How to Open a Read-Only Excel File Without a Prompt Using VBA
Have you ever tried to open a read-only Excel file using VBA, only to be interrupted by a prompt asking if you want to open the file as read-only? This can be frustrating, especially if you’re automating a process that needs to open many read-only files. Fortunately, there’s a way to open read-only Excel files without the prompt using VBA. In this article, we’ll show you how you can use VBA to open a read-only file in excel without facing those annoying prompts.
What Causes the Read-Only Prompt in Excel?
When you try to open a read-only Excel file, whether manually or using VBA, Excel displays a prompt asking if you want to open the file as read-only. This prompt appears because the file has been marked as read-only, either by the file system or by Excel itself.
There are a few reasons why a file might be marked as read-only:
- The file has been explicitly marked as read-only in the file system
- The file is being used by another process or user
- The file is located on a read-only network share or drive
- The file has been marked as read-only by Excel to protect its contents
Regardless of the reason, the read-only prompt can be a hindrance when you’re trying to automate a process using VBA.
Why Opening Read-Only Files Without a Prompt Matters
Opening read-only files without a prompt using VBA is important for several reasons:
- Automation: If you’re automating a process that involves opening many read-only files, the read-only prompt can interrupt the process and require manual intervention. By suppressing the prompt, you can ensure that your automated process runs smoothly.
- Efficiency: Manually clicking through read-only prompts can be time-consuming, especially if you need to open many files. By using VBA to open read-only files without a prompt, you can save time and work more efficiently.
- User Experience: If you’re building an application that opens read-only files, suppressing the read-only prompt can provide a better user experience. Users may not understand why they’re seeing a prompt and may find it confusing or annoying.
How to Open a Read-Only Excel File Without a Prompt Using VBA
To open a read-only Excel file without a prompt using VBA, you can use the Workbooks.Open
method with the ReadOnly
parameter set to True
. Here’s an example:
Sub OpenReadOnlyFile()
Dim wb As Workbook
Set wb = Workbooks.Open("C:\path\to\file.xlsx", ReadOnly:=True)
End Sub
In this example, the Workbooks.Open
method is used to open the file located at "C:\path\to\file.xlsx"
. The ReadOnly
parameter is set to True
, which tells Excel to open the file in read-only mode without displaying the prompt.
Handling Files with Passwords
If the read-only file is password-protected, you can use the Password
parameter of the Workbooks.Open
method to specify the password. Here’s an example:
Sub OpenPasswordProtectedReadOnlyFile()
Dim wb As Workbook
Set wb = Workbooks.Open("C:\path\to\file.xlsx", ReadOnly:=True, Password:="password")
End Sub
In this example, the Password
parameter is set to the password required to open the file.
Handling Files with Links to Other Workbooks
If the read-only file contains links to other workbooks, you may see additional prompts when opening the file using VBA. To suppress these prompts, you can use the UpdateLinks
parameter of the Workbooks.Open
method. Here’s an example:
Sub OpenReadOnlyFileWithLinks()
Dim wb As Workbook
Set wb = Workbooks.Open("C:\path\to\file.xlsx", ReadOnly:=True, UpdateLinks:=False)
End Sub
In this example, the UpdateLinks
parameter is set to False
, which tells Excel not to update any links in the file when opening it.
Handling Files with Macros
If the read-only file contains macros, you may see a security warning when opening the file using VBA. To suppress this warning, you can use the AutomationSecurity
property of the Application
object. Here’s an example:
Sub OpenReadOnlyFileWithMacros()
Application.AutomationSecurity = msoAutomationSecurityLow
Dim wb As Workbook
Set wb = Workbooks.Open("C:\path\to\file.xlsx", ReadOnly:=True)
End Sub
In this example, the AutomationSecurity
property is set to msoAutomationSecurityLow
, which allows macros to run without displaying a security warning. Note that this is not recommended for untrusted files, as it can be a security risk.
Best Practices for Opening Read-Only Excel Files Using VBA
When opening read-only Excel files using VBA, there are a few best practices to keep in mind:
Use Error Handling
It’s always a good idea to use error handling when working with files in VBA. This can help prevent crashes and other issues if something goes wrong while opening the file. Here’s an example of how to use error handling when opening a read-only file:
Sub OpenReadOnlyFileWithErrorHandling()
On Error Resume Next
Dim wb As Workbook
Set wb = Workbooks.Open("C:\path\to\file.xlsx", ReadOnly:=True)
If Err.Number <> 0 Then
' Handle the error
MsgBox "Error opening file: " & Err.Description
End If
On Error GoTo 0
End Sub
In this example, the On Error Resume Next
statement is used to continue execution even if an error occurs. The Err
object is then checked to see if an error occurred while opening the file. If an error did occur, a message box is displayed with the error description.
Close the File When You’re Done
When you’re finished working with a read-only file that you’ve opened using VBA, it’s important to close the file to free up system resources. You can use the Close
method of the Workbook
object to close the file. Here’s an example:
Sub OpenAndCloseReadOnlyFile()
Dim wb As Workbook
Set wb = Workbooks.Open("C:\path\to\file.xlsx", ReadOnly:=True)
' Do something with the file
wb.Close SaveChanges:=False
End Sub
In this example, the Close
method is called on the Workbook
object represented by the wb
variable. The SaveChanges
parameter is set to False
to indicate that any changes made to the file should not be saved.
Use Absolute File Paths
When specifying the path to the read-only file you want to open, it’s best to use an absolute file path rather than a relative path. This can help ensure that your code works correctly regardless of the current working directory. Here’s an example:
Sub OpenReadOnlyFileWithAbsolutePath()
Dim wb As Workbook
Set wb = Workbooks.Open("C:\path\to\file.xlsx", ReadOnly:=True)
End Sub
In this example, the absolute file path "C:\path\to\file.xlsx"
is used to specify the location of the read-only file.
Use Conditional Statements
In some cases, you may want to perform different actions based on whether the file is read-only or not. You can use conditional statements to check the ReadOnly
property of the Workbook
object and take appropriate action. Here’s an example:
Sub CheckReadOnlyStatus()
Dim wb As Workbook
Set wb = Workbooks.Open("C:\path\to\file.xlsx", ReadOnly:=True)
If wb.ReadOnly Then
' File is read-only, do something here
MsgBox "File is read-only."
Else
' File is not read-only, do something else here
MsgBox "File is not read-only."
End If
wb.Close SaveChanges:=False
End Sub
In this example, the ReadOnly
property of the Workbook
object is checked using an If
statement. If the file is read-only, a message box is displayed indicating that the file is read-only. If the file is not read-only, a different message box is displayed.
Troubleshooting Common Issues
If you’re having trouble opening a read-only Excel file using VBA, here are a few things to check:
Make Sure the File Path is Correct
Double-check that the file path you’re using in your VBA code is correct. If the file path is incorrect, Excel won’t be able to find the file and will display an error.
Check the File Permissions
Make sure that you have permission to open the read-only file. If you don’t have permission, Excel will display an error when you try to open the file using VBA.
Check for Other Open Instances of Excel
If you have other instances of Excel open, they may be holding a lock on the read-only file you’re trying to open. Close any other instances of Excel and try again.
Check for Network Issues
If the read-only file is located on a network drive or share, make sure that the network connection is stable and that you have permission to access the file. Network issues can cause errors when trying to open files using VBA.
Final Thoughts
Opening a read-only Excel file without a prompt using VBA can save you time and frustration when automating processes that involve read-only files. By using the Workbooks.Open
method with the ReadOnly
parameter set to True
, you can open read-only files silently, without interrupting your code with prompts.
Just remember to use error handling, close the file when you’re done, use absolute file paths, and troubleshoot any issues that may arise. With these best practices in mind, you’ll be able to work with read-only Excel files using VBA with ease.
FAQs
What is a read-only Excel file?
A read-only Excel file is a spreadsheet that has been marked as read-only, either by the file system or by Excel itself. When a file is marked as read-only, it can be opened and viewed, but not edited or saved.
Why does Excel display a prompt when opening a read-only file?
Excel displays a prompt when opening a read-only file to inform the user that the file cannot be edited and to ask if they want to open the file as read-only. This prompt can be disruptive when automating processes using VBA.
How can I open a read-only Excel file without a prompt using VBA?
To open a read-only Excel file without a prompt using VBA, you can use the Workbooks.Open
method with the ReadOnly
parameter set to True
. This tells Excel to open the file in read-only mode without displaying the prompt.
What if the read-only file is password-protected?
If the read-only file is password-protected, you can use the Password
parameter of the Workbooks.Open
method to specify the password. This allows you to open password-protected read-only files using VBA.
What are some best practices for opening read-only Excel files using VBA?
Some best practices for opening read-only Excel files using VBA include using error handling to catch and handle any errors that may occur, closing the file when you’re finished working with it to free up system resources, using absolute file paths to ensure your code works correctly, and using conditional statements to perform different actions based on whether the file is read-only or not.
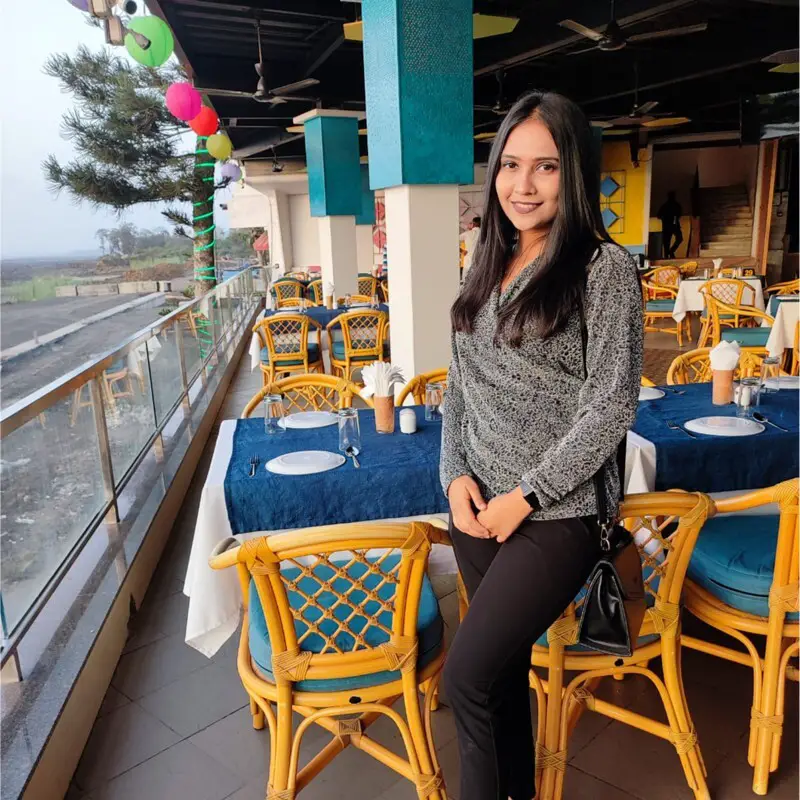
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.