How to Run JavaScript on a Web Page Using Excel VBA?
Excel VBA is a powerful automation tool that allows users to control external applications like web browsers. One common use case is controlling Internet Explorer (IE) to interact with web pages. If you want to run JavaScript on a web page using Excel VBA, the process involves using InternetExplorer.Application along with the execScript method.
In this guide, we will explain the step-by-step process, common challenges, and best practices for successfully executing JavaScript using VBA. Whether you are automating form submissions, triggering page events, or retrieving data from dynamic content, this tutorial will make the process simple and understandable.
Why Use VBA to Run JavaScript on a Web Page?
Running JavaScript using VBA is useful when you need to:
- Automate interactions with dynamic web pages.
- Trigger client-side events.
- Extract data loaded by JavaScript functions.
- Submit web forms requiring JavaScript validation.
Since JavaScript controls much of the interactive content on web pages, knowing how to trigger it directly via VBA can save time and enable more advanced automation.
Prerequisites – Setting Up VBA to Control Internet Explorer
Step 1 – Add Reference to Microsoft Internet Controls
Before using VBA to control Internet Explorer, you need to add a reference in the VBA Editor.
- Open Excel and press ALT + F11 to open the VBA Editor.
- Go to Tools > References.
- Scroll down and check Microsoft Internet Controls.
- Click OK.
This reference allows VBA to interact with Internet Explorer through COM objects.
Step 2 – Create Internet Explorer Object in VBA
The next step is to create an InternetExplorer.Application object, which gives VBA control over the browser.
Dim ie As Object
Set ie = CreateObject("InternetExplorer.Application")
Step 3 – Navigate to the Target Web Page
Use the Navigate method to load the desired web page.
ie.Navigate "http://example.com"
Step 4 – Wait Until Page Loads Completely
Before executing JavaScript, ensure the page has fully loaded. Use a Do While Loop to check the Busy property.
Do While ie.Busy
DoEvents
Loop
Step 5 – Run JavaScript Using execScript Method
Once the page loads, you can trigger JavaScript using the execScript method.
ie.Document.parentWindow.execScript "javascriptFunction()", "javascript"
Full Example VBA Code
Sub RunJavaScriptOnWebPage()
Dim ie As Object
Set ie = CreateObject("InternetExplorer.Application")
ie.Visible = True
ie.Navigate "http://example.com"
Do While ie.Busy
DoEvents
Loop
ie.Document.parentWindow.execScript "javascriptFunction()", "javascript"
Set ie = Nothing
End Sub
Handling Frames – Running JavaScript in Specific Frames
Many web pages use frames or iframes, especially older websites. To execute JavaScript inside a specific frame, you need to target the frame’s document.
Example VBA Code for Handling Frames
Sub RunJavaScriptOnWebPageWithFrames()
Dim ie As Object
Dim frame As Object
Set ie = CreateObject("InternetExplorer.Application")
ie.Visible = True
ie.Navigate "http://example.com"
Do While ie.Busy
DoEvents
Loop
Set frame = ie.Document.Frames(0)
frame.contentWindow.execScript "javascriptFunction()", "javascript"
Set frame = Nothing
Set ie = Nothing
End Sub
Explanation of Key Elements
Term | Description |
---|---|
Frames(0) | Targets the first frame (index starts at 0). |
contentWindow | Accesses the frame’s window object. |
execScript | Runs the JavaScript function inside the frame. |
Best Practices for Running JavaScript via Excel VBA
1. Always Wait for Page to Load
JavaScript functions may not exist until the page fully loads. Use ie.ReadyState
and ie.Busy
to ensure the page is ready.
Do While ie.Busy Or ie.ReadyState <> 4
DoEvents
Loop
2. Use Correct Frame Reference
If a page has multiple frames, Frames(0) may not contain the needed script. Use:
Set frame = ie.Document.Frames("frameName")
3. Check for JavaScript Errors
If the page’s JavaScript function fails, VBA may not show a clear error. Adding error handling helps detect such issues.
On Error Resume Next
frame.contentWindow.execScript "javascriptFunction()"
If Err.Number <> 0 Then
MsgBox "JavaScript execution failed."
End If
Common Issues and Troubleshooting
1. Page Still Loading
If the execScript method fails, check if the page is fully loaded. Add an additional check for ReadyState.
Do While ie.ReadyState <> 4
DoEvents
Loop
2. Function Not Defined
If the target JavaScript function does not exist or is misspelled, execScript
will silently fail. Double-check:
- Function name.
- Correct case (JavaScript is case-sensitive).
- Availability within the correct frame.
3. Frames Not Detected
If ie.Document.Frames.Length
returns 0, the page might not use traditional frames. Instead, modern pages often use iframes loaded dynamically with JavaScript.
Alternative – Use HTML Element Events
If direct execScript calls fail, another option is triggering an onclick event of an element that calls the target script.
ie.Document.getElementById("buttonID").Click
This approach is sometimes more reliable.
Retrieving Data from JavaScript Execution
VBA cannot directly capture return values from execScript
. To work around this, modify a page element’s content using the JavaScript function, and read that element back in VBA.
Example Workflow
Step | Action |
---|---|
1 | JavaScript updates a hidden input’s value. |
2 | VBA reads the value from the input. |
ie.Document.getElementById("resultField").innerText = myFunction()
Dim result As String
result = ie.Document.getElementById("resultField").innerText
Important Note – Internet Explorer Deprecation
Microsoft Internet Explorer is officially deprecated. This approach works with legacy systems but is not recommended for new automation tasks. Consider switching to Selenium with VBA, which supports modern browsers like Chrome and Edge.
Final Thoughts
Running JavaScript on a web page using Excel VBA can be highly effective for automating web processes. By following the structured steps outlined above, you can ensure reliable automation while handling both standard web pages and framed content. As Internet Explorer phases out, explore options like Selenium for more modern browser automation with VBA.
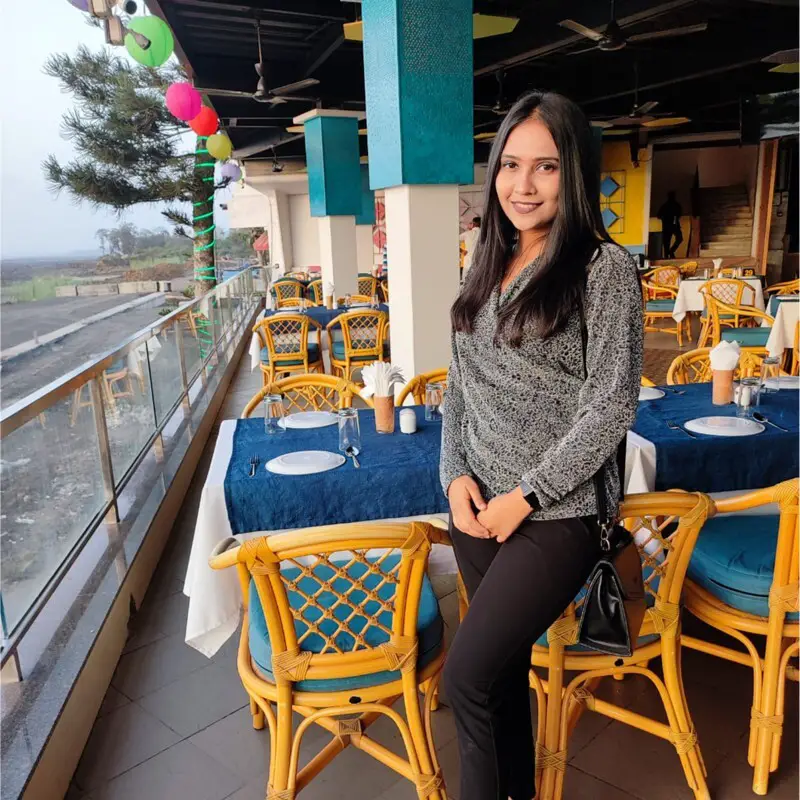
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.
There are already multiple ways to do this without the use of JavaScript. I am not sure why this is necessary.
examples
‘ Create an XMLHTTP object
Set oXMLHTTP = CreateObject(“MSXML2.ServerXMLHTTP”)
‘ Open the URL
oXMLHTTP.Open “GET”, sURL, False
‘ Send the request
oXMLHTTP.Send
‘ Get the response (source code)
and
‘ Get elements by class name
Set pElements = pHTML.getElementsByClassName(domClassName)
‘ Iterate through the elements
For Each pElement In pElements
If pElement.getAttribute(domAttribute) = domAttributeValue Then
‘ Process the element (e.g., extract data)
‘ …
End If
Next pElement
sPageHTML = oXMLHTTP.responseText
or
With CreateObject(“WINHTTP.WinHTTPRequest.5.1”)
.Open “GET”, url, False
.Send
pHTML.body.innerHTML = .ResponseText
End With
Well, more is always better. Using VBA to run Javascript on webpage has many benefits including automating web-based tasks, scraping web data into Excel for analysis, and interacting with web applications directly from Excel.
It’s good if you can do it without Javascript, but some people like me do need this.
Thanks for commenting!