Open Workbooks Without Activating Them in Excel VBA
Did you know that you can perform operations on workbooks in Excel VBA without activating them?
When working with VBA macros, it’s common to need to open a workbook in the background without disrupting your workflow or causing the screen to flash. Activating a workbook can be problematic, even if the ScreenUpdating property is set to False.
In this article, we will explore various solutions to open workbooks without activation, allowing you to perform operations seamlessly. Whether you’re using the Workbooks. Open method, manipulating the Visible property, opening workbooks as add-ins, using workbook variables, or leveraging event-driven programming, we have you covered.
Let’s dive into the details and learn how to open workbooks without activating them in Excel VBA.
Using the Workbooks.Open method
You can use the Workbooks.Open method in VBA to open a workbook without activating it. This method allows you to access and manipulate the workbook’s data and structure while keeping your original workbook active.
To use the Workbooks.Open method, you need to specify the file path and name of the workbook you want to open. You can also provide additional parameters, such as password protection, read-only mode, or specific file formats.
Here is an example of VBA code that demonstrates how to open a workbook without activation using the Workbooks.Open method:
Dim wb As Workbook
Set wb = Workbooks.Open("C:\Documents\Workbook.xlsx", False, True)
In this example, the Workbook.xlsx file located at C:\Documents is opened without activating it. The False parameter specifies that the workbook should not be updated from the source file, while the True parameter indicates that the workbook should be opened in read-only mode.
By assigning the opened workbook to the wb variable, you can refer to it in your VBA code without activating it or changing the active workbook. You can perform various operations on the workbook, such as reading data, modifying cells, or executing macros, all while keeping your original workbook active in the background.
Setting the Visible property to False
Another way to open a workbook in the background without activation is by setting the Visible property of the workbook to False. This hides the workbook from the user’s view, allowing you to perform operations on it without activating it.
VBA Code |
---|
|
Opening the workbook as an add-in
If you’re working with an Excel add-in and need to access its functionality without activating or displaying it, you can open it as an add-in instead of a regular workbook. This allows you to seamlessly use the add-in’s features while keeping your workflow uninterrupted. In Excel VBA, you can accomplish this by using the following code:
Workbooks.Open Filename:="C:\Path\To\Your\Add-In.xlam", UpdateLinks:=0
In this code, “C:\Path\To\Your\Add-In.xlam” should be replaced with the actual file path and name of your add-in. The UpdateLinks argument is set to 0 to disable updating any links in the add-in.
By opening the workbook as an add-in, you can leverage its functionality through VBA code without activating it in Excel. This method is especially useful when you want to integrate the add-in’s features seamlessly into your VBA project without interrupting the user’s experience in Excel.
Opening an add-in as a workbook allows you to utilize the powerful capabilities of the add-in while keeping it hidden from the user’s view. This ensures a smooth and efficient workflow, enhancing your productivity in Excel VBA development.
Using workbook variables
In Excel VBA, you can reference a workbook without activating it by using workbook variables. By assigning the opened workbook to a variable, you can easily refer to it in your code without changing the active workbook or disrupting your workflow. This is a useful technique when you need to perform operations on a workbook without activating it.
Here is an example of VBA code that opens a workbook and references it using a workbook variable:
Dim wb As Workbook
Set wb = Workbooks.Open("C:\Path\To\Workbook.xlsx")
' Perform operations on the workbook
wb.Sheets("Sheet1").Range("A1").Value = "Hello, world!"
By declaring a variable wb
as a Workbook
object and using the Workbooks.Open
method, you can open the workbook without activating it. The opened workbook is then assigned to the wb
variable, allowing you to reference it in subsequent code lines.
With the workbook variable wb
, you can access its sheets, ranges, and other properties to interact with the workbook’s data and structure. For example, the code line wb.Sheets("Sheet1").Range("A1").Value = "Hello, world!"
sets the value of cell A1 in Sheet1 of the opened workbook to “Hello, world!” without activating the workbook.
This technique of using workbook variables provides a convenient way to reference and manipulate workbooks without the need to activate them, resulting in more efficient and streamlined VBA code.
Avoiding workbook activation with event-driven programming
When working with Excel VBA macros, you may often encounter the need to perform operations on a workbook without activating it. This can help maintain a smooth workflow and prevent disruptions to the user interface. One effective approach to achieve this is through event-driven programming.
Event-driven programming allows you to handle workbook events and execute code based on specific triggers, such as changes in cells or the opening and closing of workbooks. By leveraging workbook events, you can perform operations on the workbook without explicitly activating it.
For example, you can write VBA code that automatically executes when a workbook is opened or closed, without activating it. This allows you to keep your original workbook active while still reacting to events in the opened workbook. Here’s an example of VBA code that demonstrates event-driven programming:
Private Sub Workbook_Open()
' Code to be executed when the workbook is opened
' Perform operations on the workbook without activating it
End Sub
Private Sub Workbook_BeforeClose(Cancel As Boolean)
' Code to be executed before the workbook is closed
' Perform operations on the workbook without activating it
End Sub
By utilizing event-driven programming, you can effectively avoid workbook activation and perform operations on workbooks seamlessly. This approach helps maintain a smooth user experience while ensuring the desired functionality is achieved.
In the next section, we will discuss some best practices for opening workbooks without activating them in Excel VBA.
Best practices for opening workbooks without activating
When working with Excel VBA, opening workbooks without activating them can help improve the efficiency and performance of your code. Follow these best practices to ensure your VBA code for opening workbooks is as efficient as possible.
1. Utilize the Workbooks.Open method: The Workbooks.Open method is a powerful tool in Excel VBA that allows you to open workbooks without activating them. This method allows you to perform operations on the workbook in the background without disrupting the user’s experience.
2. Hide the workbook using the Visible property: Setting the Visible property of the workbook to False allows you to open the workbook in the background without displaying it to the user. This is useful when you need to interact with the workbook without interrupting the user’s workflow.
3. Make use of workbook variables: By assigning the opened workbook to a variable, you can reference and manipulate it without activating it or changing the active workbook. This helps maintain the flow of your code and improves its efficiency.
By following these best practices and utilizing efficient VBA code, such as the Workbooks.Open method and the Visible property, you can open workbooks without activating them and enhance the performance of your Excel VBA macros.
FAQ
How can I open a workbook in Excel VBA without activating it?
You can open a workbook without activating it by using the Workbooks.Open method in VBA. Assign the opened workbook to a variable to perform operations on it while keeping your original workbook active.
Can I open a workbook in the background without activating it?
Yes, you can open a workbook in the background without activating it by setting the Visible property of the workbook to False. This hides the workbook from the user’s view, allowing you to perform operations on it without activating it.
How can I open an Excel add-in as a workbook without activation?
To open an Excel add-in without activation, you can open it as an add-in instead of a regular workbook. This allows you to use the add-in’s functionality without activating it or displaying it to the user.
Is it possible to reference a workbook without activating it in VBA?
Yes, you can reference a workbook without activating it by using workbook variables in your VBA code. Assign the opened workbook to a variable to refer to it without activating it or changing the active workbook.
How can I avoid workbook activation using event-driven programming?
You can avoid workbook activation by using event-driven programming. By handling workbook events, you can perform operations on the workbook without activating it explicitly, allowing you to keep your original workbook active.
What are some best practices for opening workbooks without activating them in Excel VBA?
To open workbooks without activation in Excel VBA, it is important to follow some best practices for efficient and effective code. Some tips include using the Workbooks.Open method, setting the Visible property to False, and following event-driven programming techniques.
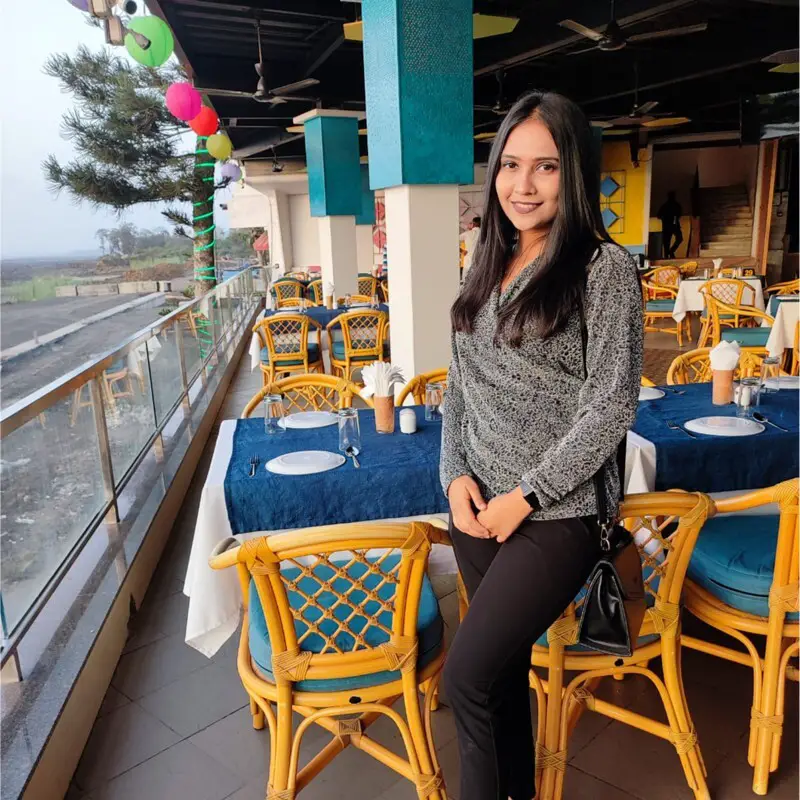
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.