How to Get a Button by Name in Excel VBA: Easy Guide
In Excel VBA, working with buttons can help automate tasks and create user-friendly interfaces. One common requirement is to reference or manipulate a button by its name. In this guide, we will teach you how to use VBA to get a button by its name and perform different operations. We will also cover the necessary VBA methods and syntax to manage controls effectively.
Understanding VBA Buttons
In Excel, buttons can be created using the Developer Tab under the Form Controls or ActiveX Controls section. These buttons are used to trigger VBA macros when clicked. In VBA, buttons are referred to as OLEObjects (ActiveX) or Shapes (Form Controls).
Types of Buttons in Excel
There are two main types of buttons:
- Form Controls – These are easier to use but have limited customization.
- ActiveX Controls – These provide more flexibility and event-driven capabilities.
Note: This article focuses on ActiveX buttons, but the code can be adjusted for Form Control buttons if needed.
Setting Up the Environment
Before diving into the code, ensure that the Developer Tab is visible in your Excel interface. If it’s not, follow these steps:
- Open Excel.
- Click on File > Options.
- In the Excel Options dialog box, select Customize Ribbon.
- Check the box next to Developer and click OK.
With the Developer Tab enabled, you can now insert buttons and access the Visual Basic for Applications (VBA) editor.
How to Get a Button by Name in Excel VBA
In Excel VBA, you can refer to buttons by their name to perform actions like enabling, disabling, changing text, or triggering a macro. Below are the methods you can use to get a button by its name.
Step 1: Accessing the VBA Editor
- Go to the Developer Tab.
- Click on Visual Basic or press
Alt + F11
to open the VBA editor. - Insert a module by clicking Insert > Module.
Step 2: Writing the VBA Code
To get a button by its name, the key is to reference the button object from the appropriate collection. In the case of ActiveX buttons, they are part of the OLEObjects
collection, while Form Control buttons are part of the Shapes
collection.
Example 1: Get an ActiveX Button by Name
The following code demonstrates how to get an ActiveX button by its name and change its caption:
Sub GetActiveXButtonByName()
Dim btn As OLEObject
' Get the button by its name
Set btn = ActiveSheet.OLEObjects("Button1")
' Change the caption of the button
btn.Object.Caption = "New Caption"
End Sub
In this code:
- “Button1” is the name of the ActiveX button.
- The OLEObjects collection is used to reference the button.
- The Caption property is updated to “New Caption.”
Example 2: Get a Form Control Button by Name
If you are working with Form Control buttons, you can use the Shapes
collection:
Sub GetFormControlButtonByName()
Dim btn As Shape
' Get the button by its name
Set btn = ActiveSheet.Shapes("Button 1")
' Change the text on the button
btn.TextFrame.Characters.Text = "New Caption"
End Sub
Here, the Shapes collection is used, and the TextFrame.Characters.Text property changes the button text.
Naming Buttons in Excel
It’s important to name your buttons appropriately for easier reference in VBA. Here’s how to rename a button:
- Select the button on the worksheet.
- In the Name Box (next to the formula bar), enter a new name (e.g.,
MyButton
). - Press Enter to save the name.
Using descriptive names like SubmitButton or ClearFormButton makes it easier to work with buttons in your VBA code.
Performing Actions with Buttons
Once you can reference a button by its name, you can perform various actions. Below are common tasks you can accomplish using VBA.
Enabling or Disabling a Button
Sometimes, you may want to disable a button based on certain conditions. Use the Enabled property to achieve this:
Sub DisableButtonByName()
Dim btn As OLEObject
Set btn = ActiveSheet.OLEObjects("Button1")
' Disable the button
btn.Object.Enabled = False
End Sub
To enable the button again, simply set Enabled to True
.
Changing the Button’s Color
For ActiveX buttons, you can also change the background color:
Sub ChangeButtonColor()
Dim btn As OLEObject
Set btn = ActiveSheet.OLEObjects("Button1")
' Change the button background color to light blue
btn.Object.BackColor = RGB(173, 216, 230)
End Sub
Assigning a Macro to a Button
You can assign a macro to run when a button is clicked. This is done by setting the OnAction property for Form Control buttons:
Sub AssignMacroToButton()
Dim btn As Shape
Set btn = ActiveSheet.Shapes("Button 1")
' Assign the macro "MyMacro" to the button
btn.OnAction = "MyMacro"
End Sub
Handling Button Events
In ActiveX buttons, you can handle events such as clicking. Events allow you to run specific code when an action is performed. Here’s how to handle a button click event:
Step 1: Insert an ActiveX Button
- Go to the Developer Tab.
- Click Insert > ActiveX Controls > Command Button.
Step 2: Add an Event Handler
Right-click the button and choose View Code. The following code will be generated automatically:
Private Sub CommandButton1_Click()
MsgBox "Button Clicked"
End Sub
Whenever the button is clicked, the MsgBox will display a message saying “Button Clicked.”
Using Loops to Get Buttons by Name
If you have multiple buttons and want to loop through them to perform actions based on their names, you can use a For Each loop. Here’s an example of how to loop through all ActiveX buttons on a worksheet:
Sub LoopThroughButtons()
Dim btn As OLEObject
For Each btn In ActiveSheet.OLEObjects
If btn.Name = "Button1" Then
' Perform an action with Button1
btn.Object.Caption = "Found Button1"
End If
Next btn
End Sub
This code loops through all OLEObjects and checks if the button’s name matches “Button1.” If it does, the caption is changed.
Working with Multiple Buttons Using Arrays
In some cases, you might want to reference multiple buttons by storing their names in an array. This is useful when you want to perform the same action on multiple buttons:
Sub GetMultipleButtonsByName()
Dim btnNames As Variant
Dim i As Integer
btnNames = Array("Button1", "Button2", "Button3")
For i = LBound(btnNames) To UBound(btnNames)
Dim btn As OLEObject
Set btn = ActiveSheet.OLEObjects(btnNames(i))
' Change the caption of each button
btn.Object.Caption = "Updated " & btnNames(i)
Next i
End Sub
In this example, three button names are stored in an array, and the caption of each button is updated in the loop.
Best Practices for Working with Buttons in VBA
Here are a few tips to ensure that your VBA code for handling buttons is efficient and maintainable:
- Use Descriptive Names: Always give your buttons descriptive names. This makes your code easier to read and maintain.
- Organize Buttons by Group: If you have multiple buttons performing related tasks, group them logically in your code.
- Error Handling: Always implement error handling, especially when dealing with controls that may or may not exist.
Table of Common Button Properties
Property | Description |
---|---|
Caption | The text displayed on the button. |
Enabled | Enables or disables the button. |
Visible | Shows or hides the button. |
BackColor | Changes the background color of the button (ActiveX only). |
OnAction | Assigns a macro to the button (Form Controls only). |
Final Thoughts
Working with buttons in Excel VBA can significantly enhance your ability to automate tasks and create interactive Excel sheets. Whether you’re getting a button by its name, changing its properties, or handling events, this guide has covered all the basics. By applying these techniques, you can streamline your workflows and improve your Excel applications.
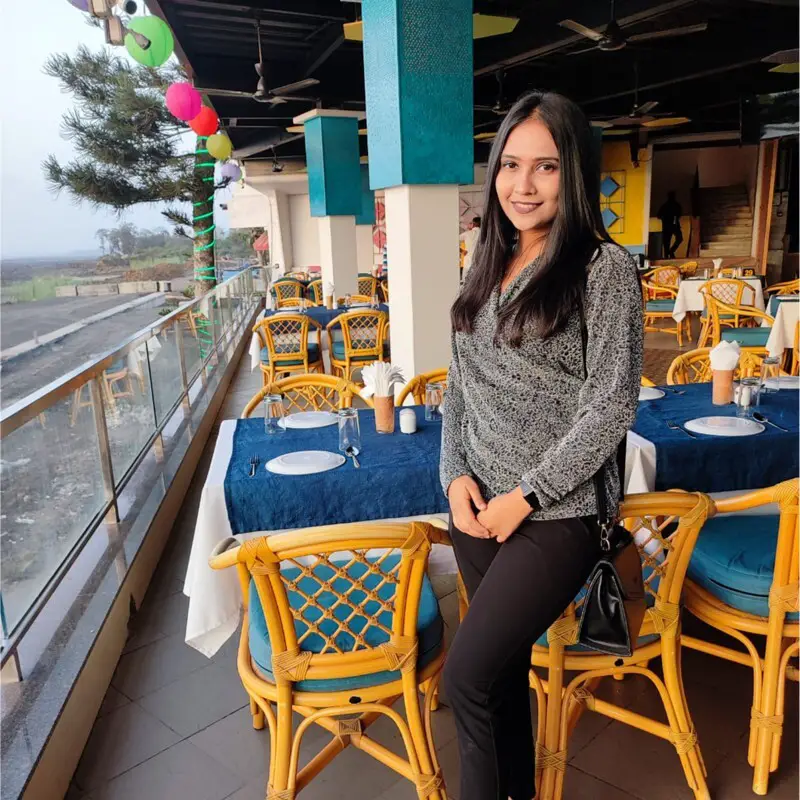
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.