Excel VBA: Refreshing Data Connections by Name
In today’s data-driven business environment, keeping information up-to-date is crucial for making informed decisions. Microsoft Excel, with its powerful data analysis capabilities, is a go-to tool for many professionals. One of its key features is the ability to connect to external data sources, allowing users to work with real-time information. However, manually refreshing these connections can be time-consuming and prone to errors. This is where Visual Basic for Applications (VBA) comes in, offering a solution to automate the process of refreshing data connections by name.
Understanding Data Connections in Excel
What are Data Connections?
Data connections in Excel are links between your workbook and external data sources. These sources can include databases, text files, web services, or other spreadsheets. By establishing these connections, Excel can retrieve and display up-to-date information without manual data entry.
Types of Data Connections
Excel supports various types of data connections, including:
- ODBC (Open Database Connectivity): For connecting to database management systems
- OLEDB (Object Linking and Embedding Database): A more advanced method for database connections
- Web Queries: For retrieving data from web pages
- Text Files: Connections to CSV or other delimited text files
- Excel Workbooks: Links to data in other Excel files
Importance of Refreshing Data Connections
Refreshing data connections ensures that your Excel workbook contains the most current information from the external source. This is particularly important for:
- Financial reporting
- Inventory management
- Sales tracking
- Performance analytics
Regular updates to these connections maintain the accuracy and relevance of your data analysis and decision-making processes.
VBA Basics for Data Connection Refresh
Key VBA Concepts for Data Refresh
To effectively use VBA for refreshing data connections, you should be familiar with:
- Workbook and Worksheet Objects: Represent the Excel file and individual sheets
- Connections Collection: Contains all data connections in a workbook
- Connection Object: Represents a single data connection
- Refresh Method: Used to update the data from the external source
Setting Up the VBA Environment
To start using VBA for data connection refresh:
- Open Excel and press Alt + F11 to open the Visual Basic Editor
- Insert a new module by clicking Insert > Module
- Write your VBA code in the module window
Refreshing Data Connections by Name Using VBA
Identifying Connection Names
Before refreshing a connection, you need to know its name. To find connection names:
- Go to the Data tab in Excel
- Click on Queries & Connections
- The names of your connections will be listed in the pane that appears
Basic VBA Code to Refresh a Single Connection
Here’s a simple VBA function to refresh a connection by name:
Sub RefreshConnectionByName(connectionName As String)
ThisWorkbook.Connections(connectionName).Refresh
End Sub
To use this function, you would call it like this:
RefreshConnectionByName "MyConnectionName"
Handling Errors in Connection Refresh
It’s important to include error handling in your VBA code to manage situations where a connection might not exist or fail to refresh:
Sub RefreshConnectionByNameWithErrorHandling(connectionName As String)
On Error Resume Next
ThisWorkbook.Connections(connectionName).Refresh
If Err.Number <> 0 Then
MsgBox "Error refreshing connection: " & connectionName & vbNewLine & Err.Description
End If
On Error GoTo 0
End Sub
Refreshing Multiple Connections
To refresh multiple connections, you can create a loop that iterates through an array of connection names:
Sub RefreshMultipleConnections()
Dim connectionNames As Variant
Dim i As Integer
connectionNames = Array("Connection1", "Connection2", "Connection3")
For i = LBound(connectionNames) To UBound(connectionNames)
RefreshConnectionByNameWithErrorHandling connectionNames(i)
Next i
End Sub
How to Find out the Names of Your Data Connections in Excel?
To find out the names of your data connections in Excel, you can follow these steps:
- Open your Excel workbook that contains the data connections.
- Go to the Data tab on the Excel ribbon.
- In the Queries & Connections group, click on Queries & Connections.
This will open the Queries & Connections pane, which displays all the connections available in your workbook. The names of your connections will be listed here.
Alternatively, for older versions of Excel or if you prefer a different method:
- Go to the Data tab.
- Click on Connections in the Connections section.
- This will open the Workbook Connections dialog box, where you can see a list of all connections in your workbook.
If you’re using VBA to manage your connections, you can use the following code to list all connection names in your workbook:
Sub ListConnectionNames()
Dim conn As WorkbookConnection
For Each conn In ThisWorkbook.Connections
Debug.Print conn.Name
Next conn
End Sub
This code will print the names of all connections to the Immediate window in the VBA editor.
Remember, connection names are typically descriptive and often relate to the data source or the purpose of the connection. They might be named after the source file, database, or the type of data they’re retrieving.
Advanced Techniques for Data Connection Refresh
Scheduling Automatic Refreshes
You can use the Windows Task Scheduler in combination with VBA to automate regular refreshes of your data connections:
- Create a VBA macro that refreshes your connections
- Save your Excel file as a macro-enabled workbook (.xlsm)
- Create a batch file that opens Excel and runs your macro
- Set up a scheduled task in Windows Task Scheduler to run the batch file at specified intervals
Selective Refresh Based on Conditions
Sometimes, you may want to refresh connections only under certain conditions. Here’s an example that refreshes a connection if a cell value meets a specific criterion:
Sub RefreshConnectionIfNeeded()
If Range("A1").Value > 100 Then
RefreshConnectionByName "SalesData"
End If
End Sub
Logging Refresh Activities
Keeping a log of refresh activities can be useful for troubleshooting and auditing. Here’s a simple logging function:
Sub LogRefreshActivity(connectionName As String, success As Boolean)
Dim logSheet As Worksheet
Set logSheet = ThisWorkbook.Sheets("RefreshLog")
logSheet.Cells(logSheet.Rows.Count, 1).End(xlUp).Offset(1, 0).Value = Now()
logSheet.Cells(logSheet.Rows.Count, 2).End(xlUp).Offset(1, 0).Value = connectionName
logSheet.Cells(logSheet.Rows.Count, 3).End(xlUp).Offset(1, 0).Value = IIf(success, "Success", "Failure")
End Sub
Best Practices for VBA Data Connection Refresh
Optimizing Performance
When working with multiple or large data connections, consider these performance optimization techniques:
- Refresh only necessary connections: Avoid refreshing all connections if only specific ones need updating
- Use background refresh: Set
BackgroundQuery = True
for connections to allow Excel to remain responsive during updates - Implement error handling: Proper error management prevents your code from hanging on failed refreshes
Security Considerations
When working with VBA and external data connections, keep these security points in mind:
- Macro security: Ensure that macro security settings in Excel allow your VBA code to run
- Data source authentication: Store credentials securely and avoid hardcoding them in VBA scripts
- File permissions: Ensure that users have the necessary permissions to access both the Excel file and the external data sources
Maintaining Code Readability and Modularity
To keep your VBA code manageable:
- Use descriptive variable and function names
- Comment your code to explain complex logic
- Break down large procedures into smaller, reusable functions
- Use constants for values that might change, such as connection names
Troubleshooting Common Issues
Connection String Errors
If you encounter connection string errors:
- Double-check the connection string in the Excel connection properties
- Ensure all required parameters are included and correctly formatted
- Verify that the data source is accessible from your network
Refresh Timeout Issues
For timeout issues during refresh:
- Increase the query timeout setting in the connection properties
- Optimize the underlying query if possible to reduce execution time
- Consider implementing a retry mechanism in your VBA code
Permissions and Authentication Problems
When facing permissions or authentication issues:
- Verify that the user running the Excel file has the necessary permissions for the data source
- Check if any passwords or credentials need updating
- Ensure that any required VPN connections are active if accessing remote data sources
Final Thoughts
Mastering the art of refreshing data connections by name using Excel VBA can significantly enhance your data management and analysis workflows. By automating these processes, you can ensure that your Excel workbooks always contain the most up-to-date information, leading to more accurate insights and better-informed decisions.
Whether you’re a financial analyst keeping tabs on market data, a sales manager tracking real-time performance, or a data scientist preparing datasets for analysis, the ability to programmatically refresh data connections in Excel is a valuable skill that will serve you well in today’s data-driven business landscape.
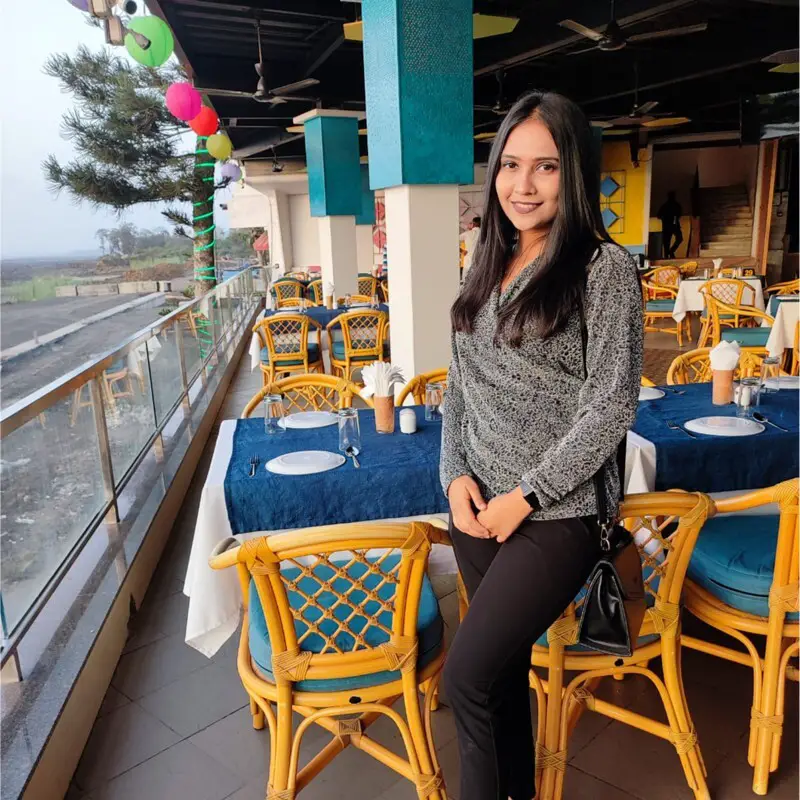
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.