Excel VBA Crashes Without Error? (Here’s the Fix)
Excel VBA (Visual Basic for Applications) is a powerful programming tool that extends Excel’s functionality, allowing users to automate repetitive tasks and create custom solutions. However, one of the most frustrating issues users encounter is when Excel VBA crashes without displaying any error message. These silent crashes leave users with no clear indication of what went wrong or how to fix the problem.
In this article, we will examine the common causes of silent VBA crashes and provide systematic troubleshooting steps to diagnose and resolve these issues.
Common Causes of Excel VBA Crashing Without Error
1. Corrupted VBA Project Components
One of the most frequent causes of silent VBA crashes is corrupted code modules within your VBA project. This corruption can occur due to:
- Improper Excel shutdown during code execution
- Power outages while saving macros
- Issues with the VBA project storage format
- Conflicts when multiple users access the same workbook
When a VBA project becomes corrupted, Excel may crash rather than displaying a meaningful error message, as it cannot properly interpret the damaged code.
2. Problematic Add-ins
Excel add-ins (both COM add-ins and Excel add-ins) can introduce compatibility issues that lead to crashes, particularly when:
- Multiple add-ins conflict with each other
- An add-in was designed for a different Excel version
- The add-in contains faulty code
- The add-in lacks proper error handling
These add-ins run in the background and can interfere with VBA execution, causing Excel to crash without warning.
3. Automation Timing Issues
Automation errors frequently occur when VBA functions try to execute during Excel’s startup process before the application is fully initialized. This timing mismatch can cause Excel to crash without displaying errors because:
- Macro security settings haven’t fully loaded
- Required references aren’t yet available
- The Excel object model isn’t completely initialized
4. Insufficient Error Handling
Poor error handling practices in VBA code can lead to silent crashes when:
- Using “On Error Resume Next” without proper error checking
- Missing error handlers in critical sections of code
- Failing to reset error handlers after use
- Not implementing structured error handling throughout the project
5. Known Excel Bugs
Microsoft Excel has several known issues related to VBA compilation and execution that can cause crashes without error messages. These bugs vary by Excel version and may include:
- Problems with specific VBA functions or methods
- Memory management issues in the VBA runtime
- Issues with the VBA compiler
How to Fix Excel VBA Crashing Without Error
1. Isolate the Problem Source
Begin your troubleshooting by determining whether the issue is with Excel itself, an add-in, or your specific VBA code:
Open Excel in Safe Mode
- Close all Excel instances completely
- Press Windows key + R to open the Run dialog
- Type
excel.exe /safe
and press Enter - Test if the problem persists in safe mode (add-ins and automation are disabled)
If Excel runs without crashing in safe mode, the issue likely involves an add-in or custom VBA code.
2. Disable and Test Add-ins
Add-ins are a common source of Excel VBA crashes. Follow these steps to identify problematic add-ins:
- Open Excel normally
- Navigate to File > Options > Add-ins
- Look at the list of active add-ins
- Select “COM Add-ins” from the dropdown and click “Go”
- Uncheck all add-ins and click OK
- Restart Excel and test if the problem persists
- If Excel no longer crashes, re-enable add-ins one by one, restarting Excel each time, until you identify the problematic add-in
Add-in Type | Location | Common Issues |
---|---|---|
COM Add-ins | File > Options > Add-ins > Manage: COM Add-ins | Compatibility conflicts, memory leaks |
Excel Add-ins | File > Options > Add-ins > Manage: Excel Add-ins | Corrupted files, code errors |
Automation Add-ins | File > Options > Add-ins > Manage: Automation Add-ins | Timing issues, missing dependencies |
3. VBA Code Inspection and Repair
If the issue appears to be with your VBA code, try these approaches:
Compile and Check for Errors
- Open the VBA Editor (Alt + F11)
- Go to Debug > Compile VBAProject
- Fix any syntax errors that are identified
- Check for logical errors in your code
Reset the VBA Environment
- Export all VBA modules to backup files
- Delete all modules from the workbook
- Save, close, and reopen the workbook
- Import the modules one by one, testing after each import
Implement Robust Error Handling
- Add proper error handling to all procedures:
Sub ExampleProcedure()
On Error GoTo ErrorHandler
' Your code here
Exit Sub
ErrorHandler:
MsgBox "Error " & Err.Number & ": " & Err.Description
End Sub
- Avoid using
On Error Resume Next
except for specific operations that might fail safely
4. Registry Modifications
For persistent issues related to VBA compilation, registry modifications may help:
- Close all Excel instances
- Open Registry Editor (Win+R, type regedit, press Enter)
- Navigate to:
HKEY_CURRENT_USER\Software\Microsoft\Office\16.0\Excel\Options
- Note: Use the appropriate version number for your Excel (16.0 = Excel 2016/365)
- Right-click the Options folder, select New > DWORD Value
- Name it
ForceVBALoadFromSource
- Set its value to 1
- Restart Excel
This forces Excel to recompile VBA code on load rather than using cached compiled code.
5. Application Repair and Updates
If the above steps don’t resolve the issue, try these system-level solutions:
Update Microsoft Office
- Open any Office application
- Go to File > Account > Update Options > Update Now
- Install all available updates
- Restart your computer
Repair Office Installation
- Open Control Panel > Programs > Programs and Features
- Find Microsoft Office in the list
- Right-click and select “Change”
- Choose “Repair” and follow the instructions
- Restart your computer after repair completes
Advanced Techniques to Fix Excel VBA Crash Without Error
1) Memory Inspection
VBA crashes often occur due to memory management issues. Use these techniques to identify memory problems:
- Add debug code to track variable values:
Debug.Print "Variable X value: " & X
- Check for proper object cleanup:
Dim ws As Worksheet
Set ws = Sheets("Sheet1")
' Code using ws
Set ws = Nothing ' Proper cleanup
2) Break on All Errors
Configure the VBA Editor to catch errors that might be occurring silently:
- Open the VBA Editor (Alt + F11)
- Go to Tools > Options > General
- Check “Break on All Errors”
- Run your code to see if any errors are caught
Use the VBA debugger to execute code line by line:
- Set a breakpoint at the beginning of your code (click in the margin)
- Press F8 to step through the code one line at a time
- Monitor the execution flow and variable values
- Look for the exact point where Excel crashes
Preventing Future VBA Crashes
1) Code Organization Best Practices
- Use modular programming techniques to isolate functionality
- Implement comprehensive error handling in all procedures
- Document code with clear comments explaining complex operations
- Test code thoroughly before deploying to production environments
2) Memory Management
- Explicitly declare all variables with proper data types
- Release object references when they’re no longer needed
- Avoid creating circular references between objects
- Use the VBA Collection object instead of arrays for dynamic data
3) Project Structure
- Organize code into logical modules by functionality
- Create a dedicated error handling module with standardized routines
- Implement a logging system to track operations and potential issues
- Use version control to track changes and enable rollbacks
Final Thoughts
Excel VBA crashes without error messages can be frustrating, but a systematic troubleshooting approach can help identify and resolve the underlying issues.
Remember that prevention is better than cure—implementing proper error handling, following code organization best practices, and regularly updating Excel will help reduce the likelihood of encountering these silent crashes in the future.
Frequently Asked Questions
Why does my Excel VBA code crash without showing any error message?
Silent VBA crashes typically occur due to corrupted VBA components, problematic add-ins, automation timing issues, insufficient error handling, or known Excel bugs. Without proper error handling, Excel often terminates execution instead of displaying meaningful error messages.
How do I determine if an add-in is causing my Excel VBA to crash?
Launch Excel in safe mode (excel.exe /safe). If it works properly, systematically disable all add-ins via File > Options > Add-ins, then re-enable them one by one while testing after each addition to identify the problematic add-in.
What registry changes can help fix Excel VBA crashes?
Add a DWORD value named ‘ForceVBALoadFromSource’ with value 1 in HKEY_CURRENT_USER\Software\Microsoft\Office\16.0\Excel\Options (adjust version number accordingly). This forces Excel to recompile VBA code on load rather than using potentially corrupted cached code.
How can I improve error handling in my VBA code to prevent crashes?
Implement structured error handlers using ‘On Error GoTo ErrorHandler’ instead of ‘On Error Resume Next’. Include exit points before error handlers and enable ‘Break on All Errors’ in the VBA Editor during development to catch silent errors.
What should I do if my Excel VBA project becomes corrupted?
Export all modules as backups, delete them from the workbook, save and close the file, then reopen it. Import modules one by one, testing after each import. This process rebuilds the VBA project structure and typically resolves corruption issues.
How can I prevent Excel VBA crashes in the future?
Implement comprehensive error handling, properly declare variables, release object references when finished, organize code logically, document thoroughly, implement logging, use version control, and keep Excel updated with the latest patches.
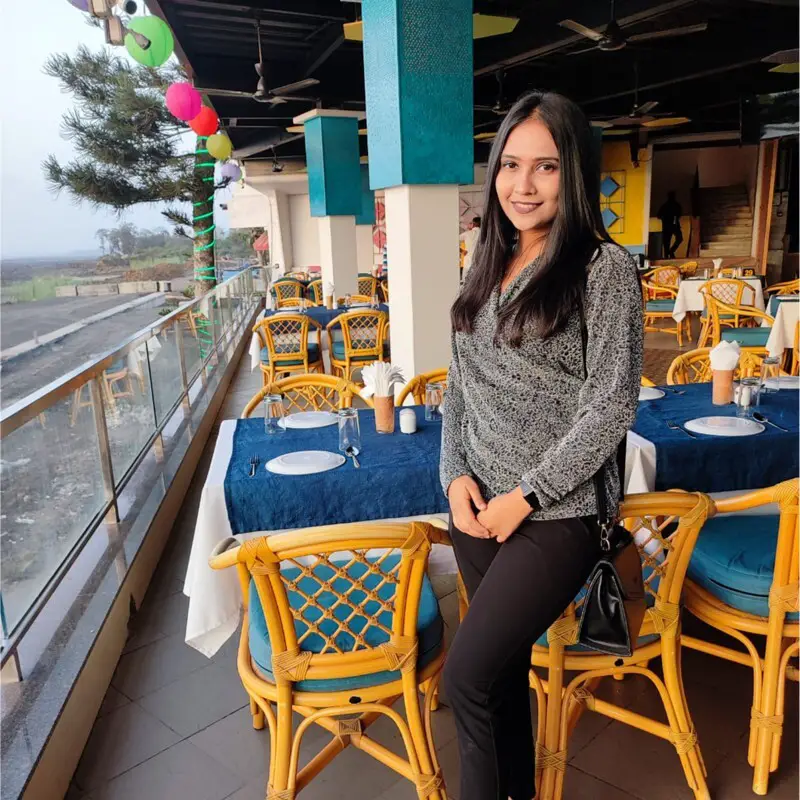
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.