Excel VBA: Paste Data to Another Sheet Without Activating
Do you want to know how to paste data to another sheet in Microsoft Excel using VBA without activating that sheet? You’ve come to the right place! In this article, we’ll provide a step-by-step guide on copying and pasting data between worksheets with VBA code, without the need to activate or select the destination sheet. Using this method can help streamline your VBA macros and make them more efficient. Let’s get started!
Understanding the Problem
When working with Excel VBA, you may often need to copy data from one worksheet and paste it into another. A common approach is to activate the destination sheet, paste the data, and then return to the original sheet. However, this method can be inefficient, especially if you are working with large datasets or need to perform this operation multiple times.
Fortunately, there is a way to paste data to another sheet without activating it, using VBA code. This approach is faster and more efficient, as it eliminates the need to switch between worksheets.
Why Activating Sheets Can Be Inefficient
Before diving into the VBA code solution, let’s understand why activating sheets can be inefficient:
- Time-consuming: Activating a sheet takes time, especially if you have a large workbook with many sheets. Each time you activate a sheet, Excel needs to redraw the sheet, which can slow down your VBA code.
- Screen flicker: When activating sheets, you may notice a visual flicker as Excel switches between sheets. This can be distracting and create an inconsistent user experience.
- Breaks the flow: If you are working on a specific sheet and your VBA code activates another sheet to paste data, it can break the user’s focus and workflow.
By pasting data without activating sheets, you can overcome these challenges and create more efficient and user-friendly VBA macros.
VBA Code to Paste Data Without Activating Destination Sheet
Here’s the VBA code to paste data from one worksheet to another without activating the destination sheet:
Sub PasteDataWithoutActivating()
Dim sourceSheet As Worksheet
Dim destinationSheet As Worksheet
Dim lastRow As Long
Dim lastColumn As Long
Set sourceSheet = ThisWorkbook.Sheets("Sheet1")
Set destinationSheet = ThisWorkbook.Sheets("Sheet2")
lastRow = sourceSheet.Cells(sourceSheet.Rows.Count, "A").End(xlUp).Row
lastColumn = sourceSheet.Cells(1, sourceSheet.Columns.Count).End(xlToLeft).Column
sourceSheet.Range(sourceSheet.Cells(1, 1), sourceSheet.Cells(lastRow, lastColumn)).Copy
destinationSheet.Range("A1").PasteSpecial xlPasteValues
Application.CutCopyMode = False
End Sub
Let’s break down the code and understand what each part does:
Step 1: Declare Variables
Dim sourceSheet As Worksheet
Dim destinationSheet As Worksheet
Dim lastRow As Long
Dim lastColumn As Long
We start by declaring the necessary variables:
sourceSheet
: Represents the worksheet containing the data to be copied.destinationSheet
: Represents the worksheet where the data will be pasted.lastRow
: Stores the last row number of the data in the source sheet.lastColumn
: Stores the last column number of the data in the source sheet.
Step 2: Set Worksheet References
Set sourceSheet = ThisWorkbook.Sheets("Sheet1")
Set destinationSheet = ThisWorkbook.Sheets("Sheet2")
Next, we set the references to the source and destination worksheets using the ThisWorkbook.Sheets
property. Replace "Sheet1"
and "Sheet2"
with the actual names of your worksheets.
Step 3: Find Last Row and Column
lastRow = sourceSheet.Cells(sourceSheet.Rows.Count, "A").End(xlUp).Row
lastColumn = sourceSheet.Cells(1, sourceSheet.Columns.Count).End(xlToLeft).Column
To determine the range of data to be copied, we find the last row and last column containing data in the source sheet. We use the Cells
property along with the End
and xlUp
/xlToLeft
methods to find the last occupied cell in column A and row 1, respectively.
Step 4: Copy Data from Source Sheet
sourceSheet.Range(sourceSheet.Cells(1, 1), sourceSheet.Cells(lastRow, lastColumn)).Copy
Using the Range
property and the Cells
references obtained in the previous step, we define the range of data to be copied from the source sheet. The Copy
method is then used to copy this range.
Step 5: Paste Data to Destination Sheet
destinationSheet.Range("A1").PasteSpecial xlPasteValues
To paste the copied data into the destination sheet, we use the PasteSpecial
method with the xlPasteValues
argument. This ensures that only the values are pasted, without any formatting or formulas.
In this example, the data is pasted starting from cell A1 in the destination sheet. You can modify the cell reference to paste the data at a different location if needed.
Step 6: Clear Clipboard
Application.CutCopyMode = False
Finally, we clear the clipboard using Application.CutCopyMode = False
to prevent any unexpected behavior in subsequent operations.
Benefits of Pasting Data Without Activating Sheets
Pasting data without activating the destination sheet offers several benefits:
- Improved Performance: By eliminating the need to activate the destination sheet, the VBA code runs faster, especially when working with large datasets or performing multiple copy-paste operations.
- Reduced Screen Flicker: When activating sheets, Excel may exhibit screen flicker, which can be visually distracting. Pasting data without activating sheets helps minimize this flicker.
- Enhanced User Experience: If you are creating a macro or an automated process, pasting data without activating sheets provides a smoother and more professional user experience, as the focus remains on the active sheet.
Tips and Best Practices
Here are some tips and best practices to keep in mind when using VBA to paste data without activating sheets:
- Always declare and set references to the source and destination sheets to avoid confusion and ensure the correct data is being copied and pasted.
- Use meaningful variable names to enhance code readability and maintainability.
- Be cautious when modifying the cell references for pasting data. Ensure that the destination range is large enough to accommodate the copied data and doesn’t overwrite existing data unintentionally.
- If you need to paste formatting or formulas along with the values, modify the
PasteSpecial
method argument accordingly (e.g.,xlPasteValuesAndNumberFormats
,xlPasteFormulas
). - Test your VBA code thoroughly to ensure it functions as expected and handles different scenarios gracefully.
Additional Considerations
When working with VBA to paste data without activating sheets, there are a few additional considerations to keep in mind:
- Workbook Structure: Ensure that the source and destination worksheets are in the same workbook. If you need to copy data between different workbooks, you’ll need to modify the code accordingly.
- Data Validation: Before copying and pasting data, it’s a good practice to validate the data in the source sheet to avoid pasting invalid or inconsistent data into the destination sheet.
- Error Handling: Implement error handling mechanisms in your VBA code to gracefully handle scenarios where the source or destination sheets are not found, or if there is insufficient space in the destination sheet to paste the data.
- Performance Optimization: If you are working with very large datasets, consider using array-based techniques to copy and paste data, as they can significantly improve performance compared to using the
Range
object.
Alternative Methods
While the VBA code provided in this article focuses on pasting data without activating sheets, there are alternative methods you can explore depending on your specific requirements:
- Using the
Worksheet.UsedRange
Property: If you want to copy the entire used range of a worksheet, you can use theUsedRange
property instead of finding the last row and column manually.
sourceSheet.UsedRange.Copy
destinationSheet.Range("A1").PasteSpecial xlPasteValues
- Copying Specific Ranges: If you need to copy a specific range of cells rather than the entire used range, you can directly specify the range address.
sourceSheet.Range("A1:D10").Copy
destinationSheet.Range("B5").PasteSpecial xlPasteValues
- Using the
Worksheet.Cells
Property: Instead of using theRange
object, you can directly access cells using theCells
property.
sourceSheet.Cells(1, 1).Copy
destinationSheet.Cells(5, 2).PasteSpecial xlPasteValues
Choose the method that best suits your needs and coding style.
Final Thoughts
Pasting data to another sheet in Excel VBA without activating the destination sheet is a valuable technique to optimize your VBA code and enhance efficiency. By using the VBA code provided in this article, you can seamlessly copy and paste data between worksheets without the need to switch between them.
Remember to declare variables, set worksheet references, find the last row and column of the data, copy the data from the source sheet, paste it into the destination sheet, and clear the clipboard afterward.
FAQs
What are the benefits of pasting data without activating sheets in Excel VBA?
Pasting data without activating sheets in Excel VBA offers several benefits, including improved performance, reduced screen flicker, and enhanced user experience. It eliminates the need to switch between worksheets, resulting in faster code execution and a smoother workflow.
How do I set the source and destination worksheet references in VBA?
To set the source and destination worksheet references in VBA, use the ThisWorkbook.Sheets("SheetName")
property. Replace "SheetName"
with the actual name of your worksheets. For example:
Set sourceSheet = ThisWorkbook.Sheets("Sheet1")
Set destinationSheet = ThisWorkbook.Sheets("Sheet2")
Can I paste formatting and formulas along with values using this method?
Yes, you can paste formatting and formulas along with values using this method. Modify the PasteSpecial
method argument accordingly. For example, use xlPasteValuesAndNumberFormats
to paste values and formatting, or xlPasteFormulas
to paste formulas.
How can I copy and paste data between different workbooks using VBA?
To copy and paste data between different workbooks using VBA, you’ll need to modify the code to reference the source and destination workbooks explicitly. Use the Workbooks("WorkbookName.xlsx")
property to specify the workbook names.
What should I do if I encounter an error while pasting data using this method?
If you encounter an error while pasting data using this method, first check if the source and destination worksheet references are correct. Ensure that the specified worksheets exist and are accessible. Additionally, implement error handling mechanisms in your VBA code to gracefully handle scenarios where the worksheets are not found or if there is insufficient space in the destination sheet to paste the data.
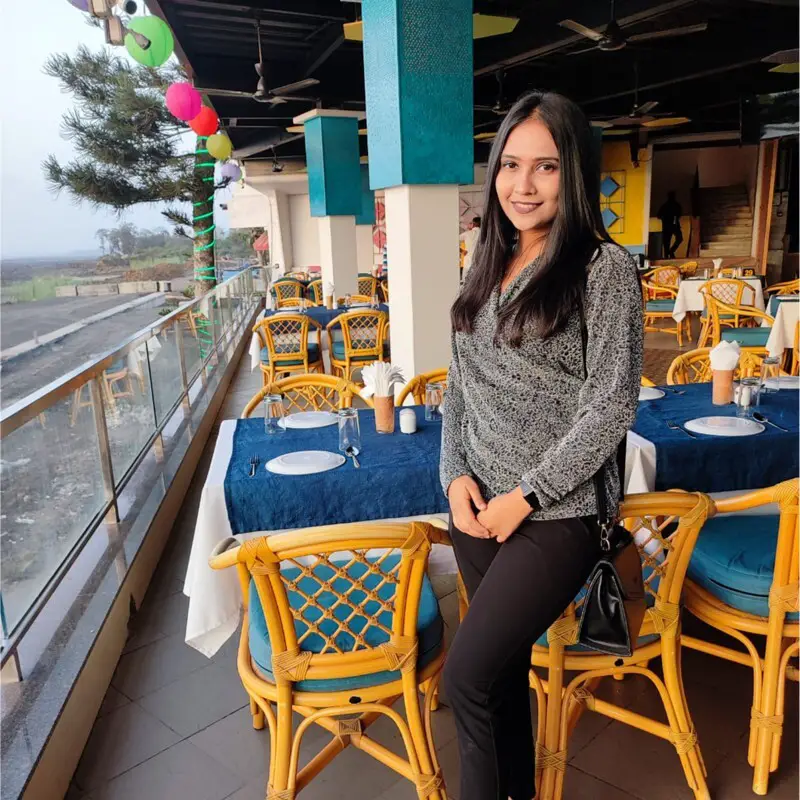
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.