VBA Excel: Insert Formulas into Cells | Tutorial
Did you know that by using VBA code in Excel, you can automate the process of inserting formulas into cells, making your spreadsheets more efficient and dynamic?
When it comes to working with complex calculations or large datasets, manually entering formulas can be time-consuming and prone to errors. However, with VBA, you can streamline this process and ensure accurate formula insertion with just a few lines of code.
In this tutorial, we will explore how to use VBA to insert formulas into cells, troubleshoot common issues, optimize your code for better performance, handle variables and complex criteria in formulas, and even transfer your VBA code to other workbooks.
If you’re looking to supercharge your Excel skills and save time on formula insertion, this tutorial is for you. Let’s dive in and discover the power of VBA in Excel!
How to use VBA to insert formulas into cells
To insert formulas into cells using VBA, you can utilize the Formula property of the Range object. This property allows you to assign a formula to a specific cell using VBA code. By leveraging the power of VBA, you can automate the process of entering formulas and make your spreadsheet more dynamic.
When using VBA to insert formulas into cells, you can specify the formula as a string within the code. This allows you to use the same syntax as you would when manually entering the formula in the formula bar. The Formula property provides a flexible and efficient way to enter complex formulas that reference other cells or sheets, and perform calculations with multiple criteria.
To use VBA to insert a formula into a cell, you need to follow these steps:
- Select the cell where you want to insert the formula.
- Access the Formula property of the Range object.
- Assign the formula as a string to the Formula property.
- Execute the VBA code to insert the formula into the cell.
Here’s an example of how you can use VBA to insert a formula into cell A1:
Sub InsertFormula()
Range("A1").Formula = "=SUM(B1:B5)"
End Sub
In this example, the formula “=SUM(B1:B5)” is assigned to cell A1 using VBA code. When you run the Sub procedure, the formula will be inserted into the cell, and the result of the sum of cells B1 to B5 will be displayed.
You can also use variables within the formula to make it more dynamic. This allows you to reference different cells or ranges based on the values of the variables. By incorporating variables and complex criteria, you can create more versatile formulas that adapt to changes in your spreadsheet.
Remember to ensure the syntax of the formula is correct when using it in VBA code. Any errors in the formula may result in unexpected results or errors when running the code. It’s always a good practice to test your code and verify the accuracy of the inserted formulas.
By using VBA to insert formulas into cells, you can streamline your workflow, save time, and create powerful and efficient spreadsheets that meet your specific needs.
Common issues and solutions when using VBA to insert formulas
When utilizing VBA to insert formulas into cells, it is not uncommon to encounter certain issues that may result in formulas returning “#N/A” or incorrect results. These issues can be frustrating, but they can be resolved by paying attention to a few key factors. Let’s explore some common problems and their solutions:
Incorrect referencing of cells or sheets within the formula
One common issue is when the formula incorrectly references cells or sheets within the VBA code. To avoid this, it is crucial to accurately reference the cells or sheets using the correct syntax. Double-check your formula’s cell references and ensure they are correctly specified.
Inconsistent data types in cells used within the formula
Another issue that may arise is inconsistent data types in the cells used within the formula. When working with formulas, it is essential to ensure that the data types are consistent and properly formatted. This ensures that the formula functions as intended and produces accurate results. Verify the data type consistency and format the cells accordingly.
If you encounter the issue of VBA formula returning “#N/A” or producing incorrect results, you can troubleshoot it by:
- Checking the syntax of the formula: Ensure that the formula syntax is correct and follows the conventions of the specific function or calculation you are using.
- Verifying the data in the cells used in the formula: Double-check the data in the cells referenced by the formula. Ensure that the data is accurate, properly formatted, and appropriate for the specific calculation or function.
By addressing these common issues and following the suggested solutions, you can resolve problems related to VBA formulas returning “#N/A” or producing incorrect results. This will help you create more reliable and efficient VBA code for inserting formulas in Excel.
Example Table:
Error | Potential Cause | Solution |
---|---|---|
#N/A | Incorrect cell or sheet referencing | Double-check formula syntax and ensure accurate referencing |
#N/A | Inconsistent data types in cells used in the formula | Verify and format cell data types consistently |
Incorrect results | Incorrect formula syntax or referencing | Review and correct formula syntax and referencing |
By following the troubleshooting steps and solutions outlined above, you can overcome common issues that arise when using VBA to insert formulas in Excel.
Optimizing VBA code for inserting formulas
When working with large spreadsheets and inserting formulas using VBA, it is crucial to optimize your code to improve performance and enhance the speed and efficiency of your spreadsheet. One effective way to optimize your VBA code is to minimize the number of times Excel recalculates the formulas. You can achieve this by using the following techniques:
1. Turn off Screen Updating
You can prevent Excel from redrawing the screen for every formula insertion by turning off screen updating. This can significantly improve the performance of your VBA code. To do this, use the Application.ScreenUpdating property and set it to False before inserting the formulas. Remember to turn it back on after completing the calculations. Here is an example:
“`vba
Application.ScreenUpdating = False
‘ Insert your formulas here
Application.ScreenUpdating = True
“`
2. Disable Automatic Calculation
Excel automatically recalculates formulas whenever there is a change in the workbook. To avoid unnecessary recalculations, you can turn off automatic calculation using the Application.Calculation property. By setting it to xlCalculationManual, you can manually recalculate the formulas only when necessary. Here’s how you can do it:
“`vba
Application.Calculation = xlCalculationManual
‘ Insert your formulas here
Application.Calculation = xlCalculationAutomatic
“`
By implementing these optimization techniques, you can significantly improve the performance of your VBA code and reduce the time it takes to insert formulas into your spreadsheet.
Optimization Technique | Description |
---|---|
Turn off Screen Updating | Prevents Excel from redrawing the screen for every formula insertion, improving performance. |
Disable Automatic Calculation | Turns off automatic calculation, enabling manual recalculation of formulas when necessary. |
Inserting formulas with variables and complex criteria
In some cases, you may need to insert formulas with variables or complex criteria using VBA. This allows you to dynamically reference cells or sheets in the formula, making it more versatile. To do this, you can concatenate the variables or criteria within the formula string using ampersands. For example, you can use variables to specify the range of cells or the lookup criteria in the formula. By incorporating variables and complex criteria, you can create more dynamic formulas that adapt to changes in your spreadsheet.
When working with VBA, using variables in formulas can greatly enhance your spreadsheet’s functionality. Variables are placeholders that can be assigned values and used within your VBA code. By inserting formulas with variables, you can build dynamic formulas that adapt to changes in your data or calculations.
Here’s an example of how you can use variables in a formula:
Dim Variable1 As Double
Variable1 = 10
Range("A1").Formula = "=B1 * " & Variable1
In this example, we declare a variable named “Variable1” and assign it a value of 10. Then, we use the ampersand (&) to concatenate the variable with the rest of the formula string. The result is a dynamic formula that multiplies the value in cell B1 by the value of Variable1.
Furthermore, you can use complex criteria in your formulas with VBA. This allows you to perform calculations based on multiple conditions and criteria. For example, you can use the IF statement in conjunction with variables to create formulas that evaluate different scenarios and return different results.
Here’s an example of how you can use complex criteria in a formula:
Dim Variable1 As Double
Variable1 = 10
Range("A1").Formula = "=IF(B1 > " & Variable1 & ", "Good", "Bad")
In this example, we use the IF statement to evaluate whether the value in cell B1 is greater than the value of Variable1. If it is, the formula returns “Good”; otherwise, it returns “Bad”. By incorporating complex criteria in your formulas, you can create powerful calculations that adapt to changing conditions.
By using variables and complex criteria in your formulas with VBA, you can significantly enhance the flexibility and functionality of your spreadsheets. Whether you need to dynamically reference cells or perform calculations based on multiple conditions, VBA provides the tools you need to create dynamic and adaptable formulas.
Benefits of inserting formulas with variables and complex criteria: |
---|
1. Increased flexibility and adaptability of formulas |
2. Ability to dynamically reference cells and sheets |
3. Enhanced calculations based on multiple conditions |
4. Greater functionality and versatility of spreadsheets |
Transferring VBA code to other workbooks
If you’re looking to transfer your VBA code to another workbook and want to reference an external workbook for formula insertion, it can be easily accomplished. Simply specify the workbook and worksheet names in your code to establish the necessary connections. By utilizing the Workbooks.Open
method, you can open the external workbook and set the workbook object, allowing you to access its contents. With the workbook object in place, you can take advantage of the ListObjects
property to refer to tables within the external workbook for formula insertion. By correctly referencing the external workbook and its tables, you can ensure the seamless execution of your VBA code across different workbooks.
Transferring VBA code to another workbook and referencing an external workbook is particularly useful when working with complex spreadsheets that require data from different sources. Whether you’re consolidating information from multiple workbooks or performing calculations across different datasets, this approach streamlines your workflow and automates the formula insertion process. By leveraging the power of VBA, you can build dynamic and efficient Excel workbooks that cater to your specific needs.
FAQ
How can I use VBA to insert formulas into cells in Excel?
What is the syntax for inserting formulas with VBA?
What issues can I encounter when using VBA to insert formulas into cells?
How can I optimize my VBA code for inserting formulas?
Can I insert formulas with variables or complex criteria using VBA?
How can I transfer my VBA code to another workbook and reference an external workbook for formula insertion?
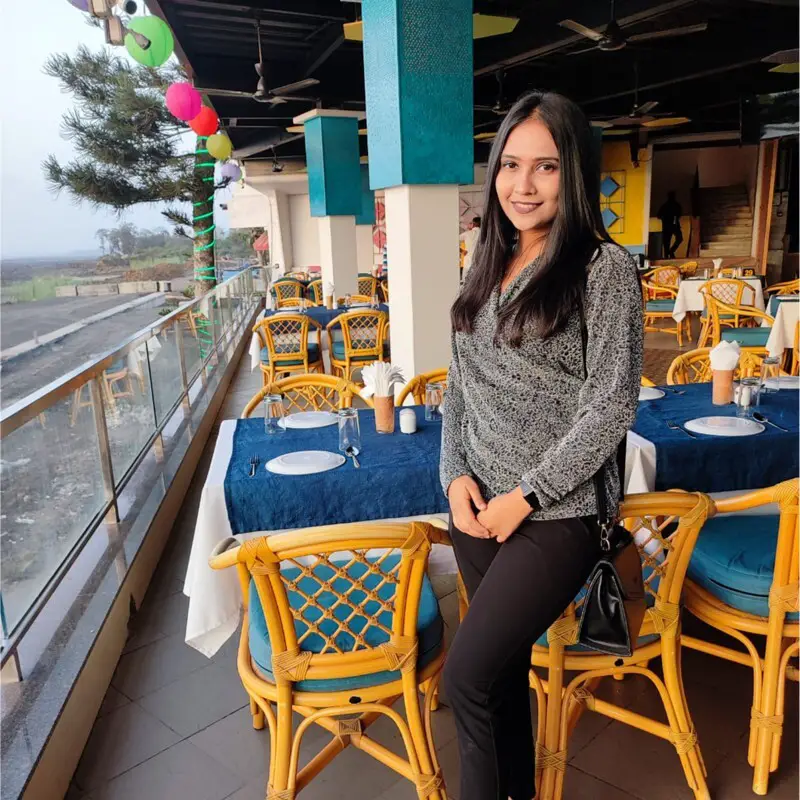
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.