Excel VBA Paste Array to Range – Quick Guide
Did you know that Excel VBA allows you to efficiently paste arrays into ranges, saving you valuable time and effort? Instead of manually looping through each cell, you can use powerful techniques to streamline your data manipulation tasks.
In this quick guide, we will explore different methods and techniques for pasting arrays to ranges using Excel VBA. Whether you’re working with large datasets or need to automate repetitive tasks, these approaches will enhance your productivity and optimize your workflow.
Key Takeaways:
- Excel VBA provides efficient ways to paste arrays into ranges.
- The Transpose function allows you to change the orientation of an array, optimizing the paste operation.
- Directly assigning the range value to the array eliminates the need for looping.
- Filling arrays and copying them to worksheets offer flexibility in data manipulation.
- Understanding additional array operations empowers developers to enhance their data manipulation capabilities.
Using the Transpose Function
One efficient method to paste an array into a range without looping is by using the Transpose function in Excel VBA. This function allows us to change the orientation of an array, converting rows to columns and vice versa. By transposing the array before pasting, we can directly assign the transposed array to the target range, effectively pasting the entire array in one step.
The Transpose function is particularly useful when dealing with large arrays or when we want to quickly convert rows into columns or vice versa. Here’s an example of how to use the Transpose function:
Sub PasteArrayWithTranspose()
Dim sourceArray As Variant
Dim targetRange As Range
' Define the source array
sourceArray = Array(1, 2, 3, 4, 5)
' Define the target range
Set targetRange = Range("A1:E1")
' Transpose the array before pasting
targetRange.Value = Application.WorksheetFunction.Transpose(sourceArray)
End Sub
In the above example, we define a source array consisting of numbers 1 to 5. We then define the target range where we want to paste the array, which in this case is a row range from column A to column E. By using the Transpose function, the array is transposed and directly assigned to the target range using the Value property of the range object.
This method provides a simple and efficient way to paste arrays into ranges without the need for looping or individual cell assignments. It is especially useful for scenarios where we want to quickly copy and paste data from one range to another or when performing calculations that require data in a different orientation.
Advantages of Using the Transpose Function
- Efficiently paste arrays into ranges without looping.
- Quickly convert rows to columns or vice versa.
- Saves time and simplifies data manipulation tasks.
By leveraging the power of the Transpose function, Excel VBA developers can streamline their code and enhance the efficiency of data manipulation operations. The Transpose function is a valuable tool to have in your Excel VBA toolkit when working with arrays and pasting data into ranges.
Directly Assigning the Range Value to the Array
In addition to using the Transpose function, another efficient approach to paste an array into a range without looping is by directly assigning the range’s value to the array. This method simplifies the process by creating a 2D array, where the rows and columns correspond to the range dimensions.
To implement this method, the target range needs to be resized to match the size of the array. By doing so, we ensure that the array will fit perfectly into the range. This approach eliminates the need for manual iteration through each cell, resulting in a faster and more streamlined solution.
Step 1: Create and Resize the Target Range
Before assigning the array to the range, we need to create the target range and resize it to match the array dimensions. The following code snippet demonstrates how to achieve this:
“`vba
Dim arrayRange As Range
Set arrayRange = ThisWorkbook.Worksheets(“Sheet1”).Range(“A1”).Resize(UBound(myArray, 1), UBound(myArray, 2))
“`
In this example, the arrayRange variable represents the target range where we want to paste the array. By using the Resize method, the range is resized based on the number of rows and columns in the array.
Step 2: Assign the Array to the Range
Once the target range is created and resized, we can assign the array directly to the range using the following code:
“`vba
arrayRange.Value = myArray
“`
By assigning the array using the Value property of the range, the entire array will be pasted into the range in one go. This method provides a more efficient way to transfer data between arrays and ranges, especially when dealing with large datasets.
Here is an example of how this method can be implemented:
“`vba
Sub PasteArrayToRange()
Dim myArray As Variant
myArray = Array(Array(1, 2, 3), Array(4, 5, 6), Array(7, 8, 9))
Dim arrayRange As Range
Set arrayRange = ThisWorkbook.Worksheets(“Sheet1”).Range(“A1”).Resize(UBound(myArray, 1), UBound(myArray, 2))
arrayRange.Value = myArray
End Sub
“`
By directly assigning the range value to the array, we can efficiently paste the entire array into a range without the need for looping or manual cell-by-cell manipulation. This method provides a streamlined solution for handling large datasets and improves the performance of Excel VBA macros.
Filling Arrays and Copying to Worksheets
In Excel VBA, filling an array with values and copying it to a worksheet can be achieved through a combination of different techniques. This section explores two methods: manually populating the array and using the Transpose function to paste it into the range, or directly taking values from a worksheet range and filling the array. Both approaches provide flexibility in data manipulation and array construction, allowing for efficient pasting of arrays into ranges.
Manually Populating Arrays
One way to fill an array is by manually populating it with values. This method is useful when you have specific data that you want to store in an array and then copy to a worksheet.
Here’s an example code snippet that demonstrates how to manually fill an array and paste it into a range using the Transpose function:
' Declare and populate the array Dim myArray(1 To 5) As Variant myArray(1) = "Apple" myArray(2) = "Banana" myArray(3) = "Orange" myArray(4) = "Grapes" myArray(5) = "Mango" ' Transpose the array and paste it to Range A1:A5 Range("A1:A5").Value = Application.Transpose(myArray)
This code snippet creates and populates an array called myArray with five fruit names. The array is then transposed using the Transpose function before being assigned to the range A1:A5 in the worksheet, effectively pasting the array data into the range.
Copying Values from a Worksheet Range
Alternatively, you can directly take values from a worksheet range and fill an array with them. This method is useful when you want to extract data from a specific range in a worksheet and perform calculations or manipulations on the array.
Here’s an example code snippet that demonstrates how to copy values from a worksheet range to an array:
' Define the range and array variables Dim rng As Range Dim myArray() As Variant ' Set the range variable to the desired range Set rng = Range("A1:A5") ' Assign the range values to the array myArray = rng.Value
This code snippet defines a range variable, rng, and an array variable, myArray. The range variable is set to the desired range (in this case, A1:A5), and the values from the range are assigned to the array using the Value property.
By filling an array with values and utilizing the Transpose function or copying values directly from a worksheet range, you can effectively paste arrays into ranges in Excel VBA, simplifying data manipulation and enhancing your coding efficiency.
Additional Array Operations and Examples
In Excel VBA, arrays offer a wide range of operations that can greatly enhance your data manipulation capabilities. Let’s explore some of these operations and see how they can be applied to efficiently paste arrays into ranges.
One useful operation is passing and receiving arrays. You can pass arrays as arguments to procedures and functions, allowing for more flexible and streamlined code. By receiving arrays as parameters, you can perform calculations and manipulations directly on the array data, eliminating the need for unnecessary copying and pasting.
Another important operation is comparing two arrays. Excel VBA provides various functions and methods that enable you to compare arrays element by element, identify differences, and perform actions based on the comparison results. This can be particularly useful when dealing with large datasets and performing data validation.
Additionally, you can dynamically fill arrays using loops and conditions. This means you can dynamically adjust the size of your array based on your data requirements, allowing for more efficient memory usage. By dynamically filling an array with data, you can then easily paste the array into a range, completing the task in a single step.
By leveraging these array operations and examples, Excel VBA developers can optimize their data manipulation workflows and efficiently paste arrays into ranges, saving time and enhancing productivity. These techniques not only simplify the process but also provide greater flexibility and control over your data. Make the most out of Excel VBA’s array capabilities and unlock the full potential of your data manipulation tasks.
FAQ
How can I efficiently paste arrays into ranges using Excel VBA?
You can efficiently paste arrays into ranges in Excel VBA by using different methods and techniques. One approach is to use the Transpose function to change the orientation of the array before pasting it into the range. Another method is to directly assign the range’s value to the array, creating a 2D array and resizing the range to match the array size.
How do I use the Transpose function in Excel VBA to paste arrays into ranges?
To use the Transpose function, you first need to change the orientation of the array by converting rows to columns and vice versa. Once the array is transposed, you can directly assign it to the target range, effectively pasting the entire array in one step.
Can I directly assign the value of a range to an array in Excel VBA?
Yes, you can directly assign the value of a range to an array in Excel VBA. This method creates a 2D array, where the rows and columns correspond to the range dimensions. By resizing the target range to match the array size, you can then assign the array to the range in one go.
How can I fill an array with values and then paste it into a worksheet?
In Excel VBA, you can fill an array with values and then paste it into a worksheet using different techniques. One way is to manually populate the array with values and use the Transpose function to paste it into the range. Alternatively, you can take values directly from a worksheet range and fill the array, providing flexibility in data manipulation and array construction.
What are some additional array operations and examples in Excel VBA?
In addition to efficiently pasting arrays into ranges, Excel VBA offers various array operations and examples. You can learn how to pass and receive arrays, compare two arrays, and dynamically fill arrays. Understanding these array operations can enhance your data manipulation capabilities and help you efficiently paste arrays into ranges in Excel.
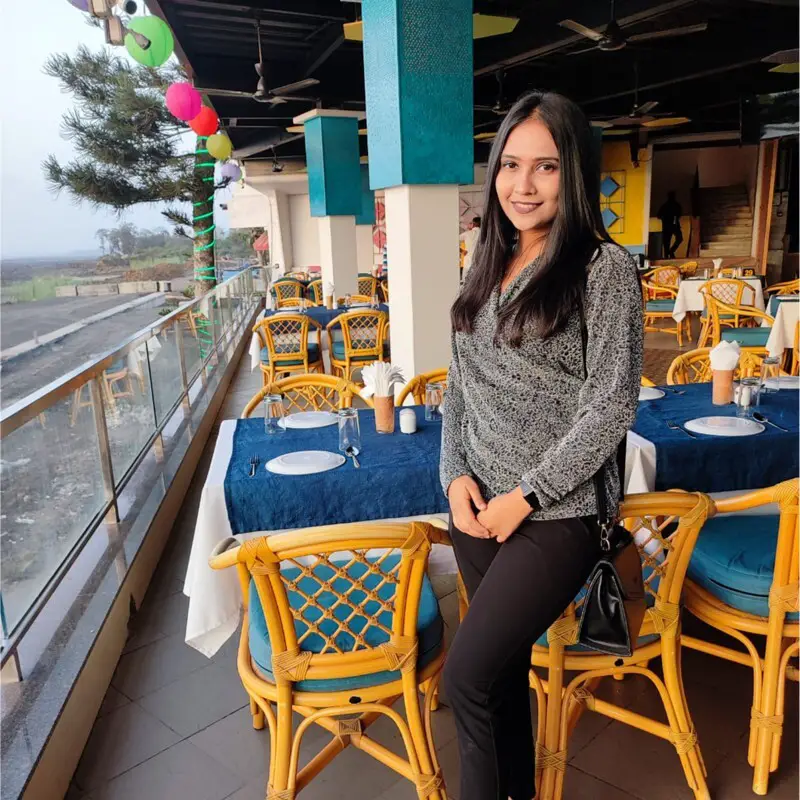
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.