the rows you’re interested in. We’ll focus on the key parameters needed for common search scenarios.
Step-by-Step: Finding Rows with a Specific Value
Now, let’s dive into the step-by-step process of finding all rows that contain a particular value using VBA in Excel:
Step 1: Define the Search Parameters
Before writing any code, it’s crucial to determine what exactly you want to search for. This will be the value you pass to the What parameter of the Find method. Additionally, consider the following:
- The range of cells you want to search in (a single column, multiple columns, the entire used range of the worksheet, etc.)
- Whether you need to match the case of the value
- Whether you want to find cells where the value is contained within the cell contents or if it needs to be an exact match
Step 2: Start the VBA Code
To begin writing your VBA code, open the Visual Basic Editor in Excel by pressing Alt+F11 or navigating to Developer > Visual Basic. In the Project Explorer, right-click on your workbook and insert a new module. In the code window, start defining a new macro using the Sub keyword followed by a descriptive name for your macro, like:
Sub FindRows()
End Sub
Step 3: Define Variables
Inside your macro, declare variables to store the essential items for your search:
- A Range object variable to represent the range of cells you want to search
- A String variable to hold the search value
- A Range object variable to store the result of the Find method
For example:
Dim searchRange As Range
Dim searchValue As String
Dim foundCell As Range
Step 4: Assign the Search Range and Value
Next, assign the appropriate values to the searchRange and searchValue variables. For this example, let’s assume we want to search the entire used range of the active worksheet for the value “John”:
Set searchRange = ActiveSheet.UsedRange
searchValue = "John"
Step 5: Find the First Match
Now, use the Find method to locate the first cell containing the search value within the specified range:
Set foundCell = searchRange.Find(What:=searchValue, LookIn:=xlValues, LookAt:=xlWhole, MatchCase:=False)
This line of code searches the used range for the value “John”, looking specifically in cell values, matching the whole contents of cells, and ignoring the case.
The result of the Find method will be stored in the foundCell variable. If no match is found, foundCell will be Nothing.
Step 6: Find All Other Matches
Assuming the first match was found successfully, you can proceed to loop through the range and find any additional matches. To avoid an infinite loop, store the address of the first found cell, then use the FindNext method starting from the cell after the found cell to continue the search:
If Not foundCell Is Nothing Then
Dim firstAddress As String
firstAddress = foundCell.Address
Do
'Process the found cell here
Set foundCell = searchRange.FindNext(foundCell)
Loop While Not foundCell Is Nothing And foundCell.Address <> firstAddress
End If
Within the loop, you can perform any desired actions on each found cell, such as copying its row to a new location, adding it to a results list, or applying formatting. The loop will continue until no more matches are found or the search circles back to the first found cell.
Step 7: Close the Macro
Finally, make sure to properly end your macro with the End Sub keywords.
Complete VBA Code Example
Here’s a complete example of a VBA macro that finds all rows containing the value “John” and copies them to a new worksheet:
Sub FindRows()
Dim searchRange As Range
Dim searchValue As String
Dim foundCell As Range
Set searchRange = ActiveSheet.UsedRange
searchValue = "John"
Set foundCell = searchRange.Find(What:=searchValue, LookIn:=xlValues, LookAt:=xlWhole, MatchCase:=False)
If Not foundCell Is Nothing Then
Dim resultSheet As Worksheet
Set resultSheet = ThisWorkbook.Sheets.Add(After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count))
resultSheet.Name = "Search Results"
Dim copyRowIndex As Long
copyRowIndex = 1
Dim firstAddress As String
firstAddress = foundCell.Address
Do
foundCell.EntireRow.Copy Destination:=resultSheet.Rows(copyRowIndex)
copyRowIndex = copyRowIndex + 1
Set foundCell = searchRange.FindNext(foundCell)
Loop While Not foundCell Is Nothing And foundCell.Address <> firstAddress
End If
End Sub
This macro searches for “John” in the used range of the active sheet, creates a new worksheet named “Search Results,” and copies each row containing “John” to the new sheet.
Tips for Finding Rows in Excel VBA
To further enhance your VBA code for finding rows in Excel, consider the following tips:
- Be specific with your search range – Searching the entire used range can be slow for very large datasets. If you know the specific column(s) the value is likely to be in, set your range accordingly to improve performance.
- Use error handling – Wrap your Find operations in error handling code using On Error statements to gracefully handle scenarios where no matches are found and avoid run-time errors.
- Optimize for performance – If you need to search for multiple values, it’s often more efficient to use AutoFilter on your data instead of performing repeated Find operations.
- Consider using a Dictionary – If you frequently need to look up rows based on a key value, copying the relevant data into a Dictionary object can enable much faster lookups compared to searching the worksheet each time.
- Leverage wildcard characters – The Find method supports the use of wildcard characters like
*
(matches any number of characters) and?
(matches a single character). Use these to perform partial or flexible matches. - Utilize the After parameter – If you know the approximate location of the value you’re searching for, you can use the After parameter to start the search from a specific cell, potentially speeding up the process.
Real-World Examples
To illustrate the practical applications of finding rows with a specific value in Excel VBA, let’s explore a couple of real-world examples:
Example 1: Extracting Data for a Specific Customer
Suppose you have a large worksheet containing sales data for multiple customers. You need to extract all the rows related to a specific customer and copy them to a separate sheet for further analysis.
Sub ExtractCustomerData()
Dim customerName As String
customerName = "ABC Company"
Dim salesData As Range
Set salesData = ActiveSheet.UsedRange
Dim foundCell As Range
Set foundCell = salesData.Find(What:=customerName, LookIn:=xlValues, LookAt:=xlWhole, MatchCase:=False)
If Not foundCell Is Nothing Then
Dim customerSheet As Worksheet
Set customerSheet = ThisWorkbook.Sheets.Add(After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count))
customerSheet.Name = customerName
Dim copyRow As Long
copyRow = 1
Dim firstAddress As String
firstAddress = foundCell.Address
Do
foundCell.EntireRow.Copy Destination:=customerSheet.Rows(copyRow)
copyRow = copyRow + 1
Set foundCell = salesData.FindNext(foundCell)
Loop While Not foundCell Is Nothing And foundCell.Address <> firstAddress
End If
End Sub
Example 2: Highlighting Overdue Tasks
Let’s say you have a task list in Excel with a column indicating the due date for each task. You want to highlight all the rows where the due date has passed, i.e., the tasks are overdue.
Sub HighlightOverdueTasks()
Dim taskList As Range
Set taskList = ActiveSheet.UsedRange
Dim foundCell As Range
Set foundCell = taskList.Find(What:="Overdue", LookIn:=xlValues, LookAt:=xlWhole, MatchCase:=False)
If Not foundCell Is Nothing Then
Dim firstAddress As String
firstAddress = foundCell.Address
Do
foundCell.EntireRow.Interior.Color = RGB(255, 0, 0)
Set foundCell = taskList.FindNext(foundCell)
Loop While Not foundCell Is Nothing And foundCell.Address <> firstAddress
End If
End Sub
These examples demonstrate how the techniques covered in this article can be adapted to solve various real-world problems in Excel using VBA.
Final Thoughts
In this article, we’ve explored how to leverage the power of Excel VBA’s Find method to locate and manipulate rows containing a specific value. By following the step-by-step guide and code examples provided, you can efficiently search through your data and take action on the matching rows.
While the Find approach is effective for many scenarios, it’s important to consider performance implications when working with very large datasets. In such cases, exploring alternative techniques like AutoFilter or using a Dictionary for lookups can lead to more optimized solutions.
Remember to adapt the code examples to fit your specific use case, handle potential errors, and optimize your search range and parameters for the best results. With a solid understanding of finding rows using VBA, you’ll be well-equipped to tackle a wide range of data manipulation tasks in Excel.
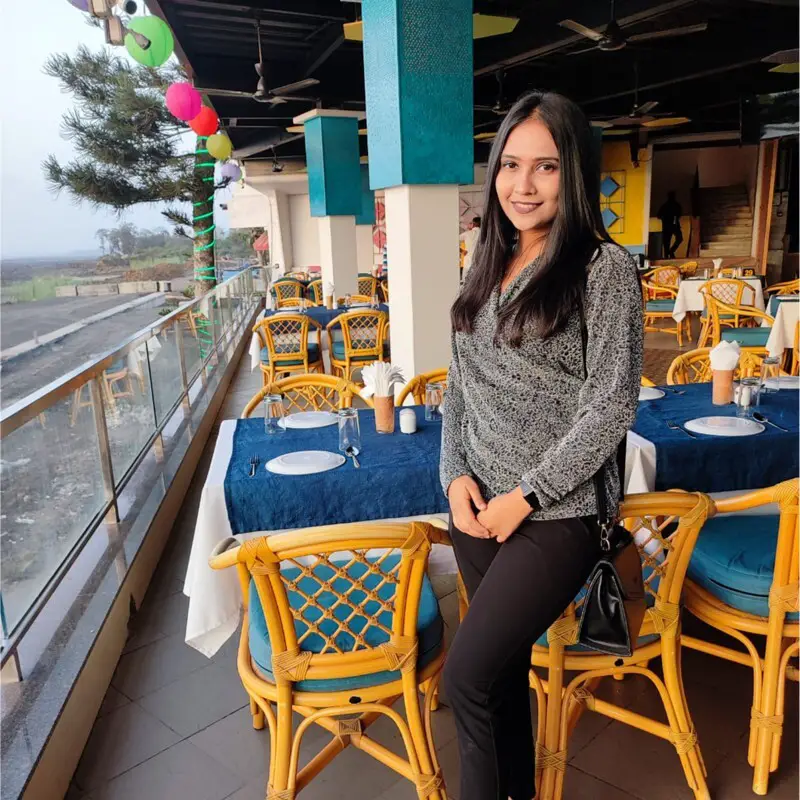
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.