Excel VBA R1C1 Formula with Variable Guide
Did you know that the R1C1 formula in Excel VBA provides a powerful way to reference cells using row and column numbers? Unlike the traditional A1-style notation, R1C1 notation allows for more flexibility in creating dynamic formulas. By incorporating variables, you can further enhance the functionality and adaptability of your VBA code.
In this guide, we will delve into how to use the R1C1 formula with variables in Excel VBA. Whether you want to sum up values, perform multiplication, or divide numbers, we’ve got you covered. By the end of this article, you’ll have a thorough understanding of how to leverage the R1C1 formula with variables to create dynamic and efficient VBA code.
Key Takeaways:
- The R1C1 formula notation in Excel VBA uses row and column numbers instead of the traditional A1-style referencing system.
- By incorporating variables into R1C1 formulas, you can create dynamic formulas that adjust based on the location of the cells.
- There are three suitable ways to use the R1C1 formula with variables in Excel VBA: summing up values, performing multiplication, and dividing numbers.
- Applying the FormulaR1C1 property allows you to set R1C1 formulas for specific ranges of cells.
- By referencing the row and column numbers in the formula, you can dynamically perform calculations based on the cell locations.
Introduction to the R1C1 Formula in Excel VBA
The R1C1 formula in Excel VBA is a powerful notation that allows you to reference cells using row and column numbers. Unlike the familiar A1-style referencing system, the R1C1 notation provides the flexibility of using relative and absolute references, making it an essential tool for creating dynamic formulas.
Understanding R1C1 Formula Notation
In the R1C1 notation, the ‘R’ represents the row number and the ‘C’ represents the column number. For example, R1C1 refers to the cell at the first row and first column, while R1C2 refers to the cell at the first row and second column.
The R1C1 formula notation uses square brackets to enclose the row and column numbers. To create a relative reference, you can use square brackets with a combination of absolute and relative values. Absolute references are denoted by adding a dollar sign ($) before the row or column number.
Benefits of Using R1C1 Formula Notation
The R1C1 formula notation offers several advantages over the A1-style notation. Firstly, it allows for easier replication and adjustment of formulas across multiple cells, especially when working with ranges or iterative calculations. Secondly, it provides a consistent way to reference cells, making formulas more understandable and maintainable. Lastly, the R1C1 notation is particularly useful when generating formulas dynamically in VBA code, as it simplifies the process of constructing the formula string.
Let’s take a look at an example:
Dim rng As Range
Set rng = Range("A1:A10")
rng.FormulaR1C1 = "=SUM(RC[-1]:RC[-2])"
In the above code snippet, we set the FormulaR1C1 property for the range A1:A10 to calculate the sum of the two preceding columns for each row. This demonstrates how the R1C1 formula notation can be used to create dynamic formulas efficiently.
Visual Representation of R1C1 Formula Notation
To further illustrate the R1C1 formula notation, consider the following table:
Option | Explanation |
---|---|
RC[-2] | Refers to the cell in the same row and two columns to the left |
R[-1]C | Refers to the cell in the row above and the same column |
R[2]C[-1] | Refers to the cell two rows below and one column to the left |
R[1]C[1] | Refers to the cell in the row below and one column to the right |
By understanding how to interpret the R1C1 formula notation, you can leverage its flexibility and power to create more dynamic and efficient formulas in Excel VBA.
How to Use R1C1 Formula with Variable in Excel VBA: 3 Suitable Ways
When working with the R1C1 formula in Excel VBA, using variables can greatly enhance the flexibility and dynamic nature of your code. In this section, we will explore three suitable ways to utilize the R1C1 formula with variables in Excel VBA. These methods will allow you to perform calculations and manipulate data in a more efficient and customizable manner.
1. Applying the R1C1 Formula with Variables to Sum Up Values
One of the first ways you can leverage the power of the R1C1 formula with variables in Excel VBA is by using it to perform summations. By setting the formulaR1C1 property and referencing the appropriate row and column numbers, you can dynamically sum up values based on the location of the cells. Below is an example of how this can be achieved:
' Define variables and set their values
Dim sumRange As Range
Dim resultCell As Range
Set sumRange = Range("A1:A5")
Set resultCell = Range("C1")
' Assign the R1C1 formula with variables to the result cell
resultCell.FormulaR1C1 = "=SUM(R[-4]C[-2]:R[-1]C[-2])"
2. Performing Multiplication Using the R1C1 Formula with Variables
In addition to summing up values, you can also utilize the R1C1 formula with variables to perform multiplication operations. By referencing the appropriate row and column numbers within the formula, you can create dynamic calculations that adjust based on the location of the cells. Take a look at the following example:
' Define variables and set their values
Dim MultiplyRange As Range
Dim resultCell As Range
Set MultiplyRange = Range("A1:A5")
Set resultCell = Range("C1")
' Assign the R1C1 formula with variables to the result cell
resultCell.FormulaR1C1 = "=PRODUCT(R[-4]C[-2]:R[-1]C[-2])"
3. Using the R1C1 Formula with Variables to Divide Values
The R1C1 formula with variables can also be employed to divide values in Excel VBA. By referencing the appropriate row and column numbers within the formula, you can create dynamic calculations that adjust based on the location of the cells. Here’s an example of how this can be done:
' Define variables and set their values
Dim DivideRange As Range
Dim resultCell As Range
Set DivideRange = Range("A1:A5")
Set resultCell = Range("C1")
' Assign the R1C1 formula with variables to the result cell
resultCell.FormulaR1C1 = "=R[-4]C[-2]/R[-1]C[-2]"
By understanding and utilizing these three suitable methods, you can harness the power of the R1C1 formula with variables in Excel VBA to perform a wide range of calculations and data manipulations. Experiment with these techniques to enhance your VBA code and achieve greater efficiency in your Excel workflows.
Apply R1C1 Formula with Variable to Sum Up in Excel VBA
To sum up values using the R1C1 formula with variables in Excel VBA, you can make use of the FormulaR1C1
property. This property allows you to set a formula in R1C1 notation for a specific range of cells. By referencing the row and column numbers in the formula, you can dynamically sum up values based on the location of the cells.
Here’s an example of how you can apply the R1C1 formula with variables to sum up values in Excel VBA:
Sub SumUpValues()
Dim SumRange As Range
Dim SumFormula As String
' Define the range of cells to sum up
Set SumRange = Range("A1:A5")
' Create the R1C1 formula
SumFormula = "=SUM(R[-1]C:R[3]C)"
' Set the formula for the sum range
SumRange.FormulaR1C1 = SumFormula
End Sub
In this example, we first define the range of cells to sum up using the Range
function. Next, we create the R1C1 formula by assigning it to a variable. The formula uses relative references to specify the range to sum up (R[-1]C:R[3]C
, which represents the range from 1 row above to 3 rows below the current cell). Finally, we set the formula for the sum range using the FormulaR1C1
property.
By using variables in the R1C1 formula, you can easily adjust the range based on your specific requirements. This makes it easier to create dynamic formulas that adapt to changes in your data.
With the provided VBA code and the flexibility of the R1C1 formula, you can easily sum up values based on your specific needs. Whether it’s calculating totals, subtotals, or any other type of summation, the R1C1 formula with variables in Excel VBA provides a powerful solution.
Summary: Apply R1C1 Formula with Variable to Sum Up in Excel VBA
By using the FormulaR1C1
property and variables, you can dynamically sum up values in Excel VBA. The R1C1 formula notation allows you to reference cells using row and column numbers, providing flexibility and adaptability. By adjusting the range based on your needs, you can easily create dynamic formulas that automatically recalculate as your data changes.
Benefits | Implementation Steps |
---|---|
Flexibility in referencing cells | 1. Define the range of cells to sum up 2. Create the R1C1 formula with variables 3. Set the formula for the sum range |
Dynamic formulas that adjust to changes | |
Ease of use and adaptability |
Perform Multiplication Using R1C1 Formula with Variable in Excel VBA
If you’re looking to perform multiplication operations using the R1C1 formula with variables in Excel VBA, you’re in the right place. By utilizing the power of the R1C1 notation, you can create dynamic formulas that adjust to the location of different cells. This flexibility allows for more efficient and automated calculations in your VBA code.
To multiply values using the R1C1 formula with variables, you’ll need to set the appropriate formula in R1C1 notation. This involves referencing the row and column numbers of the cells you want to multiply, which ensures accurate calculations regardless of the cell’s position.
Let’s take a look at an example VBA code snippet:
'Set variables
Dim firstCell As Range
Dim secondCell As Range
Dim resultCell As Range
'Initialize variables
Set firstCell = Range("A1")
Set secondCell = Range("B1")
Set resultCell = Range("C1")
'Set the formula in R1C1 notation
resultCell.FormulaR1C1 = "=RC[-2] * RC[-1]"
In the above code, we define three range variables: firstCell
, secondCell
, and resultCell
. We then set the values of these variables to the desired cell references (e.g., Range("A1")
). Finally, we use the FormulaR1C1
property of the resultCell
to assign the multiplication formula in R1C1 notation.
By utilizing variables in the formula, you can easily change the cell references to suit your specific needs. This flexibility enables you to perform multiplication operations dynamically, adapting to different scenarios without the need for manual adjustments.
Here’s an example table showcasing the multiplication of values using the R1C1 formula with variables:
Value 1 | Value 2 | Result |
---|---|---|
5 | 3 | 15 |
10 | 2 | 20 |
8 | 4 | 32 |
By implementing the R1C1 formula with variables in your Excel VBA code, you can efficiently perform any required multiplication calculations. The dynamic nature of R1C1 notation allows for easy adjustments and scalability, making your code more robust and adaptable.
Use R1C1 Formula with Variable to Divide in Excel VBA
To divide values using the R1C1 formula with variables in Excel VBA, you can utilize the FormulaR1C1 property. This property allows you to set the appropriate formula in R1C1 notation, incorporating variables to create dynamic formulas. By referencing the row and column numbers of the desired cells, you can ensure that the formula adjusts seamlessly based on the location of the cells.
Implementing the R1C1 formula with variables to perform division in Excel VBA is straightforward. By using the appropriate syntax and manipulating the FormulaR1C1 property, you can create efficient and accurate division formulas. Whether you need to divide specific cells or incorporate more complex calculations, the R1C1 notation with variables offers versatility and precision in your Excel VBA code.
Here’s an example of how you can use the R1C1 formula with variables to divide values in Excel VBA:
Sub DivideValues()
Dim dividend As Variant
Dim divisor As Variant
Dim result As Variant
' Set the dividend and divisor values
dividend = Range("A1").Value
divisor = Range("B1").Value
' Perform the division using the R1C1 formula with variables
result = Application.Evaluate("=R[-1]C/R[,-1]C")
' Output the result to a specific cell
Range("C1").Value = result
End Sub
In the above example, we’re dividing the values in cells A1 and B1 and storing the result in cell C1. By utilizing the R1C1 formula with variables within the Application.Evaluate method, we ensure that the result adjusts based on the location of the cells.
By incorporating the R1C1 formula with variables, you can enhance the flexibility and efficiency of your division calculations in Excel VBA. Experimenting with different variables and ranges will help you unlock the full potential of this powerful feature.
FAQ
What is the R1C1 formula in Excel VBA?
The R1C1 formula in Excel VBA is a notation that uses row and column numbers to reference cells. It provides the ability to use relative and absolute references, making it a powerful tool for creating dynamic formulas.
How can I use the R1C1 formula with variables in Excel VBA?
There are three suitable ways to use the R1C1 formula with variables in Excel VBA. You can use it to sum up values, perform multiplication, and divide values. Each method offers flexibility in referencing cells based on their location.
How do I apply the R1C1 formula with variables to sum up values in Excel VBA?
To sum up values using the R1C1 formula with variables in Excel VBA, you can use the FormulaR1C1 property. This property allows you to set a formula in R1C1 notation for a specific range of cells. By referencing the row and column numbers in the formula, you can dynamically sum up values based on the location of the cells.
How can I perform multiplication using the R1C1 formula with variables in Excel VBA?
If you need to multiply values using the R1C1 formula with variables in Excel VBA, you can do so by setting the appropriate formula in R1C1 notation. By referencing the row and column numbers of the cells you want to multiply, you can create a dynamic formula that adjusts based on the location of the cells.
How do I use the R1C1 formula with variables to divide values in Excel VBA?
To divide values using the R1C1 formula with variables in Excel VBA, you can use the FormulaR1C1 property and set the appropriate formula in R1C1 notation. By referencing the row and column numbers of the cells you want to divide, you can create a dynamic formula that adjusts based on the location of the cells.
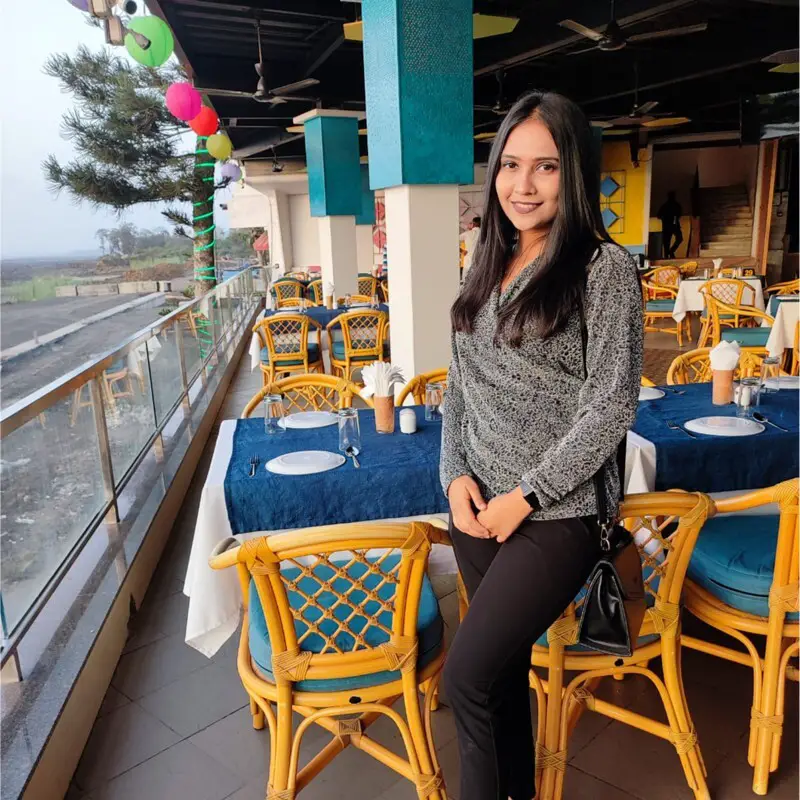
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.