How to Open an Excel Workbook with a Variable Name Using VBA?
Are you looking to open an Excel workbook using a variable name in VBA? Using variables to represent workbook names allows you to write more flexible and dynamic VBA code. In this article, we’ll show you exactly how to open an Excel workbook with a variable name using VBA.
Understanding the Workbooks.Open Method
The key to opening a workbook using a variable name is the Workbooks.Open method. This VBA method allows you to open a workbook by specifying its file path. The basic syntax is:
Workbooks.Open (“filepath”)
Where “filepath” is a string representing the full path to the Excel file you want to open, enclosed in quotes.
For example, to open a workbook named “Sales Report.xlsx” located in the “Documents” folder, you would use:
Workbooks.Open (“C:\Documents\Sales Report.xlsx”)
The Workbooks.Open method has several optional parameters you can use to control how the workbook is opened:
Parameter | Description |
---|---|
UpdateLinks | Specifies how links in the workbook are updated (0=Don’t update, 1=Update external links, 2=Update remote links, 3=Update both) |
ReadOnly | Specifies if the workbook is opened in read-only mode (True/False) |
Format | Specifies the delimiter and data format when opening a text file as a workbook |
Password | Specifies the password to open a protected workbook |
WriteResPassword | Specifies the password to modify a write-reserved workbook |
IgnoreReadOnlyRecommended | Specifies if read-only recommendations are ignored (True/False) |
Origin | Specifies the origin of delimited data when opening a text file as a workbook |
Delimiter | Specifies the delimiter character when opening a text file as a workbook |
Editable | Specifies if the workbook is opened in edit mode (True/False) |
Notify | Specifies if the user is notified when the workbook is opened (True/False) |
Converter | Specifies the converter to use when opening the workbook |
AddToMru | Specifies if the workbook is added to the most recently used (MRU) file list (True/False) |
You likely won’t need to use most of these parameters, but it’s good to be aware of the options available.
Using a Variable to Represent the File Path
Rather than hardcoding the file path into the Workbooks.Open method, you can store it in a variable. This makes your code more flexible, as you can easily change the workbook being opened by modifying the variable’s value.
To use a variable to represent the file path:
- Declare a String variable to hold the file path
- Assign the desired file path to the variable
- Use the variable inside the Workbooks.Open method
Here’s an example:
Dim wbPath As String
wbPath = "C:\Documents\Sales Report.xlsx"
Workbooks.Open (wbPath)
You can also assign the file path to the variable using the InputBox function, allowing the user to select the workbook file themselves:
Dim wbPath As String
wbPath = Application.GetOpenFilename(Title:="Select Workbook to Open")
If wbPath <> "False" Then
Workbooks.Open (wbPath)
Else
MsgBox "No workbook was selected."
End If
This code displays an “Open” dialog box with the title “Select Workbook to Open”. If the user selects a workbook file, its path is assigned to the wbPath variable and the workbook is opened. If the user cancels the dialog, the variable will contain the string value “False”.
Building the File Path Using Concatenation
In many cases, you’ll need to construct the file path dynamically based on values stored in variables. To do this, use the concatenation operator (&) to join the variable values together into a complete file path string.
For instance, to build a file path from separate variables representing the directory, workbook name, and file extension:
Dim wbDir As String
Dim wbName As String
Dim wbExt As String
Dim wbPath As String
wbDir = "C:\Documents\"
wbName = "Sales Report"
wbExt = ".xlsx"
wbPath = wbDir & wbName & wbExt
Workbooks.Open (wbPath)
This code will open the “Sales Report.xlsx” workbook located in the “C:\Documents\” directory.
You can also use the Format function to construct the file path using placeholders:
Dim wbPath As String
Dim wbName As String
wbName = "Sales Report"
wbPath = "C:\Documents\{0}.xlsx"
wbPath = Format(wbPath, wbName)
Workbooks.Open (wbPath)
This code uses the Format function to replace the {0} placeholder in the wbPath string with the value of the wbName variable.
Handling Possible Errors
When opening workbooks using variable names, it’s important to add error handling in case the specified workbook doesn’t exist or there are issues accessing it. Use the On Error statement to gracefully handle any runtime errors.
Here’s an example that checks if the workbook exists before trying to open it:
Dim wbPath As String
wbPath = "C:\Documents\Sales Report.xlsx"
On Error Resume Next
Workbooks.Open (wbPath)
If Err.Number <> 0 Then
Select Case Err.Number
Case 1004
MsgBox "The specified workbook could not be found."
Case 76
MsgBox "The specified path was invalid."
Case Else
MsgBox "An unexpected error occurred: " & Err.Description
End Select
End If
On Error GoTo 0
This code attempts to open the workbook. If an error occurs, it checks the error number (Err.Number) and displays a specific message based on the error using a Select Case statement. Errors 1004 and 76 are likely error numbers if the file is missing or the path is invalid. Any other errors will display the generic error description.
Opening Workbooks in a Loop Using Variables
A common scenario is needing to open multiple workbooks, one at a time, using a loop. By storing the workbook names or paths in an array variable, you can easily loop through and open each one.
Here’s an example that opens three workbooks in a loop:
Dim wbNames(1 To 3) As String
Dim i As Integer
wbNames(1) = "Sales Report"
wbNames(2) = "Inventory Count"
wbNames(3) = "Customer Orders"
For i = 1 To 3
Workbooks.Open ("C:\Documents\" & wbNames(i) & ".xlsx")
Next i
This code stores the workbook names in the wbNames array variable. It then loops through the array using a For Next loop, opening each workbook by building its file path dynamically.
You can also use the Dir function to loop through all Excel files in a specific directory:
Dim wbPath As String
wbPath = "C:\Documents\"
Dim wbFile As String
wbFile = Dir(wbPath & "*.xlsx")
Do While wbFile <> ""
Workbooks.Open (wbPath & wbFile)
wbFile = Dir()
Loop
This code uses the Dir function to get the first Excel file (.xlsx) in the “C:\Documents\” directory. It then opens that workbook and uses Dir again to get the next file. The loop continues until Dir returns an empty string, meaning no more files were found.
Best Practices for Opening Workbooks Using Variables
To ensure your VBA code that opens workbooks using variables is reliable and efficient, follow these best practices:
Best Practice | Description |
---|---|
Use absolute file paths | Whenever possible, use a complete file path (like “C:\Folder\file.xlsx”) rather than a relative path, to avoid issues. |
Include the file extension | Always include the workbook’s full file extension (like “.xlsx”) to prevent VBA from having to guess the correct extension. |
Implement error handling | Add error handling code to gracefully deal with scenarios where the specified workbook file is missing or inaccessible. |
Ensure variables are declared and initialized | Always declare your variables and initialize them with a starting value to avoid potential errors. |
Close unneeded workbooks | After your code finishes working with an opened workbook, close it using the Workbook.Close method to free up memory and resources. |
Use meaningful variable names | Give your variables descriptive, meaningful names to make your code more readable and maintainable. |
Avoid hard-coding values | Whenever possible, avoid hard-coding things like file paths and sheet names directly in your code. Use variables instead. |
By following these tips, you can write VBA code to open workbooks using variables that is robust and efficient.
Final Thoughts
Opening an Excel workbook with a variable name using VBA is a powerful technique that allows you to write flexible, dynamic code. By representing workbook file paths with String variables, you can easily open different workbooks without having to modify your code.
The key steps are:
- Use the Workbooks.Open method to open the workbook
- Store the file path in a String variable
- Concatenate variable values to construct the full file path
- Implement error handling to deal with missing or inaccessible files
- Follow best practices like using absolute paths and closing unneeded workbooks
By mastering these techniques, you’ll be able to automate workbook operations more effectively using VBA in Excel. Whether working with a single workbook or opening multiple files in a loop, using variables to represent workbook names will make your code more versatile and easier to maintain.
FAQs
What is the main method used to open a workbook with a variable name in VBA?
How do you store the file path of the workbook in a variable?
Dim wbPath As String
wbPath = "C:\Documents\Sales Report.xlsx"
What is the benefit of using variables to represent workbook names in VBA?
How can you construct the file path dynamically using variables?
Dim wbDir As String
Dim wbName As String
Dim wbExt As String
Dim wbPath As String
wbDir = "C:\Documents\"
wbName = "Sales Report"
wbExt = ".xlsx"
wbPath = wbDir & wbName & wbExt
Why is error handling important when opening workbooks using variables?
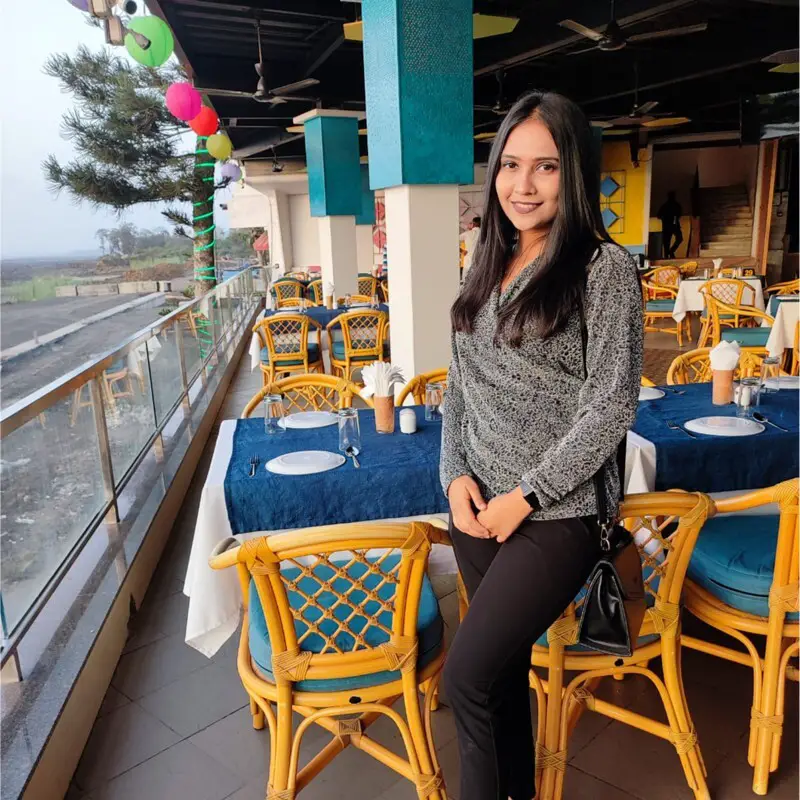
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.