Solving Excel VBA ByRef Argument Type Mismatch
Did you know that the ByRef Argument Type Mismatch error is one of the most common issues encountered when working with Excel VBA macros?
When you’re troubleshooting macros and encounter this error, it can be frustrating and time-consuming to pinpoint the cause and find a solution. But fear not! In this article, we will delve into the world of Excel VBA and explore how to resolve this error effectively.
Key Takeaways
- ByRef Argument Type Mismatch error is a common issue in Excel VBA macros.
- Understanding the difference between ByRef and ByVal is crucial in resolving this error.
- Common causes of this error include incorrect variable declarations and mismatched data types.
- Resolving the error involves checking variable declarations, argument types, and parameter types.
- Following best practices can help you avoid encountering this error in the first place.
Understanding ByRef and ByVal in VBA.
When working with VBA, it is essential to have a clear understanding of how arguments are passed to functions and subroutines. VBA provides two methods for passing arguments: ByRef and ByVal.
ByRef:
ByRef stands for “by reference.” When an argument is passed ByRef, any changes made to the argument within the function or subroutine will affect the original variable. In other words, the argument and the original variable refer to the same memory location.
ByVal:
On the other hand, ByVal stands for “by value.” When an argument is passed ByVal, a copy of the argument’s value is passed to the function or subroutine. Any changes made to the argument within the function or subroutine will not affect the original variable.
Understanding the difference between ByRef and ByVal is crucial in resolving the ByRef Argument Type Mismatch error. This error occurs when there is a mismatch between the variable types of the arguments being passed. By knowing when to use ByRef and when to use ByVal, you can avoid this error and ensure that your VBA code functions correctly.
ByRef Example:
Let’s consider an example:
Sub IncrementByOne(ByRef num As Integer)
num = num + 1
End Sub
Sub Main()
Dim x As Integer
x = 5
IncrementByOne x
MsgBox x ' Output: 6
End Sub
In the above example, the function “IncrementByOne” takes an argument “num” passed ByRef. When the value of “num” is incremented within the function, the original variable “x” also gets incremented.
ByVal Example:
Now, let’s consider another example:
Sub Square(ByVal num As Double)
num = num * num
MsgBox num ' Output: 25
End Sub
Sub Main()
Dim x As Double
x = 5
Square x
MsgBox x ' Output: 5
End Sub
In this example, the function “Square” takes an argument “num” passed ByVal. When the value of “num” is squared within the function, the original variable “x” remains unchanged.
By understanding the behavior of ByRef and ByVal, you can effectively manage the arguments in your VBA code and avoid common errors like ByRef Argument Type Mismatch.
Common Causes of ByRef Argument Type Mismatch.
When working with VBA, the ByRef Argument Type Mismatch error can occur due to several common causes. It is important to understand these causes in order to effectively troubleshoot and resolve this error.
1. Declaring Multiple Variables on a Single Line
One frequent cause of the ByRef Argument Type Mismatch error is declaring multiple variables on a single line without specifying the data type for each variable separately. This can lead to one of the variables being declared as a Variant, resulting in a mismatch when passing arguments. It is crucial to ensure that each variable is declared with the appropriate data type.
2. Passing Arguments of Different Data Types
Another common cause of the ByRef Argument Type Mismatch error is passing arguments of different data types than what is expected by the function or subroutine. It is essential to carefully review the data types of the arguments and ensure that they match the data types specified in the parameter declaration of the function or subroutine.
By addressing these causes, you can significantly reduce the occurrence of the ByRef Argument Type Mismatch error in your VBA code.
Example:
Incorrect Code | Corrected Code |
---|---|
Sub Example(ByRef var1, var2 As Integer) | Sub Example(ByRef var1 As Variant, ByVal var2 As Integer) |
Sub Example(var1 As String, var2 As Integer) | Sub Example(ByRef var1 As String, ByVal var2 As Integer) |
By ensuring that each variable is declared with the correct data type and matching the argument data types with the parameter data types, you can avoid the ByRef Argument Type Mismatch error in your VBA code.
Resolving the ByRef Argument Type Mismatch Error.
To resolve the ByRef Argument Type Mismatch error in VBA, follow these steps:
- Ensure that all variables are declared with the correct data types.
- If you are declaring multiple variables on a single line, be sure to specify the data type for each variable separately.
- Double-check that the arguments being passed to the function or subroutine match the declared parameter types.
- If necessary, use casting or conversion functions to ensure compatibility between argument and parameter types.
- Consider using the ByVal keyword instead of ByRef if it aligns with your application’s requirements.
By following these steps, you can troubleshoot and resolve the ByRef Argument Type Mismatch error, ensuring that your VBA macros run smoothly.
Example of Resolving the ByRef Argument Type Mismatch.
Let’s take a look at an example to demonstrate how to resolve the ByRef Argument Type Mismatch error in VBA programming. Suppose we have a function called “ProcessString” that takes a string argument and returns a cleaned string. If we encounter the error when calling this function, we can follow these steps to resolve it:
Step 1: Check the Data Type
The first step is to check the data type of the argument being passed to the “ProcessString” function. Ensure that the argument is indeed a string, as expected.
Step 2: Review Parameter Declaration
Next, review the parameter declaration of the “ProcessString” function. Make sure that the parameter is properly declared as a string type. If it is declared differently, such as a variant or another data type, correct it to match the expected data type.
Step 3: Make Necessary Corrections and Adjustments
After identifying any discrepancies between the argument data type and the function’s parameter declaration, make the necessary corrections and adjustments. Update the argument or parameter declaration to ensure that they match in data type.
By following these steps, you should be able to resolve the ByRef Argument Type Mismatch error in your VBA code. This will allow the “ProcessString” function to be called successfully without any issues, ensuring smooth execution of your code.
See the image above for a visual representation of the steps involved in resolving the ByRef Argument Type Mismatch error.
Best Practices to Avoid ByRef Argument Type Mismatch.
Preventing the ByRef Argument Type Mismatch error is crucial to ensure smooth VBA coding. By following these best practices, you can minimize the chances of encountering this error and improve the overall efficiency of your macros.
- Declare variables with appropriate data types: Always specify the correct data type when declaring variables. This helps to avoid any confusion or mismatch when passing arguments to functions or subroutines.
- Avoid declaring multiple variables on a single line without specifying the data type for each: If you need to declare multiple variables, make sure to specify the data type for each variable separately. This ensures that each variable has its own explicit data type and eliminates any potential mismatch.
- Use explicit data type conversions when necessary: When passing arguments to functions or subroutines, it is essential to ensure compatibility between the arguments’ data types and the parameters’ expected data types. Use explicit data type conversions, such as CStr(), CDbl(), or CInt(), to convert arguments to the appropriate data types if needed.
- Consider using ByVal instead of ByRef: ByRef means passing arguments by reference, where any changes made to the arguments within the function or subroutine affect the original variables. In some cases, using ByVal, which passes arguments by value, might be more appropriate and help avoid the ByRef Argument Type Mismatch error.
- Test thoroughly: Before deploying your VBA code, thoroughly test it to identify any potential ByRef Argument Type Mismatch errors. Develop a comprehensive testing strategy and ensure that your code handles a variety of scenarios with different argument types and data structures.
By implementing these best practices, you can significantly reduce the occurrence of the ByRef Argument Type Mismatch error and enhance the reliability and efficiency of your Excel VBA macros.
Conclusion.
The ByRef Argument Type Mismatch error is a common issue that VBA developers often encounter. However, by gaining a clear understanding of the difference between ByRef and ByVal, identifying the common causes of this error, and following best practices, you can effectively troubleshoot and resolve it.
To prevent this error, always ensure that your variable declarations, argument types, and parameter types are accurately defined and compatible. Be diligent in specifying the data types for each variable when declaring multiple variables on a single line. Additionally, consider using explicit data type conversions when necessary to guarantee compatibility between arguments and parameters.
By implementing these strategies, you can minimize the occurrence of the ByRef Argument Type Mismatch error and ensure the smooth execution of your VBA macros. Remember to regularly double-check your code to ensure all variables and arguments are appropriately defined, giving you the confidence that your programs will run seamlessly.
FAQ
What is the ByRef Argument Type Mismatch error in Excel VBA?
The ByRef Argument Type Mismatch error occurs when there is a mismatch between the variable types of the arguments being passed to a function or subroutine in VBA.
What is the difference between ByRef and ByVal in VBA?
ByRef means that the argument is passed by reference, and any changes made to the argument within the function or subroutine will affect the original variable. ByVal means that the argument is passed by value, and any changes made to the argument will not affect the original variable.
What are the common causes of the ByRef Argument Type Mismatch error in VBA?
Common causes of the error include declaring multiple variables on a single line without specifying the data type for each variable separately, and passing arguments of different data types than what is expected by the function or subroutine.
How can I resolve the ByRef Argument Type Mismatch error in VBA?
To resolve the error, you can ensure that all variables are declared with the correct data types, double-check that the arguments being passed match the declared parameter types, and consider using ByVal instead of ByRef if appropriate.
Can you provide an example of how to resolve the ByRef Argument Type Mismatch error?
Sure! Let’s say we have a function called “ProcessString” that takes a string argument and returns a cleaned string. If we encounter the error when calling this function, we can check the data type of the argument being passed and review the function’s parameter declaration to ensure it matches the expected data type.
What are some best practices to avoid the ByRef Argument Type Mismatch error in VBA?
Best practices include declaring variables with the appropriate data types, avoiding declaring multiple variables on a single line without specifying the data type for each, using explicit data type conversions when necessary, and considering using ByVal instead of ByRef when passing arguments.
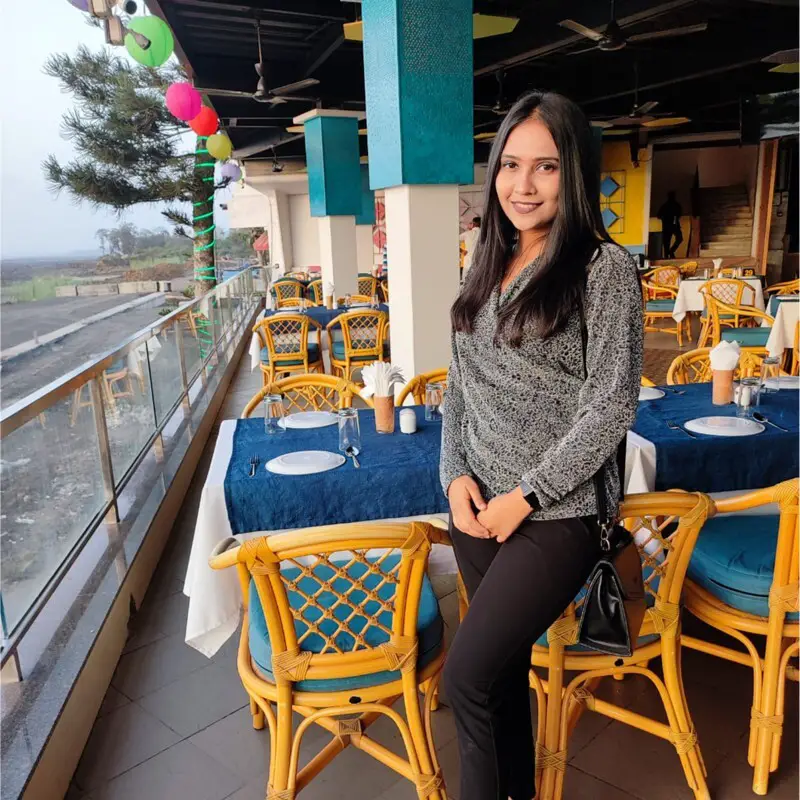
Vaishvi Desai is the founder of Excelsamurai and a passionate Excel enthusiast with years of experience in data analysis and spreadsheet management. With a mission to help others harness the power of Excel, Vaishvi shares her expertise through concise, easy-to-follow tutorials on shortcuts, formulas, Pivot Tables, and VBA.