How to Paste Values Without Formatting in Excel Using VBA?
Do you need to paste data from one part of an Excel workbook to another without carrying over the source formatting? Excel VBA provides a simple way to paste values without formatting, allowing you to cleanly transfer data while preserving the destination worksheet’s existing formatting. In this article, we’ll show you exactly how to use VBA code to paste values without formatting in Excel.
Understanding the PasteSpecial Method in Excel VBA
The key to pasting values without formatting lies in the PasteSpecial method. This powerful VBA method gives you fine-grained control over how content is pasted in Excel. With the right parameters, you can specify to only paste the values and not copy over the source formatting.
The basic syntax for the PasteSpecial method in Excel VBA is:
Range("destination_range").PasteSpecial Paste:=xlPasteValues
Let’s break this down:
Range("destination_range")
specifies the cell or range where you want to paste the data. Replace “destination_range” with the actual cell reference, like “A1” or “B2:D10”..PasteSpecial
is the VBA method that enables pasting with special options.Paste:=xlPasteValues
tells Excel to only paste the values, ignoring any formatting.
By setting Paste:=xlPasteValues
, you ensure only the raw data is pasted without any formatting coming along for the ride. This is the crucial piece for pasting values without formatting in VBA.
Why Paste Values Without Formatting?
There are many situations where you might want to paste only the values and not the formatting when working with data in Excel. Here are a few common scenarios:
- Preserving Existing Formatting: If you’ve carefully formatted a worksheet and want to paste new data into it without disrupting that formatting, pasting values is the way to go. This lets you update the data while keeping your worksheet looking consistent.
- Removing Unwanted Formatting: Sometimes you copy data from a source that has formatting you don’t want, like odd fonts, colors, or cell styles. Pasting only the values gives you a clean slate to work with in your destination worksheet.
- Pasting into Templates: If you have a nicely formatted template where you want to paste raw data, using PasteSpecial with xlPasteValues ensures your template formatting stays intact while the data gets updated.
- Avoiding Formula Conflicts: When you copy cells that contain formulas and paste them elsewhere, the formulas will adjust to the new location, which can sometimes cause errors or unexpected results. Pasting only the values avoids this issue, as it transfers the computed value, not the underlying formula.
By pasting values without formatting, you can sidestep a variety of formatting issues and keep your worksheets looking polished and consistent. Excel VBA makes this process quick and painless.
Step-by-Step Example: Pasting Values Without Formatting
Now let’s walk through a concrete example to demonstrate how to paste values without formatting using VBA in Excel. Imagine you have data in the range A1:C10 that you want to paste into the range E1:G10, but you don’t want the formatting from A1:C10 to overwrite the formatting in E1:G10.
Here are the steps to accomplish this:
- First, copy the source data that you want to paste. In VBA, you would use code like this:
Range("A1:C10").Copy
- Then, use the PasteSpecial method to paste only the values to the destination range:
Range("E1").PasteSpecial Paste:=xlPasteValues
Note that you only need to specify the top-left cell of the paste destination range, in this case E1. Excel will automatically paste into the range E1:G10 since the copied data from A1:C10 occupies 10 rows and 3 columns.
Putting it all together, here’s the full VBA subroutine to copy and paste values without formatting:
Sub PasteValuesWithoutFormatting()
Range("A1:C10").Copy
Range("E1").PasteSpecial Paste:=xlPasteValues
End Sub
When you run this macro, it will copy the data from A1:C10, and then paste only the values into E1:G10, preserving any existing formatting in the destination range. The values will be pasted but the formatting from the source range will be left behind.
Handling Errors
When working with VBA macros, it’s important to anticipate and handle potential errors that could occur. One common issue is if the user tries to run the macro without anything copied to the clipboard. This will raise an error on the PasteSpecial line, since there’s nothing to paste.
To make your macro more robust, you can add error handling to check if there’s data on the clipboard before attempting to paste. Here’s an updated version of the subroutine that includes error handling:
Sub PasteValuesWithoutFormatting()
On Error Resume Next
Range("E1").PasteSpecial Paste:=xlPasteValues
If Err.Number <> 0 Then
MsgBox "Please copy data to the clipboard before running this macro."
End If
End Sub
Now if the user runs the macro without copying data first, they’ll see a friendly message box reminding them to copy before running the macro.
Keyboard Shortcut for Pasting Without Formatting
In addition to using VBA, there’s a handy keyboard shortcut in Excel to paste values without formatting:
- Select the cells you want to copy and press
Ctrl+C
to copy. - Navigate to the destination cells where you want to paste the values.
- Press
Alt+E+S+V
thenEnter
to paste only the values, without any formatting.
This can be a quick way to paste values in Excel without relying on VBA code. However, the VBA approach is still very useful when you need to automate the process or integrate pasting values into a larger macro.
Pasting Values & Other Pasting Options
The PasteSpecial method in VBA is quite versatile and lets you control various aspects of pasting beyond just values. Here are a few other common pasting options:
Paste Option | Description |
---|---|
xlPasteFormats | Pastes only the formatting from the copied cells |
xlPasteComments | Pastes only the comments from the copied cells |
xlPasteFormulas | Pastes formulas from the copied cells |
xlPasteValuesAndNumberFormats | Pastes values and number formatting |
To use any of these options, simply replace xlPasteValues
in the PasteSpecial line with the desired constant. For example:
Range("E1").PasteSpecial Paste:=xlPasteFormats
This would paste only the formatting from the copied cells to the destination range, leaving the actual data unchanged.
Clearing the Clipboard
After copying and pasting data, it’s good practice to clear the clipboard so you don’t accidentally paste the copied data elsewhere later. To clear the clipboard in VBA, simply use:
Application.CutCopyMode = False
You can add this line after the PasteSpecial line in your macro to clear the clipboard after pasting the values.
Paste Values to a Different Worksheet
So far, we’ve looked at pasting values within the same worksheet, but what if you want to paste values to a different worksheet in your workbook? VBA makes this easy too.
To paste to a different worksheet, you just need to qualify the destination range with the worksheet name. Here’s an example:
Sub PasteValuesToOtherSheet()
Range("A1:C10").Copy
Worksheets("Sheet2").Range("E1").PasteSpecial Paste:=xlPasteValues
End Sub
In this macro, we’re copying from the range A1:C10 on the active sheet, then pasting the values to cell E1 on a worksheet named “Sheet2”. Just replace “Sheet2” with the actual name of your destination worksheet.
You can also use the sheet’s code name in place of its display name. To find the code name, go to the VB Editor (Alt+F11), find your worksheet in the Project pane, and look at the (Name) property. Using the code name can be handy if your worksheet name contains spaces or special characters.
Final Thoughts
Pasting values without formatting is a common task in Excel, and VBA makes it easy to automate. By using the PasteSpecial method with xlPasteValues
, you can quickly transfer data from one part of a workbook to another while preserving the existing formatting in the destination range.
Remember, the PasteSpecial method isn’t just for values – you can use it to selectively paste formatting, formulas, comments, and more. By mastering this versatile tool, you open up a world of possibilities for manipulating and transferring data in Excel.
FAQs
What is the purpose of pasting values without formatting in Excel VBA?
How do you paste values without formatting using Excel VBA?
Range("E1").PasteSpecial Paste:=xlPasteValues
What other pasting options are available with the PasteSpecial method?
Is there a keyboard shortcut for pasting values without formatting in Excel?
How can I paste values to a different worksheet using Excel VBA?
Worksheets("Sheet2").Range("E1").PasteSpecial Paste:=xlPasteValues
. Replace “Sheet2” with the actual name of your destination worksheet.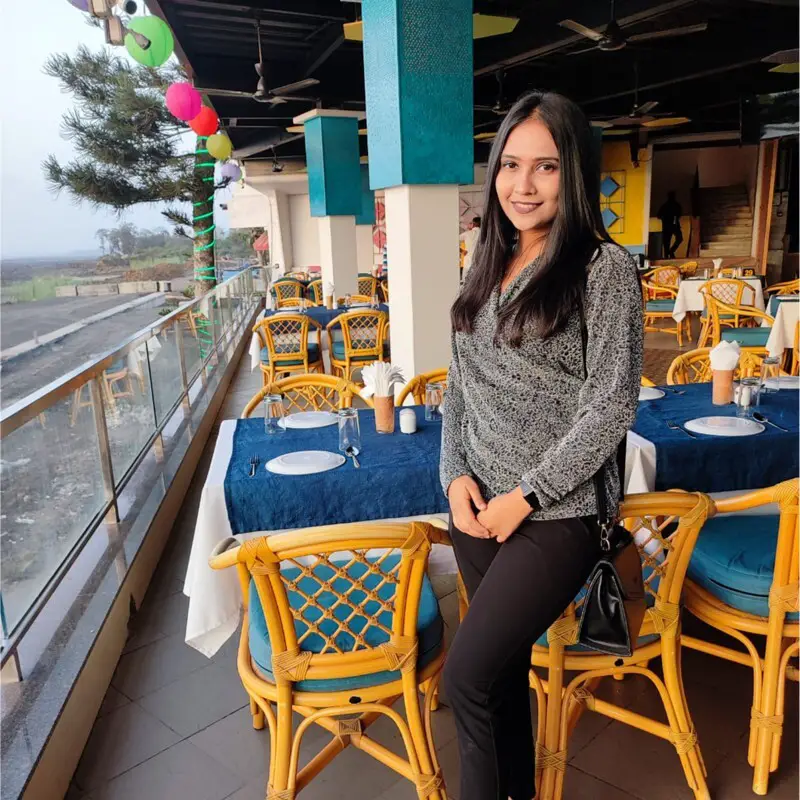
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.