Export CSV Files Without Quotes Using Excel VBA
Did you know that when exporting CSV files using Excel VBA, it is common to encounter issues where the exported file contains double quotes around each value?
This can cause problems when trying to integrate the exported data with other systems. But don’t worry, we’ve got you covered! In this article, we will learn how to export CSV files without quotes using Excel VBA, ensuring clean data transfer and seamless integration.
Exporting a Single Value as a CSV File
If you only need to export a single value as a CSV file, you can easily accomplish this using a macro in Excel VBA. One efficient approach is to define the specific value you want to export as a named range in your workbook. This allows you to reference the value directly without the need for complex data manipulation.
Here’s a step-by-step guide on how to export a single value as a CSV file without quotes:
- Open your Excel workbook and navigate to the worksheet containing the desired value.
- Select the cell that contains the value and assign it a name. To do this, go to the “Formulas” tab, click on “Define Name” in the “Defined Names” group, and enter a suitable name for the value. For this example, let’s name it “SingleValue”.
- In the VBA editor, insert the following code:
Sub ExportSingleValueAsCSV()
Dim SingleVal As Variant
SingleVal = Range("SingleValue").Value
Open "C:\Path\To\Your\Destination\File.csv" For Output As #1
Print #1, SingleVal
Close #1
End Sub
Make sure to replace “C:\Path\To\Your\Destination\File.csv” in the code with the actual destination path and file name where you want to save the CSV file. Ensure that you have write permissions to that location.
When you run the macro, it will export the single value assigned to the “SingleValue” named range as a CSV file without any quotes.
To run the macro, you can assign it to a button on your worksheet or execute it using the VBA editor. After running the macro, you can find the exported CSV file at the specified destination.
This method provides a quick and straightforward solution for exporting a single value as a CSV file without quotes using Excel VBA.
Advantages | Disadvantages |
---|---|
Easy implementation | Limited to exporting a single value |
No additional data manipulation required |
By following these steps, you can export a single value as a CSV file effortlessly with Excel VBA.
Exporting Multiple Values as a CSV File
If you have multiple values that need to be exported as a CSV file without quotes, you can easily modify the previous code to loop through the values and append them to the CSV file. This allows for efficient exporting of data in bulk, saving you time and effort.
Here’s an example of how you can export multiple values as a CSV file using Excel VBA:
Sub ExportMultipleValues()
Dim ws As Worksheet
Dim rng As Range
Dim value As Variant
Dim csvData As String
Dim filePath As String
' Set the worksheet and range containing the values
Set ws = ThisWorkbook.Worksheets("Sheet1")
Set rng = ws.Range("A1:A10") ' Modify the range as per your requirements
' Loop through each value in the range and append it to the CSV data
For Each value In rng
csvData = csvData & value & ","
Next value
' Remove the trailing comma from the CSV data
csvData = Left(csvData, Len(csvData) - 1)
' Prompt user to choose a file path to save the CSV file
filePath = Application.GetSaveAsFilename(fileFilter:="CSV Files (*.csv), *.csv")
' Check if a valid file path is provided
If filePath "False" Then
' Save the CSV data to the chosen file path
Open filePath For Output As #1
Print #1, csvData
Close #1
MsgBox "CSV file exported successfully!"
Else
MsgBox "Invalid file path. Export cancelled."
End If
End Sub
This code loops through the range specified in the Set rng
line and appends each value to the CSV data, separated by commas. The trailing comma is then removed, and the user is prompted to choose a file path to save the CSV file. If a valid file path is provided, the CSV data is saved to the chosen file path.
This method allows for easy exporting of multiple values as a CSV file without quotes, providing a streamlined approach to data management and integration.
Value 1 | Value 2 | Value 3 |
---|---|---|
Data A | Data B | Data C |
Data X | Data Y | Data Z |
Handling Different Datatypes
When exporting CSV files without quotes using Excel VBA, it is crucial to consider the datatypes of the values being exported. By default, Excel VBA treats all values as text, which can lead to issues such as numeric values being exported with leading zeros or as strings instead of actual numbers. To ensure the correct handling of different datatypes during the CSV export process, you can utilize the following code:
Sub ExportCSVWithoutQuotes()
Dim rng As Range
Dim cell As Range
Dim csvData As String
' Set the range containing the values to be exported
Set rng = ThisWorkbook.Range("A1:A5")
' Loop through each cell in the range
For Each cell In rng
' Check the datatype of the cell value
Select Case VarType(cell.Value)
' If the cell value is numeric, format it without leading zeros
Case vbDouble, vbInteger, vbLong
csvData = csvData & Format(cell.Value, "0") & ","
' If the cell value is a date, format it as a date
Case vbDate
csvData = csvData & Format(cell.Value, "mm/dd/yyyy") & ","
' If the cell value is a string, include it as is
Case vbString
csvData = csvData & cell.Value & ","
' Handle other datatypes here if needed
End Select
Next cell
' Remove the trailing comma from the csvData string
If Len(csvData) > 0 Then
csvData = Left(csvData, Len(csvData) - 1)
End If
' Export the csvData string to a CSV file
Open "C:\Path\to\export.csv" For Output As #1
Print #1, csvData
Close #1
End Sub
This code snippet demonstrates how to handle different datatypes during the CSV export process. It checks the datatype of each value before exporting it, ensuring that numeric values are formatted correctly without leading zeros and date values are exported in the desired date format. You can customize this code according to your specific requirements and the datatypes you are working with.
Additional Considerations
When exporting CSV files without quotes using Excel VBA, there are a few additional considerations to keep in mind:
- Data Validation: Before exporting the CSV file, validate the data to ensure it meets the required format and integrity. This can include checking for empty values, improper formatting, or any other inconsistencies that may cause issues.
- Character Encoding: Consider the character encoding of the exported CSV file, especially if you are dealing with international characters or special symbols. Choose an appropriate encoding format, such as UTF-8, to preserve the integrity of the data.
- File Size: Depending on the volume of data being exported, consider the file size limitations. Large CSV files can be challenging to handle and may cause performance issues. If necessary, break down the export into smaller, more manageable chunks.
- Error Handling: Implement error handling mechanisms in your Excel VBA code to gracefully handle any unexpected errors that may occur during the export process. This will ensure that the user is notified of any issues and can take appropriate actions.
By considering these factors, you can enhance the efficiency and accuracy of your CSV file exports using Excel VBA.
Sample Error Handling Code:
Here is an example of how you can implement error handling in your Excel VBA code:
“`vba
Sub ExportCSVFile()
On Error GoTo ErrorHandler
‘ Your export code here
Exit Sub
ErrorHandler:
MsgBox “An error occurred during the export process: ” & Err.Description
Exit Sub
End Sub
“`
Common Character Encoding Formats
Encoding Format | Description |
---|---|
UTF-8 | The most widely used character encoding format, supports a wide range of characters and symbols. |
ASCII | A basic character encoding format that supports only the English alphabet and a limited set of symbols. |
ISO-8859-1 | An encoding format commonly used for Western European languages, supports characters with diacritical marks. |
By understanding and addressing these additional considerations, you can ensure a smooth and successful CSV file export process using Excel VBA.
Alternative Solutions
If you are still facing issues with exporting CSV files without quotes using Excel VBA, there are alternative solutions you can explore. One option is to use third-party libraries or tools specifically designed for CSV file exports. These libraries often provide more flexibility and customization options compared to Excel VBA. Additionally, you can consider using other programming languages, such as Python or R, which have built-in functions for exporting CSV files.
Here is a comparison between Excel VBA, third-party libraries, and other programming languages for exporting CSV files:
Criteria | Excel VBA | Third-Party Libraries | Other Programming Languages |
---|---|---|---|
Flexibility | Limited | High | High |
Customization Options | Limited | High | High |
Built-in CSV Export Functions | Yes | Yes | Yes |
Learning Curve | Medium | Medium to High | Medium to High |
Integration with Existing Workflows | High | High | High |
While Excel VBA offers a basic CSV export functionality, third-party libraries and other programming languages provide more advanced features and greater control over the export process. These alternatives can handle complex data structures, offer better performance, and are often easier to learn and integrate into existing workflows.
In particular, third-party libraries like Pandas (for Python) and utils (for R) are widely used for CSV file exports due to their robust capabilities and extensive community support. These libraries provide a wide range of options for fine-tuning the exported CSV files according to specific requirements.
If you have experience or are willing to learn Python or R, exploring these alternative solutions can empower you with advanced CSV export capabilities and enhance your data integration processes.
Conclusion
Exporting CSV files without quotes using Excel VBA is a crucial skill for ensuring clean data transfer and seamless integration with other systems. By following the methods outlined in this article, you can overcome the issue of double quotes around exported values, resulting in reliable and compatible CSV files.
Whether you need to export a single value or multiple values, it is important to consider datatype handling and additional considerations for a successful CSV export. Excel VBA allows for efficient exporting of single values as well as multiple values by using macros and looping techniques. However, with the default settings, you may encounter challenges with numeric values being treated as text.
Therefore, it is crucial to ensure proper handling of different datatypes when exporting CSV files without quotes. By utilizing the appropriate code snippets and techniques provided in this article, you can correctly export numeric values and avoid leading zeros or numeric values being exported as strings.
If you find that Excel VBA does not meet your specific requirements or if you need more flexibility, there are alternative solutions available. Third-party libraries and tools explicitly designed for CSV file exports offer enhanced customization options. Additionally, considering other programming languages like Python or R, which include built-in functions for exporting CSV files, can provide further flexibility.
FAQ
What issues can occur when exporting CSV files using Excel VBA?
The exported file may contain double quotes around each value, which can cause problems when integrating the data with other systems.
How can I export a single value as a CSV file without quotes using Excel VBA?
You can define the value as a named range in your workbook and use a macro to save it as a CSV file without quotes.
How can I export multiple values as a CSV file without quotes using Excel VBA?
You can modify the code to loop through the values and append them to the CSV file.
What should I consider when exporting CSV files without quotes in terms of datatypes?
Excel VBA treats all values as text by default, so numeric values may be exported with leading zeros or as strings. You can handle different datatypes correctly using specific code.
What additional considerations should I keep in mind when exporting CSV files without quotes?
Ensure compatibility with other systems, check for any encoding issues, and test the exported file in different scenarios.
What alternative solutions can I explore if I am facing issues with exporting CSV files without quotes using Excel VBA?
You can use third-party libraries or tools designed for CSV file exports, or consider using other programming languages like Python or R that have built-in functions for exporting CSV files.
How can exporting CSV files without quotes using Excel VBA benefit clean data transfer and seamless integration?
By following the methods outlined in this article, you can overcome the issue of double quotes around exported values and ensure smooth integration with other systems.
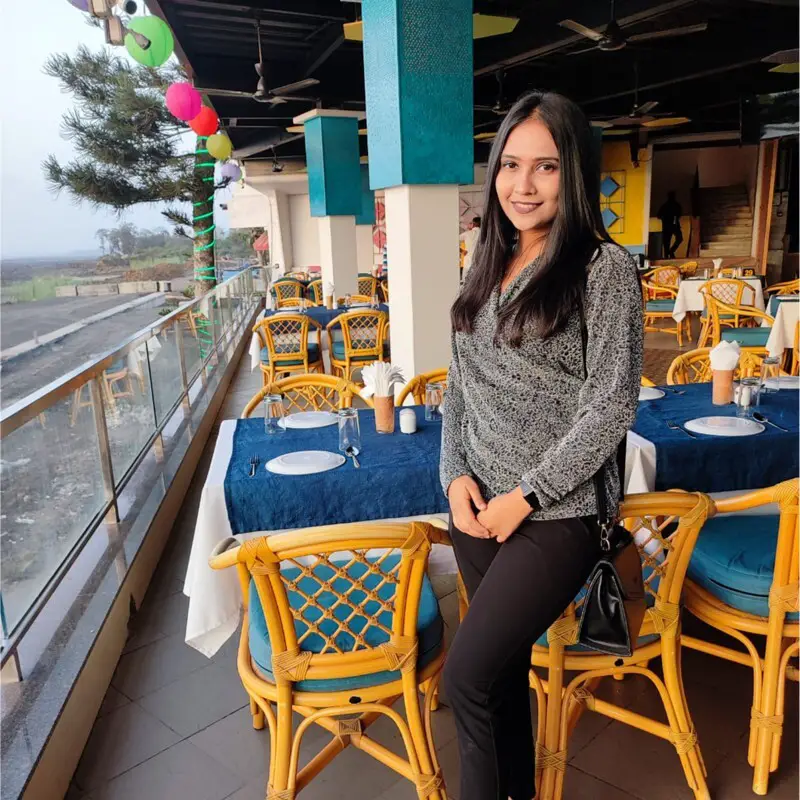
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.