Read Text Files Without Opening Them in Excel VBA
Did you know that you can read the contents of a text file in Excel VBA without actually opening the file? It’s true! This powerful feature allows you to extract information quickly and efficiently, without the need to manually open each file. Whether you’re dealing with large data files or simply want to streamline your workflow, being able to read text files without opening them in Excel VBA can be a game-changer.
In this article, we’ll explore a method that leverages the cmdLine object and the FindStr command to accomplish this task. We’ll delve into the details of how this method works and provide step-by-step instructions for implementing the code. Additionally, we’ll discuss considerations for handling multiple text files, performance optimization techniques, as well as testing and troubleshooting tips. By the end of this article, you’ll have a comprehensive understanding of how to read text files without opening them in Excel VBA.
Using cmdLine and FindStr for Efficient Text File Reading
When it comes to reading text files without opening them in Excel VBA, the cmdLine object and the FindStr command provide a powerful solution. By utilizing these tools, you can extract the first line of a text file without the need to open it. This approach offers significant efficiency gains, especially when dealing with large files. Here’s how it works:
- Create a CMD process using the cmdLine object in Excel VBA.
- Use the FindStr command within the CMD process to search for a specific character or string in the text file.
- Extract the line that contains the desired data, eliminating the need to open the file in Excel or any other program.
By leveraging the cmdLine object and the FindStr command, you can significantly reduce the processing time required to read text files in Excel VBA. This approach is particularly useful when dealing with large data sets where efficiency is crucial.
Example:
Here’s an example of how to use the cmdLine object and the FindStr command in Excel VBA to read the first line of a text file without opening it:
Sub ReadTextFileWithoutOpening() Dim cmdLine As Object Dim filePath As String Dim command As String Dim output As String ' Set the file path and command filePath = "C:\path\to\your\text\file.txt" command = "cmd /c FindStr /R /N ^""^"" " & filePath ' Create a CMD process Set cmdLine = CreateObject("WScript.Shell") ' Execute the command and capture the output output = cmdLine.Exec(command).StdOut.ReadAll ' Extract the first line Dim firstLine As String firstLine = Split(output, vbCrLf)(0) ' Print the first line Debug.Print firstLine End Sub
Advantages of Using cmdLine and FindStr:
Using the cmdLine object and the FindStr command in Excel VBA offers several advantages:
- Efficiency: The cmdLine and FindStr approach allows for faster processing of text files compared to traditional methods that involve opening each file individually.
- No file opening: With this approach, you can read the desired data from a text file without actually opening it in Excel or any other program. This can be beneficial when working with sensitive or complex files.
- Flexibility: The FindStr command provides extensive search capabilities, allowing you to find specific characters, strings, or patterns within a text file.
Implementing the Code for Reading Text Files
To read text files without opening them in Excel VBA, you can implement the following code:
- Declare a variable to hold the file path and name of the text file.
- Initialize the cmdLine object and set it to the cmd.exe process.
- Construct the FindStr command to search for the desired line in the text file.
- Execute the FindStr command using the cmdLine object and retrieve the output.
- Extract the desired line from the output.
- Process or display the extracted line as needed.
Here’s an example implementation of the code:
Dim filePath As String
Dim cmdLine As Object
Dim output As String
filePath = "C:\Path\To\Text\File.txt"
Set cmdLine = CreateObject("WScript.Shell")
output = cmdLine.Exec("cmd /c findstr /c:""YourSearchKeyword"" """ & filePath & """").StdOut.ReadAll
'Extract the desired line from the output
'Process or display the extracted line as needed
Ensure that you replace “YourSearchKeyword” with the specific character or string you want to search for in the text file.
Handling Multiple Text Files
If you need to read multiple text files without opening them in Excel VBA, you can adapt the code to handle this scenario. By using a loop, you can iterate through a folder containing the files, filter them by their extension, and then apply the same code logic for each file. This allows for efficient processing of multiple files in one execution.
To accomplish this, you can utilize the FileSystemObject
and Folder
objects in Excel VBA. Here is an example of how to handle multiple text files:
1. Get the Folder object
Use the Application.FileDialog(msoFileDialogFolderPicker)
method to prompt the user to select the folder containing the text files. This will return the path of the selected folder. Then, create a FileSystemObject
and Folder
object using the folder path.
2. Filter and process the text files
With the Folder
object, you can access all the files within the selected folder. Iterate through the files using a loop and filter them by their extension. In this case, we are looking for text files, so you can check if the file extension matches “.txt”.
3. Assign a unique file number
Using the FreeFile
function, assign a unique file number to each text file. This ensures that each file can be opened and read individually without any conflicts.
4. Read the content of each text file
Apply the code logic from earlier sections to read the content of each text file. Use the unique file number obtained in the previous step to open the file and extract the desired information. Depending on your specific requirements, you can extract the first line, specific lines, or the entire contents of the file.
Here is an example of how the code would look:
Sub HandlingMultipleTextFiles()
Dim fs As Object
Dim folder As Object
Dim file As Object
Dim fileNumber As Integer
' Prompt user to select the folder containing text files
Set fs = CreateObject("Scripting.FileSystemObject")
Set folder = fs.GetFolder("C:\Path\To\Folder")
' Iterate through each file in the folder
For Each file In folder.Files
' Check if the file is a text file (.txt)
If LCase(Right(file.Name, 4)) = ".txt" Then
' Assign a unique file number to each text file
fileNumber = FreeFile
' Open the file for reading
Open file.Path For Input As fileNumber
' Read the content of the file
LineInput #fileNumber, firstLine
' Process the first line of the file
'... your code here
' Close the file
Close fileNumber
End If
Next file
End Sub
You can further enhance this code by adding error handling, logging, or additional processing based on your specific needs.
By implementing this approach, you can handle multiple text files in Excel VBA without the need to open each file individually, improving efficiency and streamlining your workflow.
Considerations for Performance Optimization
When it comes to reading large text files without opening them in Excel VBA, performance optimization is crucial. By implementing certain strategies, you can significantly improve the efficiency and speed of your code. Here are some considerations to keep in mind:
1. Use the FindStr Command with Regex Patterns
In order to locate the desired data more efficiently, consider using the FindStr command with appropriate regex patterns. This command allows you to search for specific characters or strings within a file and retrieve the matching line. By using regex patterns, you can refine your search criteria and speed up the process.
2. Utilize the FreeFile Function
By utilizing the FreeFile function in Excel VBA, you can ensure that each file is assigned a unique file number. This allows you to open and read multiple files simultaneously, saving time and resources. Furthermore, closing each file once you have finished reading it is essential to reduce memory usage and enhance overall performance.
3. Optimize Memory Usage
Large text files can consume a significant amount of memory when reading them in Excel VBA. To optimize memory usage, consider using the following techniques:
- Keep unnecessary variables and objects to a minimum.
- Release memory by setting objects to
Nothing
once they are no longer needed. - Use the
Erase
statement to clear arrays.
4. Test Performance with Different File Sizes
In order to ensure optimal performance, it is essential to test your code with files of varying sizes. This will help you gauge the efficiency of your implementation and identify potential bottlenecks. Test your code with small, medium, and large text files to gain a comprehensive understanding of its performance characteristics.
5. Implement Error Handling
While optimizing performance is important, it is equally crucial to handle potential errors gracefully. Implement error handling mechanisms in your code to anticipate and adequately respond to issues such as file not found or permission errors. This will help maintain the integrity and reliability of your code.
By considering these performance optimization techniques, you can significantly enhance the efficiency and speed of your Excel VBA code when reading large text files without opening them. Fine-tuning your implementation based on these considerations will ensure a smooth and effective workflow.
Testing and Troubleshooting
Once you have implemented the code for reading text files without opening them in Excel VBA, it is crucial to thoroughly test and troubleshoot the functionality. Testing the code with different file sizes, formats, and configurations will ensure that it performs as expected in various scenarios. This step is essential to identify any potential issues and make necessary adjustments.
During testing, consider using text files of varying sizes, from small to large, to verify the code’s efficiency. By evaluating different file formats, such as .txt, .csv, and .log, you can ensure the code can handle various file types seamlessly. Additionally, test the code with different file configurations, including files located in different directories or network paths.
An important aspect of testing is to check how the code handles potential errors. Validate if the code gracefully handles scenarios such as files not found or permission issues. Implement robust error handling techniques in your code to capture and handle these exceptions appropriately.
Once you have tested the code thoroughly and identified any issues, it is time to troubleshoot. Go through the code line by line to identify any logical or syntax errors. Use debugging tools and techniques available in the Excel VBA environment to pinpoint and resolve any bugs that may affect the code’s functionality.
FAQ
Can I read a text file in Excel VBA without actually opening it?
Yes, you can read a text file in Excel VBA without opening it by using specific code. This method allows for faster processing of large files and avoids the need to open each file individually.
What is the most efficient method for reading text files without opening them in Excel VBA?
The most efficient method for reading text files without opening them in Excel VBA involves using the cmdLine object and the FindStr command. This method significantly reduces the processing time, especially when dealing with large files.
How do I implement the code for reading text files without opening them in Excel VBA?
To implement the code for reading text files without opening them in Excel VBA, you can follow a few steps. This involves using the cmdLine object and the FindStr command to extract the desired line from the text file.
Can I handle multiple text files without opening them in Excel VBA?
Yes, you can handle multiple text files without opening them in Excel VBA. By adapting the code and using a loop, you can iterate through a folder containing the files, filter them by their extension, and apply the same code logic for each file.
What considerations should I keep in mind for performance optimization when reading large text files without opening them in Excel VBA?
When reading large text files without opening them in Excel VBA, it’s important to consider performance optimization techniques. Strategies such as using appropriate regex patterns with the FindStr command and closing each file once it has been read can help improve performance.
How should I test and troubleshoot the code for reading text files without opening them in Excel VBA?
After implementing the code for reading text files without opening them in Excel VBA, it’s essential to thoroughly test and troubleshoot the functionality. Test the code with different file sizes, formats, and configurations to ensure it performs as expected. Additionally, implement error handling techniques to gracefully handle potential errors.
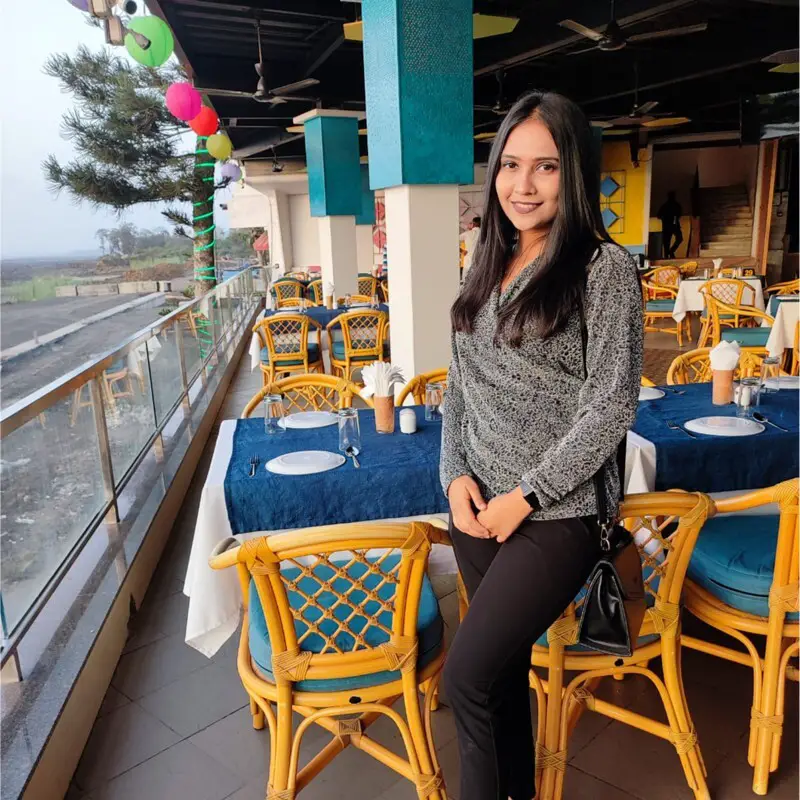
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.