Get Sheet Names Without Opening Workbooks in Excel Using VBA
Are you looking for a way to retrieve the sheet name from an Excel workbook without actually opening it? This can be a handy trick when you need to work with multiple workbooks or automate certain tasks in Excel. In this article, we will explore how you can use VBA (Visual Basic for Applications) to get the sheet name from an Excel workbook without opening it.
Understanding the VBA Code
To achieve this, we will use a simple VBA code that utilizes the Workbooks.Open
method with the ReadOnly
parameter set to True
. Here’s the code:
Sub GetSheetName()
Dim wbPath As String
Dim sheetName As String
wbPath = "C:\Path\To\Your\Workbook.xlsx"
Application.ScreenUpdating = False
With Workbooks.Open(wbPath, ReadOnly:=True)
sheetName = .Sheets(1).Name
.Close False
End With
Application.ScreenUpdating = True
MsgBox "The sheet name is: " & sheetName
End Sub
Let’s break down the code and understand each part:
Declaring Variables
Dim wbPath As String
Dim sheetName As String
We start by declaring two variables:
wbPath
: Stores the file path of the Excel workbook you want to retrieve the sheet name from.sheetName
: Stores the name of the sheet that will be retrieved.
Specifying the Workbook Path
wbPath = "C:\Path\To\Your\Workbook.xlsx"
Replace "C:\Path\To\Your\Workbook.xlsx"
with the actual file path of your Excel workbook.
Disabling Screen Updating
Application.ScreenUpdating = False
This line disables screen updating, which helps improve the performance of the code by preventing Excel from visually updating the screen during execution.
Opening the Workbook in Read-Only Mode
With Workbooks.Open(wbPath, ReadOnly:=True)
' Code to retrieve sheet name
End With
We use the Workbooks.Open
method to open the workbook specified by wbPath
. The ReadOnly
parameter is set to True
, which opens the workbook in read-only mode, preventing any changes from being made to the workbook.
Retrieving the Sheet Name
sheetName = .Sheets(1).Name
Inside the With
block, we retrieve the name of the first sheet in the workbook using .Sheets(1).Name
and assign it to the sheetName
variable. You can change the sheet index (1
in this case) to retrieve the name of a different sheet if needed.
Closing the Workbook
.Close False
After retrieving the sheet name, we close the workbook using .Close
and pass False
as the parameter to ensure that no changes are saved.
Enabling Screen Updating
Application.ScreenUpdating = True
We re-enable screen updating to restore Excel’s normal behavior.
Displaying the Sheet Name
MsgBox "The sheet name is: " & sheetName
Finally, we display the retrieved sheet name using a message box (MsgBox
) to confirm that the code worked as expected.
Modifying the Code
You can easily modify the code to suit your specific needs. Here are a few examples:
Retrieving Multiple Sheet Names
If you want to retrieve the names of multiple sheets, you can use a loop to iterate through the sheets collection. Here’s an example:
Sub GetMultipleSheetNames()
Dim wbPath As String
Dim sheetNames As String
wbPath = "C:\Path\To\Your\Workbook.xlsx"
Application.ScreenUpdating = False
With Workbooks.Open(wbPath, ReadOnly:=True)
For Each sht In .Sheets
sheetNames = sheetNames & sht.Name & vbNewLine
Next sht
.Close False
End With
Application.ScreenUpdating = True
MsgBox "The sheet names are:" & vbNewLine & sheetNames
End Sub
This code uses a For Each
loop to iterate through each sheet in the workbook and concatenates their names into the sheetNames
variable, separated by a new line (vbNewLine
). Finally, it displays all the sheet names in a message box.
Storing Sheet Names in an Array
Instead of displaying the sheet names in a message box, you can store them in an array for further processing. Here’s an example:
Sub StoreSheetNamesInArray()
Dim wbPath As String
Dim sheetNames() As String
wbPath = "C:\Path\To\Your\Workbook.xlsx"
Application.ScreenUpdating = False
With Workbooks.Open(wbPath, ReadOnly:=True)
ReDim sheetNames(1 To .Sheets.Count)
For i = 1 To .Sheets.Count
sheetNames(i) = .Sheets(i).Name
Next i
.Close False
End With
Application.ScreenUpdating = True
' Use the sheetNames array for further processing
End Sub
This code declares a dynamic array named sheetNames
to store the sheet names. Inside the With
block, it resizes the array using ReDim
to match the number of sheets in the workbook. Then, it uses a For
loop to iterate through each sheet and assigns its name to the corresponding element in the sheetNames
array. You can then use the sheetNames
array for further processing as needed.
Handling Workbooks with Password Protection
If the workbook you want to retrieve the sheet name from is password-protected, you’ll need to modify the code slightly to handle the password. Here’s an example:
Sub GetSheetNameFromProtectedWorkbook()
Dim wbPath As String
Dim sheetName As String
Dim password As String
wbPath = "C:\Path\To\Your\ProtectedWorkbook.xlsx"
password = "YourPassword"
Application.ScreenUpdating = False
With Workbooks.Open(wbPath, ReadOnly:=True, Password:=password)
sheetName = .Sheets(1).Name
.Close False
End With
Application.ScreenUpdating = True
MsgBox "The sheet name is: " & sheetName
End Sub
In this modified code, we introduce a new variable password
to store the password required to open the protected workbook. When calling the Workbooks.Open
method, we include the Password
parameter and pass the password
variable as its value.
Make sure to replace "YourPassword"
with the actual password for the protected workbook.
Handling Errors and Exceptions
When working with external workbooks, it’s important to handle potential errors and exceptions that may occur. Here’s an example of how you can modify the code to include error handling:
Sub GetSheetNameWithErrorHandling()
Dim wbPath As String
Dim sheetName As String
wbPath = "C:\Path\To\Your\Workbook.xlsx"
Application.ScreenUpdating = False
On Error Resume Next
With Workbooks.Open(wbPath, ReadOnly:=True)
If Err.Number <> 0 Then
MsgBox "Error opening the workbook: " & Err.Description
Else
sheetName = .Sheets(1).Name
.Close False
End If
End With
On Error GoTo 0
Application.ScreenUpdating = True
If sheetName <> "" Then
MsgBox "The sheet name is: " & sheetName
End If
End Sub
In this modified code, we use the On Error Resume Next
statement to prevent the code from halting if an error occurs. After opening the workbook, we check the Err.Number
property to determine if an error occurred. If an error is detected, we display an error message using MsgBox
, including the error description (Err.Description
).
If no error occurs, we proceed with retrieving the sheet name as before. Finally, we use the On Error GoTo 0
statement to restore the default error handling behavior.
We also add a conditional check before displaying the sheet name to ensure that it’s not an empty string, indicating that the retrieval was successful.
Final Thoughts
Getting the sheet name from an Excel workbook without opening it is a useful technique that can save time and resources when working with multiple workbooks or automating tasks in Excel. By using VBA and the Workbooks.Open
method with the ReadOnly
parameter set to True
, you can easily retrieve the sheet name without modifying the original workbook.
Throughout this article, we explored various scenarios and modifications to the VBA code, including retrieving multiple sheet names, storing sheet names in an array, handling password-protected workbooks, and implementing error handling.
Feel free to adapt the code examples provided to fit your specific requirements. With a little creativity, you can extend this technique to retrieve other information from workbooks without opening them, such as cell values, range addresses, or even perform calculations on the data.
Remember to always handle errors and exceptions appropriately when working with external workbooks to ensure a smooth and reliable user experience.
FAQs
What is the benefit of getting the Excel sheet name without opening the workbook?
Getting the Excel sheet name without opening the workbook can save time and resources when working with multiple workbooks or automating tasks in Excel. It allows you to retrieve the sheet name without the need to fully open and load the entire workbook, which can be particularly useful when dealing with large or complex workbooks.
Can I retrieve the names of multiple sheets using this method?
Yes, you can modify the VBA code to retrieve the names of multiple sheets. Instead of retrieving just the first sheet name, you can use a loop to iterate through the sheets collection and store or process the names of all the sheets in the workbook.
How can I handle password-protected workbooks when using this technique?
To handle password-protected workbooks, you need to modify the VBA code slightly. When opening the workbook using the Workbooks.Open
method, include the Password
parameter and provide the password as its value. This will allow the code to open the protected workbook and retrieve the sheet name.
Is it possible to retrieve other information from the workbook without opening it?
Yes, you can extend this technique to retrieve other information from the workbook without opening it. By modifying the VBA code, you can access various properties and methods of the workbook and its sheets, such as cell values, range addresses, or even perform calculations on the data. However, keep in mind that the workbook is opened in read-only mode, so you won’t be able to make any changes to the workbook using this method.
What should I do if an error occurs while trying to retrieve the sheet name?
It’s important to handle errors and exceptions when working with external workbooks. You can modify the VBA code to include error handling using the On Error Resume Next
statement. This will prevent the code from halting if an error occurs. Check the Err.Number
property to determine if an error occurred and display an appropriate error message. Use the On Error GoTo 0
statement to restore the default error handling behavior after the workbook is closed.
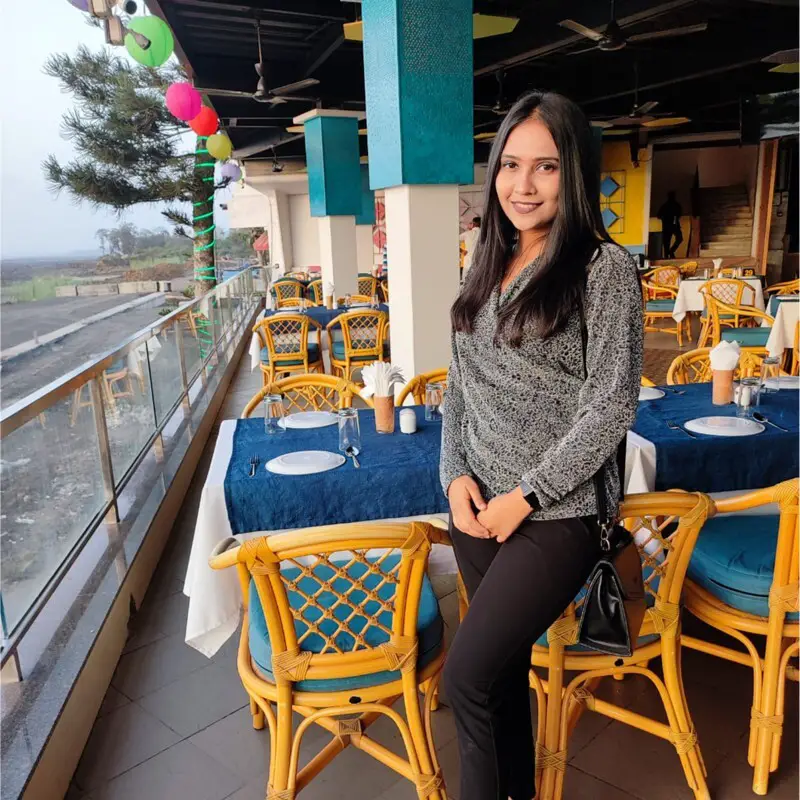
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.